Creating Custom React Native Components for Reusability
In the fast-paced world of mobile app development, code reusability has become a paramount concern. React Native, a popular framework for building cross-platform mobile applications, provides a powerful toolset to create reusable components that enhance development efficiency, consistency, and maintainability. In this blog post, we will dive deep into the process of creating custom React Native components for improved reusability, accompanied by code samples and best practices.
Table of Contents
1. Why Custom Components Matter
Before we delve into the technical aspects, let’s discuss why custom components are a game-changer in React Native development.
1.1. Encapsulation and Abstraction
Custom components allow you to encapsulate complex UI logic, styles, and functionality into a single unit. This encapsulation enhances the separation of concerns, making your codebase more maintainable and less prone to bugs.
1.2. Consistency and Branding
By creating custom components that adhere to your app’s design guidelines, you ensure a consistent look and feel across your application. This is particularly crucial for branding and providing a polished user experience.
1.3. Time and Effort Efficiency
Once you’ve crafted a custom component, you can reuse it throughout your application or even across different projects. This dramatically reduces development time and effort, allowing you to focus on building unique features.
2. Step-by-Step Guide to Creating Custom Components
Let’s dive into the practical steps of creating custom React Native components.
2.1. Component Identification
Begin by identifying a piece of UI or functionality that you’ll likely use in multiple places within your app. This could be a button, a card, a navigation bar, or any other UI element.
2.2. Component Structure
Create a new directory within your project’s source code, specifically dedicated to your custom components. This directory structure promotes organization and separation from your main application logic.
2.3. Component Code
Inside the custom component directory, create a new JavaScript/TypeScript file for your component. Begin by importing necessary modules:
javascript import React, { PureComponent } from 'react'; import { View, Text, TouchableOpacity, StyleSheet } from 'react-native';
Next, define your component as a class or a functional component:
javascript class CustomButton extends PureComponent { render() { return ( <TouchableOpacity style={styles.button}> <Text style={styles.text}>Custom Button</Text> </TouchableOpacity> ); } }
2.4. Component Props
To make your component versatile, allow customization through props. Props are parameters that you pass to your component when you use it. For instance, in our custom button component, we can make the text and the button’s color customizable:
javascript class CustomButton extends PureComponent { render() { const { text, onPress, buttonColor } = this.props; return ( <TouchableOpacity style={[styles.button, { backgroundColor: buttonColor }]} onPress={onPress}> <Text style={styles.text}>{text}</Text> </TouchableOpacity> ); } } CustomButton.defaultProps = { buttonColor: '#3498db', // Default button color };
2.5. Styling
Define component-specific styles using the StyleSheet module:
javascript const styles = StyleSheet.create({ button: { padding: 10, borderRadius: 5, alignItems: 'center', justifyContent: 'center', }, text: { color: 'white', fontSize: 16, }, });
2.6. Using the Custom Component
Now that you’ve created your custom component, you can use it anywhere in your application:
javascript import React from 'react'; import { View, StyleSheet } from 'react-native'; import CustomButton from './path-to-your-custom-button'; const App = () => { return ( <View style={styles.container}> {/* Use the custom button component */} <CustomButton text="Press Me" onPress={() => alert('Button pressed!')} buttonColor="#e74c3c" /> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, }); export default App;
3. Best Practices for Creating Reusable Components
To ensure your custom React Native components are truly reusable and maintainable, consider these best practices:
3.1. Keep Components Small and Focused
Each custom component should have a specific purpose and focus. This promotes reusability and simplifies testing.
3.2. Utilize Props for Customization
Use props to allow flexibility in customizing your components’ appearance and behavior. This makes your components versatile across different use cases.
3.3. Document Your Components
Write clear documentation for your custom components, detailing their props, usage examples, and any special considerations. This will make it easier for other developers (or even your future self) to understand and utilize the components.
3.4. Test Thoroughly
Just like any other part of your application, custom components should be thoroughly tested. Implement unit tests and integration tests to catch potential issues early in the development process.
3.5. Version and Publish
Consider using a package manager like npm or yarn to version and publish your custom components as packages. This way, you can easily share them across different projects and even with the larger developer community.
Conclusion
Creating custom React Native components is a powerful technique for enhancing code reusability and improving development efficiency. By encapsulating UI logic and functionality into reusable units, you can achieve consistency, save time, and build applications more efficiently. Follow the step-by-step guide and best practices outlined in this blog post to create custom components that will elevate your React Native projects to new heights of reusability and maintainability. Happy coding!
Table of Contents
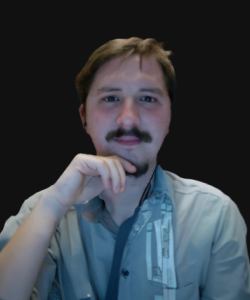
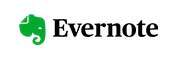