React Native and Firebase Authentication: User Authentication in Apps
In the ever-evolving landscape of app development, user authentication stands as a crucial pillar for securing user data and providing personalized experiences. React Native, with its cross-platform capabilities, coupled with Firebase Authentication, offers a robust solution for implementing user authentication seamlessly across various platforms. Let’s delve into how these two technologies work together to ensure secure and efficient user authentication in mobile apps.
Understanding React Native
React Native has gained immense popularity among developers for its ability to build native-like mobile applications using JavaScript and React. Its component-based architecture allows for code reusability and faster development cycles, making it a preferred choice for many app projects.
Firebase Authentication
Firebase Authentication, a product of Google’s Firebase platform, offers a comprehensive set of features for authenticating users in mobile and web applications. It provides various authentication methods, including email/password, phone number, social media logins, and more, simplifying the implementation of user authentication in apps.
Integration Steps
1. Setting up Firebase
Begin by creating a Firebase project and enabling Firebase Authentication in the Firebase console. Obtain the necessary configuration details, such as the Firebase SDK setup and authentication methods.
2. Installing React Native Firebase
Utilize the React Native Firebase library, a community-driven collection of Firebase modules for React Native, to seamlessly integrate Firebase services into your React Native app. Install the required modules using npm or yarn.
npm install @react-native-firebase/app @react-native-firebase/auth
3. Implementing Authentication Flows
Leverage the Firebase Authentication API within your React Native app to implement various authentication flows, such as user registration, login, password reset, and account management. Customize the authentication UI to align with your app’s design and user experience.
Example Code Snippet
import React, { useState } from 'react'; import { View, Button, TextInput, Alert } from 'react-native'; import auth from '@react-native-firebase/auth'; const AuthScreen = () => { const [email, setEmail] = useState(''); const [password, setPassword] = useState(''); const handleSignIn = async () => { try { const userCredential = await auth().signInWithEmailAndPassword(email, password); console.log('User signed in successfully!', userCredential.user); } catch (error) { Alert.alert('Error', error.message); } }; return ( <View> <TextInput placeholder="Email" value={email} onChangeText={setEmail} /> <TextInput placeholder="Password" secureTextEntry value={password} onChangeText={setPassword} /> <Button title="Sign In" onPress={handleSignIn} /> </View> ); }; export default AuthScreen;
Conclusion
React Native, in tandem with Firebase Authentication, offers a powerful solution for implementing user authentication in mobile apps. By following the integration steps and leveraging the Firebase Authentication API, developers can ensure secure and efficient authentication experiences for their app users.
External Resources
- React Native Firebase Documentation
- Firebase Authentication Documentation
- React Native Community GitHub Repository
Incorporating React Native and Firebase Authentication into your app development workflow empowers you to deliver seamless and secure authentication experiences, setting the foundation for building user-centric mobile applications.
Table of Contents
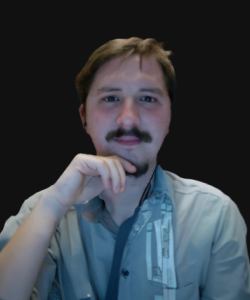
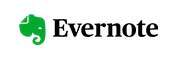