Debugging Performance Issues in React Native Apps
React Native has gained immense popularity for building cross-platform mobile applications, allowing developers to create efficient and performant apps using JavaScript. However, like any technology, React Native apps can encounter performance issues that can impact the user experience. Debugging these issues effectively is crucial to ensuring a smooth and responsive app. In this blog post, we’ll delve into various strategies, tips, and code samples to help you debug performance issues in your React Native applications.
Table of Contents
1. Understanding Performance Bottlenecks
Before diving into debugging, it’s important to understand the common performance bottlenecks that can affect React Native apps:
- Rendering and Reconciliation: React Native’s rendering mechanism updates the UI by performing a process called reconciliation. Excessive rendering, unnecessary re-renders, or a complex component tree can lead to performance degradation.
- JavaScript Execution: Heavy computation or running expensive operations on the JavaScript thread can slow down the app’s responsiveness, as it’s a single-threaded environment.
- Network and Data: Slow API calls or excessive data fetching can impact the app’s speed and responsiveness, leading to sluggish user interactions.
- Memory Usage: Improper memory management, memory leaks, and retaining unnecessary data in memory can lead to app crashes and slowdowns.
- UI Thread Blocking: Long-running operations on the UI thread, such as image decoding or layout calculations, can cause janky animations and unresponsive UI elements.
2. Profiling Tools
React Native provides several built-in tools for performance analysis and debugging:
2.1. React DevTools
React DevTools is a browser extension that allows you to inspect the component hierarchy, monitor state changes, and track component updates. It can be used for both React web and React Native apps. By analyzing component re-renders, you can identify unnecessary updates and optimize rendering.
2.2. Performance Monitor
The Performance Monitor is a tool included in React Native that helps you measure and visualize your app’s performance metrics. It provides insights into frame rates, CPU usage, memory consumption, and more. You can enable it using the following code:
javascript import { YellowBox } from 'react-native'; YellowBox.ignoreWarnings(['Remote debugger']); import PerfMonitor from 'react-native/Libraries/Performance/RCTRenderingPerf'; PerfMonitor.toggle();
2.3. Flipper
Flipper is a powerful debugging tool by Facebook that allows you to inspect the UI, network requests, and logs in your React Native app. It provides detailed information about the app’s behavior and can help you pinpoint performance issues.
3. Strategies for Debugging Performance Issues
Now that we’re familiar with the tools, let’s explore strategies for debugging performance issues in React Native apps:
3.1. Identify Reconciliation Problems
Excessive re-renders and unnecessary updates can significantly slow down your app. Use React DevTools or the Performance Monitor to identify components that are being re-rendered frequently. Optimize these components by implementing shouldComponentUpdate or using React’s memoization techniques.
javascript class MyComponent extends React.Component { shouldComponentUpdate(nextProps, nextState) { return this.props.someValue !== nextProps.someValue; } render() { // Component rendering logic } }
3.2. Avoid Costly Operations on the UI Thread
Performing heavy computations, image decoding, or other time-consuming tasks on the main UI thread can lead to unresponsive UI. Utilize Web Workers or libraries like react-native-fast-image to offload tasks to background threads.
3.3. Dealing with Expensive List Rendering
Rendering large lists can be a performance challenge. Consider using the FlatList component, which only renders the visible items and recycles views as the user scrolls. Implement the keyExtractor prop to help React Native efficiently manage the list updates.
javascript <FlatList data={data} keyExtractor={(item) => item.id.toString()} renderItem={({ item }) => <ListItem item={item} />} />
3.4. Memory Management and Garbage Collection
Improper memory management can lead to memory leaks and app crashes. Use tools like the Memory tab in the Performance Monitor or memory profiling in Flipper to identify memory consumption patterns. Release unused resources and objects by setting them to null when they are no longer needed.
javascript class MyComponent extends React.Component { componentDidMount() { this.timer = setInterval(() => { // Some logic }, 1000); } componentWillUnmount() { clearInterval(this.timer); this.timer = null; // Release the timer } render() { // Component rendering logic } }
3.5. Network and Data Optimizations
Slow network requests can significantly impact user experience. Use tools like Flipper to monitor network requests and their timings. Implement techniques such as caching, lazy loading, and optimizing data payloads to reduce network overhead.
Conclusion
In conclusion, debugging performance issues in React Native apps is a critical skill for delivering a seamless user experience. By understanding common performance bottlenecks, utilizing profiling tools, and implementing optimization strategies, you can create apps that are responsive, smooth, and performant. Remember that performance optimization is an ongoing process, and regular monitoring and fine-tuning are essential for maintaining a high-quality app. Happy coding!
Performance issues are a common challenge in React Native app development, but armed with the right knowledge and tools, you can effectively identify and address them. By understanding the potential bottlenecks, utilizing the built-in profiling tools, and implementing optimization strategies, you’ll be well-equipped to create high-performance React Native apps that provide a delightful user experience.
Table of Contents
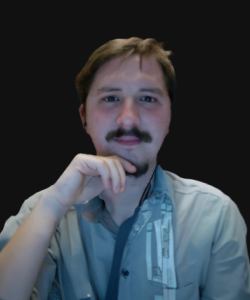
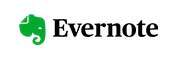