Conquer Debugging Challenges in React Native: Tips and Tricks for Developers
React Native has taken the world of mobile application development by storm, so much so that businesses are increasingly looking to hire React Native developers. Its unique ability to create near-native performance apps using JavaScript is a boon for developers who no longer need to juggle between Objective-C, Swift, or Java for iOS and Android apps, respectively. However, like every other technology, developing in React Native is not without its challenges. One primary issue that developers and organizations who hire React Native developers encounter is debugging their React Native applications. This blog post is dedicated to guiding both seasoned developers and those who are looking to hire React Native developers through the labyrinth of debugging React Native apps with a set of handy tips and tricks.
Understanding the Basics of Debugging in React Native
Before diving deep into debugging, it is important to understand what the term “debugging” actually implies. In the simplest terms, debugging is a process of identifying and resolving issues or bugs that prevent the software (in our case, a React Native application) from functioning correctly.
Debugging in React Native involves:
– Checking the code to identify any errors.
– Logging useful information that could help in diagnosing issues.
– Stepping through the code to see the flow and find where it deviates from what’s expected.
– Inspecting variables and state values while the code executes.
Now that we understand what debugging is and what it entails, let’s dive into some tips and tricks that will help you debug your React Native apps more effectively.
1. Use Console.log Wisely
One of the simplest yet most powerful tools in a developer’s arsenal is the `console.log()` function. While it might seem basic, it provides a wealth of information about the behavior of your application. Consider the following example:
```javascript fetch('https://myapi.com/data') .then((response) => response.json()) .then((data) => { console.log('Fetched Data:', data); }) .catch((error) => { console.error('Fetching Error:', error); }); ```
In the above code, `console.log()` is used to display the data fetched from the API. If the fetch operation fails, `console.error()` is used to print the error information. This can help identify if the issue is with the data fetched or with the fetch operation itself.
2. Leverage the Power of the React Native Debugger
The React Native Debugger is an open-source tool that combines the power of the JavaScript debugger, React Devtools, and Redux Devtools. It offers an interface to inspect and manage React and Redux state, view component hierarchies, and debug JavaScript code within a single window.
To start the debugger, run the command: `react-native debug` in your terminal. Then, in your app, open the developer menu (CMD+D on iOS, CMD+M on Android) and select ‘Debug JS Remotely’. Your app will now connect to the debugger.
3. Use Breakpoints Effectively
Breakpoints allow you to pause the execution of your code at a particular line. This enables you to inspect the values of variables and the application state at that specific point in time, which can provide significant clues about any bugs.
For instance, let’s say you’re looping through an array to perform some operation:
```javascript const numbers = [1, 2, 3, 4, 5]; let total = 0; numbers.forEach((number, index) => { total += number; // Add a breakpoint here }); ```
By adding a breakpoint inside the `forEach` loop, you can step through each iteration of the loop, inspecting the value of `total` and `number` at each step.
4. Inspect Network Requests with Chrome Developer Tools
Network requests are a common source of errors in applications. By using the Network tab in Chrome Developer Tools, you can inspect all network requests made by your application, including the request method (GET, POST, etc.), the status of the response, and the response body. This can be especially helpful for diagnosing issues with APIs or other backend services.
5. Keep an Eye on Error Messages and Stack Traces
React Native provides quite informative error messages and stack traces. Often, the error message provides a hint about the type of issue, while the stack trace shows exactly where the error originated in your code.
6. Use the `componentDidCatch` Lifecycle Method for Error Boundaries
React 16 introduced a new lifecycle method, `componentDidCatch`, which can be used to catch JavaScript errors anywhere in a component’s child tree and display a fallback UI instead of crashing.
```javascript class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } componentDidCatch(error, info) { this.setState({ hasError: true }); console.log('Error:', error); console.log('Info:', info.componentStack); } render() { if (this.state.hasError) { return <h1>Something went wrong.</h1>; } return this.props.children; } } ```
In the above example, any error in a child component will be caught by `componentDidCatch` and logged, and a fallback UI will be displayed.
7. Use the Performance Tab in React Developer Tools
React Devtools’ Performance tab allows you to record and inspect how your app renders. You can identify bottlenecks in your app’s render performance and see how your components mount, update, and unmount over time.
Conclusion
Debugging might seem overwhelming at first, especially in a dynamic and powerful framework like React Native. However, with the right strategies, tools, and a bit of practice, it becomes an integral and manageable part of the development process. This becomes even more efficient when you hire React Native developers who are skilled in these practices. By understanding the techniques outlined in this blog, developers can shorten the time it takes to hunt down and fix bugs, leading to smoother development experiences and more stable applications. Whether you’re a developer aiming to refine your skills or an organization seeking to hire React Native developers for smooth app development, understanding and applying these debugging strategies can prove highly beneficial. Happy debugging!
Table of Contents
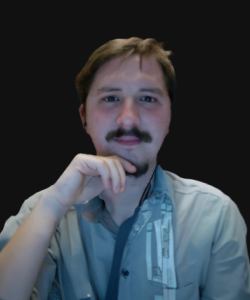
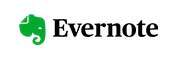