React Native and Voice Recognition: Building Voice-Controlled Apps
In the ever-evolving landscape of mobile app development, user experience plays a pivotal role. One of the most innovative and interactive ways to enhance the user experience is through voice recognition. By incorporating voice control into your React Native applications, you can create a hands-free and seamless interaction for your users. In this blog, we will dive deep into the world of React Native and voice recognition, exploring how to build voice-controlled apps that provide a unique and intuitive user experience.
Table of Contents
1. Why Voice-Controlled Apps?
Voice-controlled apps have gained immense popularity in recent years due to their convenience and accessibility. Here are some compelling reasons to consider integrating voice recognition into your React Native apps:
1.1. Enhanced User Experience
Voice-controlled apps offer a more natural and intuitive way for users to interact with your application. Users can simply speak commands or queries, making the app feel more conversational and user-friendly.
1.2. Accessibility and Inclusivity
Voice recognition technology caters to a wider audience, including those with disabilities or individuals who may have difficulty using traditional touch interfaces. By making your app voice-enabled, you can ensure that everyone can use it.
1.3. Competitive Advantage
Incorporating voice control sets your app apart from competitors and can attract users looking for a modern and engaging experience. It’s an opportunity to stand out in a crowded marketplace.
Now that we understand the benefits of voice-controlled apps, let’s explore how to implement voice recognition in React Native.
2. Getting Started with React Native
Before we dive into voice recognition, let’s ensure you have a basic understanding of React Native. If you’re new to React Native, consider this section as your quick introduction.
2.1. Setting Up Your React Native Environment
To start building voice-controlled apps, you’ll need to set up your React Native development environment. Ensure you have Node.js, npm (Node Package Manager), and the React Native CLI installed. If not, you can follow the official React Native documentation for installation instructions.
Once your environment is set up, create a new React Native project using the following command:
shell npx react-native init VoiceControlledApp
Now, navigate to your project folder:
shell cd VoiceControlledApp
You’re now ready to integrate voice recognition into your app.
3. Integrating Voice Recognition
3.1. Choosing the Right Voice Recognition Library
React Native provides various libraries and plugins to integrate voice recognition into your app. One popular choice is the React Native Voice module, which offers a straightforward way to access device voice recognition capabilities.
To add the React Native Voice library to your project, run the following command:
shell npm install react-native-voice --save
3.2. Permissions and Configuration
Voice recognition requires user permissions to access the device’s microphone. You’ll need to configure these permissions in your React Native project. First, open your AndroidManifest.xml (for Android) and Info.plist (for iOS) files and add the necessary permissions.
For Android, add the following lines to your AndroidManifest.xml:
xml <uses-permission android:name="android.permission.RECORD_AUDIO" />
For iOS, open your Info.plist file and add the following key-value pair:
xml <key>NSMicrophoneUsageDescription</key> <string>We need access to your microphone for voice recognition.</string>
3.3. Using the React Native Voice Library
Now that your environment is set up, and permissions are configured, you can start using the React Native Voice library in your app. Here’s a basic example of how to capture voice input:
javascript import Voice from 'react-native-voice'; // Initialize the voice recognition module Voice.onSpeechStart = () => { console.log('Speech recognition started'); }; Voice.onSpeechEnd = () => { console.log('Speech recognition ended'); }; Voice.onSpeechResults = (e) => { console.log('Speech results:', e.value); }; // Start voice recognition Voice.start('en-US'); // Stop voice recognition Voice.stop();
In the code above, we import the Voice module and set up event listeners for speech start, speech end, and speech results. The Voice.start(‘en-US’) method initiates voice recognition in English, and Voice.stop() stops it.
3.4. Building a Voice-Controlled Feature
Let’s take things a step further and create a simple voice-controlled feature in our React Native app. Imagine a note-taking app where users can add new notes by voice command.
Creating the VoiceNote Component
javascript import React, { useState } from 'react'; import { View, Text, TouchableOpacity } from 'react-native'; import Voice from 'react-native-voice'; const VoiceNote = () => { const [isListening, setIsListening] = useState(false); const [note, setNote] = useState(''); const startListening = () => { setIsListening(true); Voice.start('en-US'); }; const stopListening = () => { setIsListening(false); Voice.stop(); }; Voice.onSpeechResults = (e) => { setNote(e.value[0]); // Capture the recognized speech stopListening(); }; return ( <View> {isListening ? ( <Text>Listening...</Text> ) : ( <> <Text>{note}</Text> <TouchableOpacity onPress={startListening}> <Text>Start Listening</Text> </TouchableOpacity> </> )} </View> ); }; export default VoiceNote;
In this component, we create a simple voice note-taking feature. When the user presses “Start Listening,” the app begins voice recognition, and the recognized speech is displayed as a note. You can integrate this component into your app as needed.
4. Voice-Controlled Apps Use Cases
Voice recognition can be applied to various use cases across different industries. Here are some examples of voice-controlled apps:
4.1. Virtual Assistants
Voice-controlled virtual assistants like Siri, Alexa, and Google Assistant have become an integral part of our lives. You can create your own virtual assistant using React Native and voice recognition, tailored to specific tasks or industries.
4.2. Accessibility Apps
Build apps that assist individuals with disabilities by providing voice-based controls for navigation, communication, and more.
4.3. Home Automation
Voice-controlled home automation apps allow users to control smart devices like lights, thermostats, and security systems with voice commands.
4.4. Language Learning
Develop language learning apps that help users improve their pronunciation and speaking skills by providing real-time feedback through voice recognition.
4.5. Gaming
Enhance gaming experiences by incorporating voice commands for character actions, navigation, and in-game communication.
5. Best Practices for Voice-Controlled Apps
Building voice-controlled apps requires attention to detail and best practices to ensure a smooth user experience:
5.1. Clear Voice Commands
Design voice commands that are natural and easy for users to understand. Provide a list of available commands within the app or through voice prompts.
5.2. Error Handling
Implement robust error handling to gracefully handle misinterpretations or failed voice recognition attempts. Provide feedback to users when their commands are not understood.
5.3. Privacy and Data Security
Respect user privacy by clearly communicating how voice data is collected and used. Encrypt voice data and ensure it is stored securely.
5.4. Continuous Improvement
Regularly update your voice recognition models to improve accuracy and adapt to evolving speech patterns and languages.
5.5. Testing
Thoroughly test voice-controlled features with diverse user groups to identify and address usability issues and improve accuracy.
Conclusion
Voice recognition technology opens up exciting opportunities for creating interactive and user-friendly apps with React Native. Whether you want to build a virtual assistant, an accessibility app, or a voice-controlled game, the possibilities are endless. By following best practices and leveraging the React Native Voice library, you can provide an engaging and accessible user experience that sets your app apart in the competitive mobile app landscape.
So, why wait? Start building your voice-controlled React Native app today and explore the future of mobile app interaction.
Table of Contents
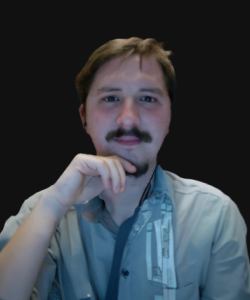
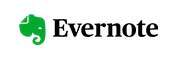