React Native and Maps: Customizing Map Markers and Overlays
In the realm of mobile app development, React Native stands out as a powerful framework for creating cross-platform applications with native-like performance. Integrating maps into React Native apps opens up a world of possibilities, from displaying location-based information to enhancing user experience with interactive features. One crucial aspect of map integration is customizing map markers and overlays to tailor the visual representation of data to your app’s needs. In this article, we’ll delve into the techniques for customizing map markers and overlays in React Native, along with practical examples to illustrate their implementation.
Customizing Map Markers
Map markers serve as visual indicators of specific locations on the map, helping users navigate and interact with the displayed data. React Native provides various methods for customizing map markers to match your app’s design aesthetic and convey relevant information effectively.
1. Changing Marker Icons
By default, React Native’s Marker component uses a standard icon to represent map markers. However, you can easily customize the marker icon by specifying a custom image or icon component. Here’s how you can do it:
import React from 'react'; import { Marker } from 'react-native-maps'; import { Image } from 'react-native'; import customMarkerIcon from './customMarkerIcon.png'; const CustomMarker = () => { return ( <Marker coordinate={{ latitude: 37.78825, longitude: -122.4324 }} title="Custom Marker" description="This is a custom marker" > <Image source={customMarkerIcon} style={{ width: 50, height: 50 }} /> </Marker> ); }; export default CustomMarker;
In this example, customMarkerIcon.png is a custom image imported and used as the marker icon.
2. Adding Custom Callouts
Callouts provide additional information when users interact with map markers. React Native’s Callout component allows you to customize the content and appearance of callouts associated with map markers. Here’s an example:
import React from 'react'; import { Marker, Callout } from 'react-native-maps'; import { Text } from 'react-native'; const CustomMarkerWithCallout = () => { return ( <Marker coordinate={{ latitude: 37.78825, longitude: -122.4324 }} title="Custom Marker" description="This is a custom marker with a callout" > <Callout> <Text>Custom Callout Content</Text> </Callout> </Marker> ); }; export default CustomMarkerWithCallout;
You can render any React component within the Callout component to display custom content.
Customizing Map Overlays
Map overlays are graphical elements overlaid onto the map surface to provide additional context or information. React Native supports various types of map overlays, including polygons, polylines, and circles, allowing for versatile customization options.
1. Drawing Polygons
Polygons are closed shapes defined by a series of coordinates, making them suitable for representing areas such as regions, boundaries, or routes on the map. Here’s how you can draw a polygon on the map:
import React from 'react'; import { Polygon } from 'react-native-maps'; const CustomPolygonOverlay = () => { const polygonCoordinates = [ { latitude: 37.8025259, longitude: -122.4351431 }, { latitude: 37.7896386, longitude: -122.421646 }, { latitude: 37.7665248, longitude: -122.4161628 }, { latitude: 37.7891195, longitude: -122.4027605 }, ]; return <Polygon coordinates={polygonCoordinates} fillColor="rgba(0, 0, 255, 0.5)" />; }; export default CustomPolygonOverlay;
In this example, polygonCoordinates define the vertices of the polygon, and fillColor specifies the color and opacity of the polygon fill.
2. Drawing Polylines
Polylines are sequences of connected line segments defined by a series of coordinates, commonly used to represent routes, paths, or boundaries on the map. Here’s how you can draw a polyline on the map:
import React from 'react'; import { Polyline } from 'react-native-maps'; const CustomPolylineOverlay = () => { const polylineCoordinates = [ { latitude: 37.8025259, longitude: -122.4351431 }, { latitude: 37.7896386, longitude: -122.421646 }, { latitude: 37.7665248, longitude: -122.4161628 }, { latitude: 37.7891195, longitude: -122.4027605 }, ]; return <Polyline coordinates={polylineCoordinates} strokeWidth={5} strokeColor="red" />; }; export default CustomPolylineOverlay;
Here, polylineCoordinates define the vertices of the polyline, and strokeWidth and strokeColor specify the thickness and color of the polyline, respectively.
Conclusion
Customizing map markers and overlays in React Native allows developers to create visually appealing and informative maps tailored to their app’s requirements. By leveraging the flexibility and versatility of React Native’s map components, you can enhance user experience and provide valuable location-based features in your mobile applications.
External Links:
Table of Contents
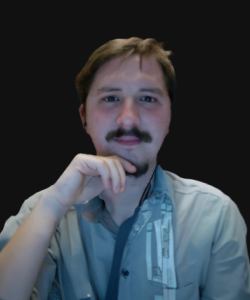
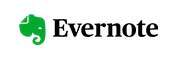