Deep Linking in React Native: Navigating to Specific App Screens
In the world of mobile app development, user experience plays a pivotal role in determining the success of an application. One aspect that significantly impacts user experience is seamless navigation. Users expect to be able to jump directly to a specific screen within an app, rather than having to navigate through multiple layers manually. This is where deep linking comes into play, especially in the context of React Native applications. In this article, we will explore the concept of deep linking and how it can be implemented effectively to enable precise screen navigation in React Native apps.
Table of Contents
1. Understanding Deep Linking
1.1. What is Deep Linking?
Deep linking is a technique that allows a user to navigate directly to a specific piece of content within a mobile application. This can be a particular screen, a product page, or any other resource that the app offers. Unlike regular links that lead to a website’s homepage, deep links take users to a targeted location within the app.
1.2. Why is Deep Linking Important?
Deep linking holds immense importance for both user convenience and marketing strategies. From a user’s perspective, it eliminates the hassle of manual navigation and provides a more streamlined experience. For businesses, deep linking enables targeted marketing campaigns by directing users to specific content, promotions, or products. Moreover, it bridges the gap between web and app interactions, allowing users to seamlessly switch between platforms while maintaining context.
2. Implementing Deep Linking in React Native
2.1. Setting Up a React Native Project
Before diving into deep linking, make sure you have a React Native project up and running. If you don’t have one, you can quickly set it up using the following commands:
bash npx react-native init DeepLinkingApp cd DeepLinkingApp
2.2. React Navigation Setup
To implement deep linking, we’ll utilize the popular library called React Navigation. It provides a flexible and easy-to-use navigation system for React Native apps. Install it using the following command:
bash npm install @react-navigation/native @react-navigation/stack
Next, let’s set up a basic stack navigator in the App.js file:
javascript import React from 'react'; import { NavigationContainer } from '@react-navigation/native'; import { createStackNavigator } from '@react-navigation/stack'; import HomeScreen from './screens/HomeScreen'; import DetailsScreen from './screens/DetailsScreen'; const Stack = createStackNavigator(); function App() { return ( <NavigationContainer> <Stack.Navigator initialRouteName="Home"> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Details" component={DetailsScreen} /> </Stack.Navigator> </NavigationContainer> ); } export default App;
2.3. Creating Navigable Screens
In this example, we have two screens: HomeScreen and DetailsScreen. For demonstration purposes, let’s assume the DetailsScreen displays information about a specific item. To create these screens, you can use the following template:
javascript // HomeScreen.js import React from 'react'; import { View, Button } from 'react-native'; const HomeScreen = ({ navigation }) => { return ( <View> <Button title="Go to Details" onPress={() => navigation.navigate('Details', { itemId: 123 })} /> </View> ); }; export default HomeScreen; // DetailsScreen.js import React from 'react'; import { View, Text } from 'react-native'; const DetailsScreen = ({ route }) => { const { itemId } = route.params; return ( <View> <Text>Details Screen</Text> <Text>Item ID: {itemId}</Text> </View> ); }; export default DetailsScreen;
In the HomeScreen, when the “Go to Details” button is pressed, we’re using the navigation.navigate method to navigate to the Details screen and passing a parameter (itemId) along with it.
2.4. Handling Deep Links
To handle deep linking, we need to configure the linking behavior in the index.js file:
javascript import { AppRegistry } from 'react-native'; import { getInitialURL } from 'react-native/Libraries/Linking/Linking'; import App from './App'; import { name as appName } from './app.json'; const handleDeepLink = async () => { const url = await getInitialURL(); if (url) { // Parse the URL and extract relevant information // Navigate to the appropriate screen based on the URL } }; getInitialURL().then((url) => { if (url) { handleDeepLink(); } else { AppRegistry.registerComponent(appName, () => App); } });
In the handleDeepLink function, you can parse the URL and extract any parameters or paths to determine the destination screen. Then, use the navigation methods provided by React Navigation to navigate to the intended screen.
3. Leveraging Deep Linking for Seamless Navigation
3.1. Navigating to Specific Screens
With the setup in place, your React Native app is now capable of deep linking. Users can access specific screens by clicking on deep links from various sources, such as messages, emails, or websites. This level of precision in navigation enhances the user experience and reduces friction.
3.2. Passing Parameters through Deep Links
One of the significant advantages of deep linking is the ability to pass parameters along with the link. This enables you to personalize the user’s experience further. In our example, we demonstrated passing the itemId parameter to the DetailsScreen. However, you can extend this to pass user IDs, product SKUs, or any other relevant data that helps in presenting targeted content.
3.3. Enhancing User Experience
Deep linking is not only about navigating users to specific screens; it’s also about creating a seamless transition between different touchpoints. Imagine a scenario where a user receives an email with a deep link to a limited-time offer. By clicking the link, the user is taken directly to the promotional screen within the app. This eliminates the need for the user to remember or search for the offer, resulting in a smoother user journey.
Conclusion
In conclusion, deep linking in React Native provides a powerful tool for enhancing user navigation and engagement within mobile applications. By allowing users to directly access specific screens and pass relevant parameters, developers can create a more personalized and seamless experience. Whether it’s for targeted marketing or improving usability, deep linking opens up a world of possibilities for creating a more connected app ecosystem.
Incorporating deep linking into your React Native app requires careful planning and consideration of user flows. However, the effort pays off in the form of improved user satisfaction, increased engagement, and a more efficient overall user experience. So, dive into the world of deep linking and unlock the full potential of your React Native applications.
Table of Contents
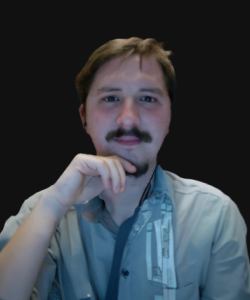
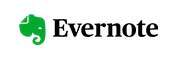