Implementing Firebase Cloud Messaging in React Native Apps
In today’s fast-paced world, real-time communication is essential for mobile applications. Firebase Cloud Messaging (FCM) is a powerful solution for adding push notification capabilities to your React Native apps. Whether you want to engage your users with timely updates or provide them with personalized content, FCM can help you achieve it seamlessly.
Table of Contents
In this comprehensive guide, we will walk you through the process of implementing Firebase Cloud Messaging in your React Native app. You’ll learn how to set up Firebase, handle push notifications, and send messages to your users in a few easy steps.
1. Prerequisites
Before we dive into the implementation, make sure you have the following prerequisites in place:
- Node.js and npm installed on your development machine.
- A React Native project set up and running.
- A Firebase account (you can create one at https://console.firebase.google.com/).
- Firebase CLI installed globally (npm install -g firebase-tools).
Step 1: Create a Firebase Project
The first step in implementing FCM is to create a Firebase project. Follow these steps:
- Go to the Firebase Console.
- Click on “Add project” and enter a name for your project.
- Click “Continue” and follow the on-screen instructions to set up your project.
- Once your project is created, click on “Add app” and select the platform (iOS or Android) you are developing for.
- Follow the setup instructions for your chosen platform, which will include downloading a configuration file (usually google-services.json for Android and GoogleService-Info.plist for iOS).
- Place the configuration file in the appropriate location in your React Native project.
Step 2: Initialize Firebase in Your React Native App
Now that you have created your Firebase project and added your app, it’s time to initialize Firebase in your React Native project.
Install the Firebase JavaScript SDK and React Native Firebase package by running the following command in your project directory:
bash npm install --save @react-native-firebase/app
Link the packages to your project:
bash npx pod-install
Initialize Firebase in your app by adding the following code to your app’s entry point (usually index.js or App.js):
javascript import { AppRegistry } from 'react-native'; import { name as appName } from './app.json'; import { App } from './App'; // Import your app component import { firebase } from '@react-native-firebase/app'; // Initialize Firebase firebase.initializeApp(); AppRegistry.registerComponent(appName, () => App);
Step 3: Request Permission for Push Notifications
To receive push notifications, you need to request permission from the user. Here’s how you can do it in React Native:
Install the @react-native-firebase/messaging package:
bash npm install --save @react-native-firebase/messaging
Request permission for push notifications in your app. You can create a function like this:
javascript import { messaging } from '@react-native-firebase/messaging'; async function requestPushNotificationPermission() { try { await messaging().requestPermission(); console.log('Notification permission granted'); } catch (error) { console.log('Notification permission denied'); } }
You can call this function when the user logs in or at an appropriate point in your app’s flow to request permission.
Step 4: Handle Incoming Notifications
Once you have permission to receive push notifications, you can handle incoming notifications in your app. Firebase Cloud Messaging allows you to customize how you handle these notifications based on your app’s needs.
Here’s a basic example of how to handle incoming notifications in React Native:
javascript import { useEffect } from 'react'; import { Alert } from 'react-native'; import messaging from '@react-native-firebase/messaging'; function App() { useEffect(() => { const unsubscribe = messaging().onMessage(async remoteMessage => { // Display a local notification Alert.alert( 'New Notification', remoteMessage.notification.body, ); }); return unsubscribe; }, []); // ... rest of your app component } export default App;
In this example, we use the onMessage event listener to handle incoming messages and display them as local notifications using Alert.alert(). You can customize the notification handling based on your app’s requirements, such as navigating to a specific screen or updating the UI.
Step 5: Send Push Notifications
Now that you can receive push notifications, let’s see how you can send them from the Firebase console or your server to your React Native app.
Sending Notifications from the Firebase Console
- Go to the Firebase Console.
- Select your project and click on “Cloud Messaging” in the left sidebar.
- Click the “Send your first message” button.
- Configure the notification message and target audience.
- Click “Next” and then “Review” to confirm the message details.
- Click “Publish” to send the notification to your app.
Sending Notifications from Your Server
You can also send notifications programmatically from your server to your React Native app using the Firebase Admin SDK or the Firebase Cloud Messaging API. Here’s an example of sending a notification using Node.js and the Firebase Admin SDK:
javascript const admin = require('firebase-admin'); const serviceAccount = require('path/to/serviceAccountKey.json'); admin.initializeApp({ credential: admin.credential.cert(serviceAccount), databaseURL: 'https://your-project-id.firebaseio.com', }); const message = { notification: { title: 'New Message', body: 'You have a new message!', }, token: 'device-token-here', // Replace with the device token of the recipient }; admin.messaging().send(message) .then((response) => { console.log('Successfully sent message:', response); }) .catch((error) => { console.log('Error sending message:', error); });
Make sure to replace ‘device-token-here’ with the actual device token of the user you want to send the notification to.
Step 6: Handle Notifications in the Background
To handle notifications in the background or when the app is closed, you can use Firebase Cloud Functions. Firebase Cloud Functions allow you to run server-side code in response to events like receiving a push notification.
Here’s a basic example of how to handle background notifications using Firebase Cloud Functions:
- Create a Firebase Cloud Function that triggers when a notification is sent.
- In your Cloud Function, handle the notification and perform the necessary actions, such as updating the database or sending a follow-up notification.
- Deploy your Cloud Function to Firebase.
- Configure your app to handle data-only notifications and react to the incoming data.
For more detailed information on handling background notifications with Firebase Cloud Functions, refer to the Firebase documentation.
Conclusion
Adding Firebase Cloud Messaging to your React Native app allows you to engage your users with real-time push notifications. In this guide, we’ve covered the essential steps to implement FCM, from setting up a Firebase project to handling incoming notifications and sending messages from both the Firebase Console and your server.
By following these steps and customizing the notification handling to fit your app’s needs, you can enhance user engagement and provide a more interactive experience for your app’s users. Stay connected with your audience, deliver timely updates, and keep them engaged with your React Native app using Firebase Cloud Messaging. Happy coding!
Table of Contents
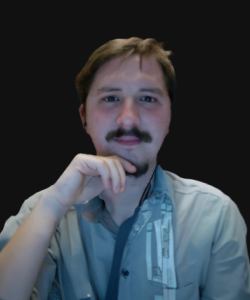
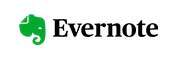