React Native and Expo: Simplifying Development and Deployment
In today’s fast-paced digital landscape, mobile app development has become a cornerstone for businesses and developers alike. However, creating mobile apps that run seamlessly across multiple platforms while maintaining a high level of development efficiency has always posed a significant challenge. Enter React Native and Expo, two game-changing technologies that have revolutionized the way developers approach mobile app development and deployment. In this article, we’ll delve into how React Native and Expo work together to simplify the development and deployment process, along with code samples and insights into their advantages.
Table of Contents
1. Understanding React Native and Expo
1.1. React Native: The Power of Native Experience
At its core, React Native is an open-source framework developed by Facebook that enables the creation of native mobile apps using JavaScript and React. What sets React Native apart is its ability to bridge the gap between native performance and the ease of web development. It achieves this by allowing developers to write components using a declarative syntax similar to React, while these components are then translated into native UI components. This approach ensures that the end product delivers a true native user experience, complete with smooth animations and seamless interactions.
1.1.1. Declarative UI Components
React Native’s declarative UI components simplify the process of building user interfaces. Here’s an example of a simple React Native component:
jsx import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; const App = () => { return ( <View style={styles.container}> <Text>Hello, React Native!</Text> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, }); export default App;
1.1.2. Hot Reloading for Swift Iterations
React Native’s hot reloading feature allows developers to instantly see the effects of their code changes without restarting the app. This greatly accelerates the development process, making it easier to iterate and refine the app’s interface and functionality.
1.2. Expo: Streamlined Development Workflow
Expo is a powerful set of tools and services built around React Native that simplifies various aspects of app development, from coding to deployment. It provides a unified workflow, eliminating the need for complex native setup and configuration.
1.2.1. Preconfigured Development Environment
Expo comes with a preconfigured development environment that eliminates the hassle of setting up simulators and emulators. Developers can start coding right away using Expo’s online editor or by setting up the Expo CLI locally.
1.2.2. Access to Native APIs
One of the challenges of mobile development is accessing native device features like the camera, geolocation, and push notifications. Expo abstracts the process by providing a set of JavaScript APIs that grant access to these native functionalities, without requiring developers to write native code.
javascript import { Camera } from 'expo-camera'; import * as Permissions from 'expo-permissions'; // Check camera permissions and capture a photo const takePhoto = async () => { const { status } = await Permissions.askAsync(Permissions.CAMERA); if (status === 'granted') { const { uri } = await Camera.takePictureAsync(); console.log('Photo taken:', uri); } };
1.2.3. Effortless Over-the-Air Updates
Expo makes updating apps a breeze with its over-the-air (OTA) update mechanism. This allows developers to push updates to users’ devices without requiring them to download a new version from an app store. This is particularly useful for quickly fixing bugs or deploying minor enhancements.
2. The Synergy Between React Native and Expo
2.1. Developing with Expo and React Native
Using React Native with Expo offers a comprehensive and streamlined development experience. Developers can take advantage of React Native’s native performance while leveraging Expo’s powerful tools for faster iteration and easier access to device capabilities.
2.1.1. Getting Started
To get started with Expo and React Native, follow these steps:
- Install Node.js and npm (Node Package Manager) if you haven’t already.
- Install the Expo CLI globally: npm install -g expo-cli
- Create a new Expo project: expo init MyProject
- Navigate to the project directory: cd MyProject
- Start the development server: expo start
2.1.2. Writing Code for React Native and Expo
With the project set up, you can start building your app using a combination of React Native and Expo APIs. Expo provides a wide range of components and APIs to simplify common tasks.
jsx import React from 'react'; import { View, Text, Button } from 'react-native'; import { Camera } from 'expo-camera'; import * as Permissions from 'expo-permissions'; const App = () => { const takePhoto = async () => { const { status } = await Permissions.askAsync(Permissions.CAMERA); if (status === 'granted') { const { uri } = await Camera.takePictureAsync(); console.log('Photo taken:', uri); } }; return ( <View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}> <Text>Hello, Expo and React Native!</Text> <Button title="Take Photo" onPress={takePhoto} /> </View> ); }; export default App;
2.2. Streamlined Deployment with Expo
Deploying mobile apps has traditionally been a complex process involving multiple steps, platform-specific configurations, and app store submissions. Expo simplifies this process by providing an easy way to deploy your app to both iOS and Android platforms.
2.2.1. Publishing Your App
To publish your Expo app, follow these steps:
Ensure you’re logged in to your Expo account in the terminal: expo login
Build a production-ready version of your app: expo build:android or expo build:ios
Once the build is complete, publish the app: expo publish
2.2.2. OTA Updates in Action
One of the standout features of Expo is its over-the-air update functionality. After publishing updates, users who have your app installed will receive the latest changes without needing to go through an app store update process.
3. Advantages and Considerations
3.1. Advantages of Using React Native and Expo
The combination of React Native and Expo offers several compelling advantages:
3.1.1. Cross-Platform Compatibility
With React Native, you can write code that works on both iOS and Android platforms, reducing development time and effort significantly.
3.1.2. Rapid Development Iterations
Expo’s hot reloading and instant updates make the development process incredibly fast and efficient.
3.1.3. Access to Native Features
Expo abstracts the complexities of native module integration, providing easy access to device features using JavaScript APIs.
3.1.4. Simplified Deployment
Expo’s streamlined deployment process removes the complexities of platform-specific configurations and app store submissions.
3.2. Considerations and Limitations
While React Native and Expo offer a plethora of benefits, it’s important to consider their limitations:
3.2.1. Custom Native Modules
For advanced use cases requiring custom native modules, React Native’s bridge might introduce performance overhead. In such cases, direct native development might be more suitable.
3.2.2. Limited Native Code Support
Expo abstracts native code access to ensure cross-platform compatibility. However, this means you might encounter limitations if you require low-level access to certain native functionalities.
3.2.3. OTA Update Risks
While OTA updates are convenient, they come with potential risks. A buggy update could negatively impact user experience and app stability.
Conclusion
React Native and Expo have ushered in a new era of mobile app development and deployment. The combination of React Native’s native-like performance and Expo’s streamlined workflow empowers developers to create powerful cross-platform apps with greater efficiency. From declarative UI components to simplified deployment, these technologies have significantly lowered the barriers to entry in the world of mobile app development. As the landscape continues to evolve, React Native and Expo remain at the forefront of the mobile development revolution, enabling developers to build incredible experiences that span across devices and platforms. So why wait? Dive into this powerful duo and unleash your creativity in the world of mobile apps!
In conclusion, React Native and Expo have transformed the way developers approach mobile app development and deployment. By combining the power of React Native’s native-like performance with Expo’s streamlined development workflow, developers can create cross-platform apps more efficiently than ever before. From writing declarative UI components to leveraging native APIs with ease, these technologies offer a wealth of benefits that simplify the development process. While there are considerations and limitations, the advantages far outweigh the drawbacks, making React Native and Expo a compelling choice for modern app development. So, whether you’re a seasoned developer or just starting, exploring React Native and Expo could be the key to unlocking a world of possibilities in the realm of mobile apps.
Table of Contents
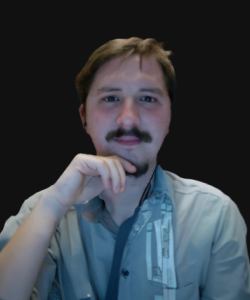
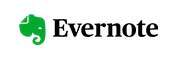