React Native and Firebase: Building Real-Time Apps with Cloud Integration
In today’s fast-paced world, mobile applications have become an integral part of our lives, serving as a bridge between technology and user experiences. Creating efficient, dynamic, and real-time mobile apps requires a potent blend of robust frameworks and seamless cloud integration. This is where the combination of React Native and Firebase shines. React Native offers a cross-platform framework for building native-like mobile apps using a single codebase, while Firebase provides a comprehensive suite of tools for cloud-based services, making real-time app development a breeze. In this blog post, we’ll delve into the world of React Native and Firebase, exploring how they come together to empower developers in crafting feature-rich, cloud-connected applications.
1. Understanding React Native and Firebase
1.1 What is React Native?
React Native is an open-source framework developed by Facebook that allows developers to build mobile applications using JavaScript and React. Unlike traditional mobile app development, where separate codebases are required for iOS and Android platforms, React Native enables developers to create a single codebase that works across both platforms, saving time and effort. It uses native components, ensuring a high-quality, native-like user experience.
1.2 What is Firebase?
Firebase, developed by Google, is a comprehensive mobile and web application development platform that provides a range of services to enhance app development. It offers real-time databases, user authentication, cloud functions, storage, and much more. Firebase’s real-time capabilities are particularly beneficial when creating apps that require instant data updates and synchronization across devices.
2. Setting Up Your Development Environment
2.1 Installing React Native
To get started, make sure you have Node.js and npm (Node Package Manager) installed. You can create a new React Native project using the following command:
bash npx react-native init YourApp
Navigate to your project directory:
bash cd YourApp
2.2 Creating a Firebase Project
- Go to the Firebase Console and sign in with your Google account.
- Click on “Add project” and follow the prompts to set up your project.
- Once your project is created, you’ll be directed to the project dashboard.
2.3 Integrating Firebase with React Native
Install the Firebase CLI:
bash npm install -g firebase-tools
Authenticate the Firebase CLI with your Google account:
bash firebase login
Initialize Firebase in your React Native project:
bash firebase init
Follow the prompts to select Firebase services and associate them with your project.
3. Real-Time Database with Firebase
3.1 Creating a Real-Time Database
The Firebase Realtime Database is a cloud-hosted NoSQL database that lets you store and sync data in real time. To set up the Realtime Database, head to the Firebase Console:
- Click on “Database” in the left sidebar.
- Choose “Create Database” and select “Start in test mode” (for simplicity in this example).
3.2 Reading and Writing Data
With your Realtime Database set up, you can start reading and writing data from your React Native app:
javascript import firebase from 'firebase/app'; import 'firebase/database'; // Initialize Firebase with your config firebase.initializeApp(yourConfig); // Write data firebase.database().ref('users/user1').set({ username: 'john_doe', email: 'john@example.com' }); // Read data firebase.database().ref('users/user1').on('value', snapshot => { const user = snapshot.val(); console.log('User:', user); });
3.3 Listening to Data Changes in Real Time
Firebase’s real-time capabilities shine when you need to update your app’s UI instantly based on changes in the database:
javascript firebase.database().ref('users/user1').on('value', snapshot => { const user = snapshot.val(); // Update UI with the new user data });
4. User Authentication and Firebase
4.1 Adding User Authentication to Your App
Firebase Authentication simplifies the process of adding user authentication to your React Native app. You can enable various sign-in methods, including email and password, Google, Facebook, and more.
4.2 Email and Password Authentication
To enable email and password authentication:
- In the Firebase Console, navigate to “Authentication” in the left sidebar.
- Choose the “Sign-in method” tab.
- Enable the “Email/Password” provider.
4.3 Social Media Authentication
To enable social media authentication:
- In the Firebase Console, navigate to “Authentication” and select the desired provider (e.g., Google or Facebook).
- Follow the setup instructions to configure the provider.
5. Cloud Functions for React Native
5.1 Introduction to Cloud Functions
Firebase Cloud Functions allow you to run custom backend code in response to events triggered by Firebase features and HTTPS requests. This is particularly useful for handling complex tasks, such as processing payments or sending notifications.
5.2 Integrating Cloud Functions with React Native
Set up your Cloud Functions environment:
bash firebase init functions
Deploy a sample function:
javascript // functions/index.js const functions = require('firebase-functions'); exports.helloWorld = functions.https.onRequest((request, response) => { response.send('Hello from Firebase!'); });
Deploy the function:
bash firebase deploy --only functions
5.3 Real-Time Triggers and Background Functions
You can create real-time triggers that run Cloud Functions in response to database changes:
javascript // functions/index.js exports.onUserDataUpdate = functions.database.ref('/users/{userId}').onUpdate(change => { // Perform actions when user data is updated });
6. Push Notifications with Firebase Cloud Messaging (FCM)
6.1 Enabling Push Notifications in Your App
To enable push notifications, you need to set up Firebase Cloud Messaging:
- In the Firebase Console, navigate to “Cloud Messaging” in the left sidebar.
- Follow the instructions to add your app and configure Firebase Cloud Messaging.
6.2 Sending Push Notifications from Firebase Console
- In the Firebase Console, go to “Cloud Messaging.”
- Click on “Send your first message.”
- Choose your target audience and compose your notification.
6.3 Handling Push Notifications in React Native
Integrate Firebase Cloud Messaging in your React Native app to receive and handle push notifications:
javascript import messaging from '@react-native-firebase/messaging'; // Request permission messaging().requestPermission().then(() => { // Permission granted }); // Handle incoming messages messaging().onMessage(remoteMessage => { // Handle the incoming message });
Conclusion
In conclusion, the integration of React Native and Firebase opens up a world of possibilities for building dynamic, real-time mobile applications with seamless cloud integration. From real-time databases to user authentication, cloud functions, and push notifications, this powerful combination equips developers with the tools they need to create cutting-edge apps that provide exceptional user experiences. Whether you’re a seasoned developer or just starting, exploring React Native and Firebase can be a game-changer in your mobile app development journey.
Table of Contents
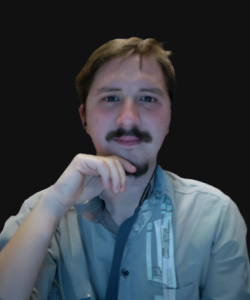
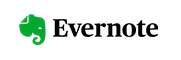