Creating Custom Animations in React Native
Animations breathe life into mobile applications, making them engaging and visually appealing. React Native, a popular framework for building cross-platform mobile apps, provides developers with powerful tools for creating custom animations. In this comprehensive guide, we’ll explore various techniques and strategies to create stunning animations in React Native, along with practical code examples.
Table of Contents
1. Why Custom Animations in React Native?
Before diving into the technical details, let’s understand why custom animations are essential for your React Native app.
1.1. Enhance User Experience
Custom animations provide a more immersive and enjoyable user experience. They can guide users through your app, convey information, and provide feedback, all while adding a touch of elegance.
1.2. Stand Out from the Crowd
In a sea of mobile apps, creating unique and eye-catching animations can set your app apart from the competition. Custom animations can help your app leave a lasting impression on users.
1.3. Express Brand Identity
Custom animations offer a creative way to express your brand’s identity. Whether it’s playful and whimsical or sleek and professional, animations can align with your brand’s personality.
1.4. Boost Engagement and Retention
Engaging animations can increase user retention rates by making your app more enjoyable to use. When users find your app visually appealing and fun, they’re more likely to keep coming back.
2. Setting Up Your React Native Environment
Before we jump into creating animations, make sure you have a React Native development environment set up. If you haven’t already, you can follow the official React Native documentation to get started.
3. Creating Basic Animations with Animated API
React Native’s Animated API is a powerful tool for creating animations. It provides a way to animate properties of React Native components smoothly. Let’s start with a basic example of animating a component’s opacity.
javascript import React, { Component } from 'react'; import { View, Animated, TouchableOpacity, Text } from 'react-native'; class FadeAnimation extends Component { constructor() { super(); this.state = { fadeAnim: new Animated.Value(0), // Initialize opacity to 0 (fully transparent) }; } componentDidMount() { Animated.timing( this.state.fadeAnim, { toValue: 1, // Animate to fully opaque duration: 1000, // Animation duration in milliseconds } ).start(); // Start the animation } render() { return ( <Animated.View style={{ opacity: this.state.fadeAnim, }} > <Text>This is a faded-in text.</Text> </Animated.View> ); } } export default FadeAnimation;
In this code snippet, we create a simple fade-in animation using the Animated API. We start by initializing the fadeAnim value to 0 (completely transparent) and then use the Animated.timing() function to animate it to a value of 1 (fully opaque) over a duration of 1000 milliseconds. The start() method triggers the animation.
4. Interpolating Animations
Interpolation allows you to create more complex animations by smoothly transitioning between different values. This technique is especially useful for animating multiple properties simultaneously.
javascript import React, { Component } from 'react'; import { View, Animated, Easing, Text } from 'react-native'; class InterpolationAnimation extends Component { constructor() { super(); this.state = { spinValue: new Animated.Value(0), scaleValue: new Animated.Value(0), }; } componentDidMount() { Animated.parallel([ Animated.timing( this.state.spinValue, { toValue: 1, duration: 1500, easing: Easing.linear, useNativeDriver: true, } ), Animated.timing( this.state.scaleValue, { toValue: 1, duration: 1000, easing: Easing.easeInOutBack, useNativeDriver: true, } ), ]).start(); } render() { const spin = this.state.spinValue.interpolate({ inputRange: [0, 1], outputRange: ['0deg', '360deg'], }); const scale = this.state.scaleValue.interpolate({ inputRange: [0, 0.5, 1], outputRange: [1, 2, 1], }); return ( <View> <Animated.View style={{ transform: [{ rotate: spin }, { scale: scale }], }} > <Text>Animated Text</Text> </Animated.View> </View> ); } } export default InterpolationAnimation;
In this example, we create an animation that combines rotation and scaling. We use Animated.parallel() to run both animations simultaneously. The interpolate() function is used to smoothly transition between values. The inputRange defines the range of input values, and the outputRange defines the corresponding output values.
5. Gesture-Based Animations
Gesture-based animations allow you to create interactive animations in response to user actions. React Native’s PanResponder can be used to capture touch gestures and update animations accordingly.
javascript import React, { Component } from 'react'; import { View, Animated, PanResponder } from 'react-native'; class GestureAnimation extends Component { constructor() { super(); this.state = { pan: new Animated.ValueXY(), }; this.panResponder = PanResponder.create({ onMoveShouldSetPanResponder: () => true, onPanResponderMove: Animated.event( [null, { dx: this.state.pan.x, dy: this.state.pan.y }], { useNativeDriver: false } ), onPanResponderRelease: () => { Animated.spring(this.state.pan, { toValue: { x: 0, y: 0 }, useNativeDriver: false, }).start(); }, }); } render() { return ( <View> <Animated.View {...this.panResponder.panHandlers} style={[ this.state.pan.getLayout(), { width: 100, height: 100, backgroundColor: 'blue', }, ]} /> </View> ); } } export default GestureAnimation;
In this example, we create a draggable element using PanResponder. The onMoveShouldSetPanResponder function allows the element to respond to touch events, onPanResponderMove updates the element’s position as it’s dragged, and onPanResponderRelease animates the element back to its initial position using Animated.spring().
6. Using Lottie for Complex Animations
For more complex animations, especially those involving vector graphics and multi-step animations, you can use Lottie. Lottie is a library that renders After Effects animations in real-time in React Native apps.
6.1. Installing Lottie
To get started with Lottie, install the required package:
bash npm install lottie-react-native
6.2. Using Lottie in React Native
Here’s a simple example of how to use Lottie in a React Native component:
javascript import React from 'react'; import { View } from 'react-native'; import LottieView from 'lottie-react-native'; const LottieAnimation = () => { return ( <View> <LottieView source={require('./animation.json')} // Replace with your animation file autoPlay loop /> </View> ); }; export default LottieAnimation;
In this example, we import the LottieView component and specify the animation source (replace ‘./animation.json’ with the path to your animation file). The autoPlay and loop properties control the animation’s behavior.
7. Performance Considerations
While animations can greatly enhance the user experience, it’s essential to consider performance, especially on mobile devices. Here are some tips to ensure smooth animations:
7.1. Use the Native Driver
Whenever possible, use the native driver for animations (useNativeDriver: true), as it offloads animation work to the native side, resulting in better performance.
7.2. Optimize Animations
Minimize the number of animations running simultaneously, and avoid heavy computations within animation functions. Optimize your animations for smooth execution.
7.3. Use RequestAnimationFrame
For complex animations or animations that involve interactions, consider using requestAnimationFrame for better control and performance.
7.4. Test on Real Devices
Always test your animations on real devices to ensure they perform well across different screen sizes and hardware configurations.
Conclusion
Custom animations can transform your React Native app from ordinary to extraordinary. Whether you’re creating simple fade-in effects or complex interactive animations, React Native provides the tools and libraries you need to bring your app to life. Remember to balance creativity with performance considerations to provide a seamless user experience. With these techniques and tips in hand, you’re ready to start crafting captivating animations that will delight your users and set your app apart.
Start experimenting with animations in React Native today, and watch your app come to life with vibrant, engaging, and user-friendly animations!
Table of Contents
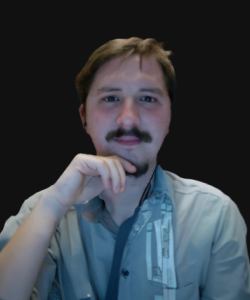
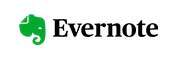