Building Voice Recognition Apps with React Native and AWS Lex
In the ever-evolving landscape of technology, voice recognition has emerged as a powerful tool, transforming the way we interact with devices and applications. With the rise of virtual assistants like Alexa and Siri, integrating voice recognition capabilities into mobile apps has become increasingly popular. In this guide, we’ll explore how to harness the power of React Native and AWS Lex to build cutting-edge voice recognition apps that deliver seamless user experiences.
Why Voice Recognition?
Voice recognition technology offers numerous benefits, including hands-free operation, accessibility for users with disabilities, and enhanced user engagement. By enabling users to interact with your app using natural language commands, you can create a more intuitive and efficient user experience.
Getting Started with React Native
React Native provides a robust framework for building cross-platform mobile applications using JavaScript and React. Its modular architecture and extensive library of pre-built components make it an ideal choice for developing voice recognition apps.
To get started, make sure you have Node.js and npm installed on your system. Then, install the React Native CLI and create a new project:
npm install -g react-native-cli react-native init VoiceRecognitionApp cd VoiceRecognitionApp
Next, install the necessary dependencies for integrating voice recognition:
npm install react-native-voice aws-sdk
Integrating AWS Lex
AWS Lex is a powerful service that enables you to build conversational interfaces for your applications. It provides automatic speech recognition (ASR) and natural language understanding (NLU) capabilities, making it easy to process and respond to user input.
First, create a new bot in the AWS Lex console and define the intents and utterances that your app will support. Then, configure the necessary permissions and deploy your bot.
In your React Native app, initialize the AWS SDK and configure it with your AWS credentials:
import AWS from 'aws-sdk'; AWS.config.update({ region: 'YOUR_AWS_REGION', credentials: new AWS.Credentials('YOUR_ACCESS_KEY_ID', 'YOUR_SECRET_ACCESS_KEY'), }); const lex = new AWS.LexRuntime();
Implementing Voice Recognition
Now that you have set up React Native and integrated AWS Lex, it’s time to implement voice recognition functionality in your app. Start by creating a new component for handling voice input:
import React, { useState } from 'react'; import { View, Button, Text } from 'react-native'; import Voice from 'react-native-voice'; const VoiceRecognition = () => { const [recognizedText, setRecognizedText] = useState(''); const startRecognition = async () => { try { Voice.start('en-US'); Voice.onSpeechResults = (e) => { setRecognizedText(e.value[0]); handleVoiceCommand(e.value[0]); }; } catch (error) { console.error(error); } }; const handleVoiceCommand = (text) => { const params = { botAlias: '$LATEST', botName: 'YOUR_BOT_NAME', inputText: text, userId: 'user-id', }; lex.postText(params, (err, data) => { if (err) console.log(err, err.stack); else console.log(data); }); }; return ( <View> <Button title="Start Listening" onPress={startRecognition} /> <Text>{recognizedText}</Text> </View> ); }; export default VoiceRecognition;
Conclusion
By combining the power of React Native and AWS Lex, you can create immersive voice recognition experiences that delight users and differentiate your app from the competition. Whether you’re building a virtual assistant, a language learning app, or a smart home control interface, voice recognition opens up a world of possibilities for innovation and user engagement.
Unlock the potential of voice technology today and revolutionize the way your users interact with your app!
External Resources
In this guide, we’ve only scratched the surface of what’s possible with voice recognition in React Native. Experiment with different features and integrations to create truly immersive and intuitive experiences for your users. Happy coding!
Table of Contents
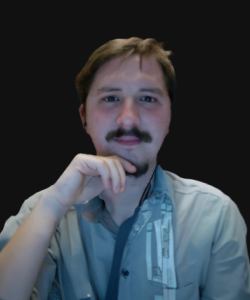
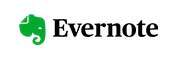