React Native Gesture Recognition: Implementing Touch Events
In the realm of mobile app development, creating engaging and interactive user interfaces is paramount. The ability to respond to user gestures like taps, swipes, and pinches not only enhances user experience but also adds a touch of elegance to your application. React Native, a popular framework for building native mobile apps using JavaScript and React, offers a robust set of tools for implementing touch gesture recognition. In this article, we’ll delve into the world of touch events in React Native, exploring their types and how to effectively utilize them to create intuitive touch interactions.
Table of Contents
1. Understanding Touch Events
Touch events are an essential aspect of mobile app development, enabling applications to respond to user interactions effectively. In React Native, touch events are essential building blocks for creating intuitive user interfaces. These events include:
1.1. Touch Start
The onTouchStart event is triggered when a user places their finger on the screen. This event is useful for detecting the initial touch point and can be used to initiate actions like highlighting elements or tracking gestures.
jsx <View onTouchStart={handleTouchStart}> {/* Your UI components */} </View>
1.2. Touch Move
The onTouchMove event is fired as the user’s finger moves across the screen after an initial touch. This event is commonly used for tracking gestures like swipes and scrolls.
jsx <View onTouchMove={handleTouchMove}> {/* Your UI components */} </View>
1.3. Touch End
The onTouchEnd event occurs when the user lifts their finger off the screen. It is commonly used to finalize actions triggered by touch events, such as navigation or confirming a selection.
jsx <View onTouchEnd={handleTouchEnd}> {/* Your UI components */} </View>
1.4. Touch Cancel
The onTouchCancel event is triggered when a touch event is interrupted or canceled, often due to system events like incoming calls. This event allows you to handle scenarios where touch interactions need to be aborted gracefully.
jsx <View onTouchCancel={handleTouchCancel}> {/* Your UI components */} </View>
2. Implementing Basic Touch Gestures
2.1. Tap Gesture
Taps are one of the most common touch gestures, used to trigger actions such as selecting an item or navigating to a new screen. Implementing tap gesture recognition in React Native involves utilizing the onPress event handler.
jsx <TouchableOpacity onPress={handleTap}> <Text>Tap me</Text> </TouchableOpacity>
2.2. Long Press Gesture
Long press gestures are ideal for invoking contextual actions or displaying additional information. React Native provides the onLongPress event handler to detect these gestures.
jsx <TouchableOpacity onLongPress={handleLongPress}> <Text>Long press me</Text> </TouchableOpacity>
3. Advanced Touch Interactions
3.1. Swipe Gesture
Swipes are powerful touch interactions used for actions like navigation or content dismissal. React Native doesn’t provide a dedicated swipe event, but you can implement swipe recognition using the combination of onTouchStart, onTouchMove, and onTouchEnd events.
jsx <View onTouchStart={handleSwipeStart} onTouchMove={handleSwipeMove} onTouchEnd={handleSwipeEnd} > {/* Swipeable content */} </View>
3.2. Pinch Gesture
Pinch gestures involve the user placing two fingers on the screen and either bringing them closer (pinch-in) or moving them apart (pinch-out). While React Native doesn’t offer a built-in pinch gesture, you can use the onTouchStart and onTouchMove events to calculate the distance between two touch points and determine the pinch action.
jsx <View onTouchStart={handlePinchStart} onTouchMove={handlePinchMove} onTouchEnd={handlePinchEnd} > {/* Scalable content */} </View>
4. Enhancing Gesture Recognition with Libraries
React Native also provides third-party libraries that simplify the implementation of complex gestures. Some popular options include:
4.1. react-native-gesture-handler
This library offers a more natural and efficient way to handle touch gestures. It provides components like PanGestureHandler and PinchGestureHandler that greatly simplify the implementation of gestures like dragging, swiping, and pinching.
jsx import { PanGestureHandler } from 'react-native-gesture-handler'; <PanGestureHandler onGestureEvent={handlePanGesture}> {/* Draggable content */} </PanGestureHandler>
4.2. react-native-swipe-gestures
For simple and customizable swipe gestures, the react-native-swipe-gestures library can be quite handy. It allows you to easily detect swipes in different directions and define corresponding actions.
jsx import GestureRecognizer from 'react-native-swipe-gestures'; <GestureRecognizer onSwipeLeft={handleSwipeLeft}> {/* Your UI components */} </GestureRecognizer>
Conclusion
In the world of mobile app development, touch gestures play a pivotal role in creating engaging and user-friendly interfaces. React Native equips developers with a robust set of tools to implement touch gesture recognition, from basic taps to more complex pinches and swipes. By harnessing the power of touch events and leveraging third-party libraries, developers can create applications that respond intuitively to users’ touch interactions, delivering exceptional user experiences. Whether you’re building a simple task app or a multimedia-rich platform, mastering touch gestures in React Native opens doors to endless possibilities for enhancing your mobile applications.
Table of Contents
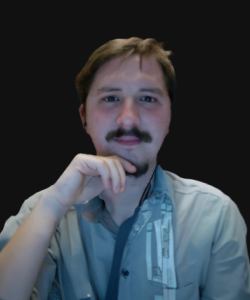
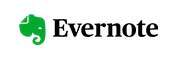