Creating Custom Hooks in React Native
React Native has gained immense popularity in the world of mobile app development due to its ability to build cross-platform apps with a single codebase. While React Native provides a rich set of built-in hooks, there are times when you need to encapsulate your custom logic for better code organization and reusability. This is where custom hooks come into play. In this blog post, we’ll explore how to create custom hooks in React Native, understand their benefits, and see practical examples.
Table of Contents
1. What Are Custom Hooks?
Custom hooks are JavaScript functions that encapsulate reusable logic in your React Native application. They are a powerful way to extract and share stateful logic and side effects across different components. Custom hooks follow the naming convention of starting with “use” to indicate that they are hooks.
Custom hooks offer several advantages:
- Code Reusability: You can encapsulate complex logic and reuse it in multiple components, reducing code duplication.
- Improved Readability: Custom hooks make your code more readable and maintainable by separating logic from the component.
- Efficiency: They help in managing stateful logic efficiently, preventing unnecessary re-renders.
Now, let’s dive into creating custom hooks step by step.
2. Creating a Custom Hook
To create a custom hook in React Native, follow these simple steps:
Step 1: Create a New File
Start by creating a new JavaScript file for your custom hook. Name it descriptively, starting with “use” to follow the hook naming convention. For example, if you’re creating a custom hook for handling form input, you can name it “useFormInput.js.”
Step 2: Define the Hook
Inside your custom hook file, define your hook function. This function can use any of the built-in hooks like useState, useEffect, or other custom hooks if needed. Let’s create a simple custom hook to toggle a boolean value:
javascript import { useState } from 'react'; function useToggle(initialValue = false) { const [value, setValue] = useState(initialValue); const toggle = () => { setValue(!value); }; return [value, toggle]; } export default useToggle;
In this example, we’ve created a useToggle hook that manages a boolean state and provides a toggle function to toggle its value.
Step 3: Use the Custom Hook
Now that you’ve defined your custom hook, you can use it in your React Native components. Import the hook at the top of your component file and use it as you would with any other hook:
javascript import React from 'react'; import { View, Text, TouchableOpacity } from 'react-native'; import useToggle from './useToggle'; function MyComponent() { const [isOn, toggle] = useToggle(false); return ( <View> <Text>{isOn ? 'ON' : 'OFF'}</Text> <TouchableOpacity onPress={toggle}> <Text>Toggle</Text> </TouchableOpacity> </View> ); } export default MyComponent;
In this example, the useToggle hook is used in the MyComponent to manage the state of a toggle switch.
3. Practical Custom Hook Examples
Let’s explore a few practical examples of custom hooks to see how they can simplify your React Native code.
3.1. Fetching Data
Fetching data from an API is a common task in mobile app development. You can create a custom hook to handle data fetching and state management:
javascript import { useState, useEffect } from 'react'; function useFetchData(url) { const [data, setData] = useState(null); const [isLoading, setIsLoading] = useState(true); useEffect(() => { fetch(url) .then((response) => response.json()) .then((result) => { setData(result); setIsLoading(false); }) .catch((error) => { console.error('Error fetching data:', error); setIsLoading(false); }); }, [url]); return { data, isLoading }; } export default useFetchData;
Now, you can easily fetch data in your components:
javascript import React from 'react'; import { View, Text } from 'react-native'; import useFetchData from './useFetchData'; function DataDisplay() { const { data, isLoading } = useFetchData('https://api.example.com/data'); if (isLoading) { return <Text>Loading...</Text>; } return ( <View> <Text>Data: {data}</Text> </View> ); } export default DataDisplay;
3.2. Theming
Managing app themes can be simplified with a custom hook. Here’s an example of a theming hook:
javascript import { useState } from 'react'; function useTheme() { const [theme, setTheme] = useState('light'); const toggleTheme = () => { setTheme(theme === 'light' ? 'dark' : 'light'); }; return { theme, toggleTheme }; } export default useTheme;
Now, you can easily switch themes in your app:
javascript import React from 'react'; import { View, Text, TouchableOpacity } from 'react-native'; import useTheme from './useTheme'; function ThemeSwitcher() { const { theme, toggleTheme } = useTheme(); return ( <View> <Text>Current Theme: {theme}</Text> <TouchableOpacity onPress={toggleTheme}> <Text>Toggle Theme</Text> </TouchableOpacity> </View> ); } export default ThemeSwitcher;
3.3. Form Handling
Handling form input can become more manageable with a custom hook. Here’s an example of a form input hook:
javascript import { useState } from 'react'; function useFormInput(initialValue = '') { const [value, setValue] = useState(initialValue); const handleChange = (text) => { setValue(text); }; return { value, onChangeText: handleChange }; } export default useFormInput;
Now, you can use this hook to handle form inputs:
javascript import React from 'react'; import { View, TextInput } from 'react-native'; import useFormInput from './useFormInput'; function MyForm() { const nameInput = useFormInput(''); return ( <View> <TextInput placeholder="Enter your name" value={nameInput.value} onChangeText={nameInput.onChangeText} /> </View> ); } export default MyForm;
4. Rules and Conventions for Custom Hooks
When creating custom hooks in React Native, there are some important rules and conventions to follow:
4.1. Start with “use”
Custom hooks should always start with the prefix “use” to make it clear that they are hooks.
4.2. Use Existing Hooks
Whenever possible, use existing built-in hooks like useState and useEffect within your custom hooks. This ensures consistency and compatibility with React Native’s lifecycle.
4.3. Keep Hooks Simple
Custom hooks should have a single responsibility and focus on one specific task. Avoid creating overly complex hooks that try to do too much.
4.4. Document Your Hooks
Provide clear documentation for your custom hooks, including their purpose, parameters, and return values. This makes it easier for other developers (and your future self) to understand and use the hooks.
4.5. Share Hooks
Consider sharing your custom hooks with the community through platforms like npm or GitHub. This can benefit other developers and lead to valuable feedback and improvements.
Conclusion
Custom hooks are a powerful tool in React Native that can greatly enhance code organization, reusability, and maintainability. By following the simple steps outlined in this blog post, you can create custom hooks for various purposes, from handling form input to managing data fetching and theming. Remember to adhere to best practices and conventions when creating custom hooks, and don’t hesitate to share your creations with the React Native community. Happy coding!
Table of Contents
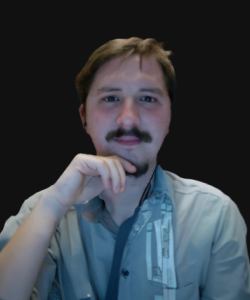
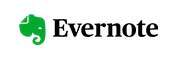