Exploring the Core of React Native: Unpacking Component Architecture
When it comes to mobile application development, the number of available frameworks can be overwhelming. Each one brings a unique set of features and benefits to the table. Among these, React Native stands out as a powerful JavaScript framework that facilitates the creation of real, natively-rendered mobile applications for both iOS and Android platforms. Given its strengths, there has been a marked increase in demand to hire React Native developers.
Derived from React – Facebook’s popular JavaScript library for building user interfaces – React Native targets mobile platforms rather than the browser. It adheres to the same design principles of React, highlighting declarative programming and a component-based architecture. In this blog post, we will delve into React Native’s component architecture, illustrating its ease of use and flexibility with practical examples.
Through this exploration, not only will you gain a deeper understanding of React Native’s core architectural principles, but you will also garner insights into the knowledge and skills required when you’re looking to hire React Native developers. It is essential to ensure that they possess the aptitude to leverage the power and flexibility of this robust framework fully.
1. Understanding Components
In the realm of React Native, components are the building blocks of your mobile application. They encapsulate parts of the user interface (UI), and these individual components can be put together to create complex UIs.
There are two types of components in React Native: functional and class-based components.
1.1 Functional Components
Functional components are JavaScript functions that accept props as an argument and return a React element. These components are typically simpler and easier to read and test. Here’s an example of a functional component:
```jsx import React from 'react'; import { Text } from 'react-native'; const HelloWorld = (props) => { return <Text>Hello, {props.name}!</Text>; }; export default HelloWorld; ```
In the example above, HelloWorld is a functional component that takes props as an argument and returns a Text component from React Native. It displays a greeting to the user based on the ‘name’ prop.
1.2 Class Components
Class components are more traditional and provide more features out of the box. They are ES6 classes that extend `React.Component` and must implement the render method. Here’s a similar HelloWorld component using class syntax:
```jsx import React, { Component } from 'react'; import { Text } from 'react-native'; class HelloWorld extends Component { render() { return <Text>Hello, {this.props.name}!</Text>; } } export default HelloWorld; ```
In this example, HelloWorld extends the Component class from React, implements the render method, and accesses props using `this.props`.
2. Composing Components
React Native thrives on the concept of “composition” – the idea of building complex UIs from smaller, reusable pieces. Let’s consider a simple app that displays a list of users and their details. We could create separate components for each piece of the UI: UserList, UserCard, UserName, and UserDetail.
2.1 UserList Component
This is a parent component that contains multiple UserCard components:
```jsx import React from 'react'; import { View } from 'react-native'; import UserCard from './UserCard'; const UserList = ({ users }) => { return ( <View> {users.map((user, index) => <UserCard key={index} user={user} /> )} </View> ); }; export default UserList; ```
In this code, the UserList component accepts a ‘users’ prop, which is an array of user objects. It then maps over this array, creating a new UserCard component for each user.
2.2 UserCard Component
This component contains UserName and UserDetail components:
```jsx import React from 'react'; import { View } from 'react-native'; import UserName from './UserName'; import UserDetail from './UserDetail'; const UserCard = ({ user }) => { return ( <View> <UserName name={user.name} /> <UserDetail detail={user.detail} /> </View> ); }; export default UserCard; ```
The UserCard component takes a ‘user’ prop and uses it to pass specific data down to the UserName and UserDetail components.
2.3 UserName and UserDetail Components
These are simple functional components displaying text:
```jsx import React from 'react'; import { Text } from 'react-native'; const UserName = ({ name }) => { return <Text>{name}</Text>; }; export default UserName; ``` ```jsx import React from 'react'; import { Text } from 'react-native'; const UserDetail = ({ detail }) => { return <Text>{detail}</Text>; }; export default UserDetail; ```
Each of these components takes a single prop and displays it as a Text component.
React Native’s component architecture offers an effective and intuitive way to build complex mobile applications. By understanding the fundamentals of functional and class components, and leveraging the power of component composition, developers can create flexible, reusable components that are easy to manage and maintain.
It is this precise understanding and ability to harness the strength of React Native’s component architecture that you should look for when you hire React Native developers. Such comprehension not only enhances code readability but also improves the scalability of your application, making it a key attribute of highly skilled React Native developers. Therefore, when hiring, ensure that your React Native developers can fully exploit this beneficial component architecture for optimum mobile application development.
3. Lifecycle of a Component
Class components in React Native have a lifecycle, which is a series of methods that get automatically called at different points during the component’s life in the application.
The lifecycle can be broken down into three main phases:
- Mounting – when the component is being created and inserted into the UI.
- Updating – when a component is being re-rendered as a result of changes to either its props or state.
- Unmounting – when the component is being removed from the UI.
Understanding these phases and their methods can be crucial for handling side effects in the application, such as API calls, timers, or manual DOM manipulation.
4. Built-In Components
React Native provides a suite of built-in components that map directly to native views. These are the primitives that allow developers to create a full-fledged UI. Some of these components include `View`, `Text`, `Image`, `ScrollView`, `StyleSheet`, `Button`, and more.
Each of these components has its own unique props and behavior. For example, `Button` provides a basic button component with a simple API, while `View` is a fundamental component for building UI, supporting style, layout, and accessibility controls.
Conclusion
React Native’s component-based architecture is one of the keys to its popularity and success, and a primary reason why many organizations choose to hire React Native developers. The components in React Native are modular, reusable, and easy to test, making it a joy for developers to use and collaborate with. This encapsulated, component-based design allows for improved productivity and maintainability, especially in larger teams.
For businesses, understanding the fundamentals of components and how they interact with each other is not just beneficial, but crucial, when you decide to hire React Native developers. This knowledge helps you gauge their expertise in managing the modularity and the synergy between components, setting them on the path to mastering React Native and driving successful mobile application development.
Table of Contents
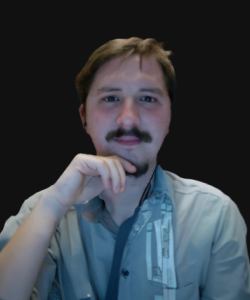
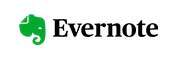