React Native and Google Sign-In: Integrating Google Authentication
In the world of mobile app development, user authentication is a critical aspect of ensuring security and personalization. With the rise of React Native as a popular framework for building cross-platform mobile applications, integrating third-party authentication services like Google Sign-In has become essential. In this guide, we’ll walk through the process of integrating Google Authentication into a React Native app, empowering you to leverage the user management capabilities provided by Google while delivering a seamless experience to your app users.
Why Google Sign-In?
Google Sign-In offers a convenient and secure way for users to sign in to your app using their Google credentials. By integrating Google Authentication, you can streamline the onboarding process for users while also gaining access to valuable user data provided by Google, such as their email address and profile information.
Prerequisites
Before diving into the integration process, ensure that you have the following prerequisites set up:
- React Native Project: Create a new React Native project or use an existing one.
- Google Cloud Console: Set up a project in the Google Cloud Console to obtain the necessary credentials for Google Sign-In.
- React Native Firebase: Install and set up React Native Firebase, which provides a convenient wrapper for Firebase services, including authentication.
Integration Steps
1. Install Dependencies
First, install the necessary dependencies for Google Sign-In using npm or Yarn:
npm install @react-native-firebase/auth @react-native-google-signin/google-signin
2. Configure Google Sign-In
Next, configure Google Sign-In in your React Native project by following the instructions provided by the @react-native-google-signin/google-signin package. This typically involves adding configuration files and linking native dependencies.
3. Implement Authentication Flow
Once Google Sign-In is configured, you can implement the authentication flow in your app. This typically involves the following steps:
- Display a “Sign In with Google” button in your app’s UI.
- Handle user interactions to initiate the Google Sign-In process.
- Use the Firebase Authentication API to authenticate users using Google Sign-In.
- Handle authentication callbacks to manage user sessions and update the UI accordingly.
4. Access User Data
After a successful sign-in, you can access user data provided by Google, such as the user’s email address, display name, and profile picture. This data can be used to personalize the user experience within your app.
Example Code
Below is a simplified example of how you can integrate Google Sign-In into your React Native app:
import React from 'react'; import { GoogleSignin, GoogleSigninButton, statusCodes } from '@react-native-google-signin/google-signin'; import auth from '@react-native-firebase/auth'; GoogleSignin.configure({ webClientId: 'your-web-client-id', }); const SignInScreen = () => { const signInWithGoogle = async () => { try { await GoogleSignin.hasPlayServices(); const { idToken } = await GoogleSignin.signIn(); const googleCredential = auth.GoogleAuthProvider.credential(idToken); await auth().signInWithCredential(googleCredential); } catch (error) { console.error('Google Sign-In Error:', error); } }; return ( <GoogleSigninButton style={{ width: 192, height: 48 }} size={GoogleSigninButton.Size.Wide} onPress={signInWithGoogle} /> ); }; export default SignInScreen;
Conclusion
Integrating Google Sign-In into your React Native app provides a seamless authentication experience for your users while also simplifying user management for your development team. By following the steps outlined in this guide and leveraging the power of React Native Firebase, you can quickly add Google Authentication to your app and focus on delivering value to your users.
External Resources:
- React Native Google Sign-In Documentation
- Firebase Authentication Documentation
- Google Cloud Console
Start integrating Google Sign-In into your React Native app today and unlock the full potential of user authentication and management. Happy coding!
Table of Contents
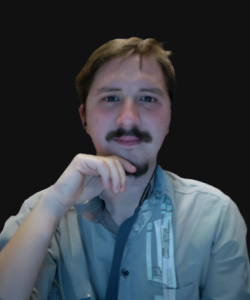
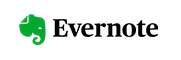