Integrating Payments in React Native Apps: Stripe and PayPal
In today’s digital age, integrating payment gateways into mobile applications has become a crucial aspect of providing a comprehensive user experience. Whether you’re building an e-commerce platform, a subscription-based service, or a donation app, enabling users to make payments seamlessly within your React Native app can significantly enhance user satisfaction and drive conversions. This tutorial will guide you through the process of integrating two popular payment gateways – Stripe and PayPal – into your React Native app.
Table of Contents
1. Getting Started with Stripe Integration
1.1. Setting Up Your Stripe Account
Before diving into the technical implementation, you’ll need to have a Stripe account. Head over to the Stripe website and sign up if you haven’t already. Once you have an account, you’ll be able to obtain the necessary API keys to process payments.
1.2. Installing Dependencies
To begin integrating Stripe into your React Native app, you’ll need to install the required packages. Open your terminal and navigate to your project directory. Run the following command to install the react-native-stripe-sdk package:
bash npm install react-native-stripe-sdk --save
1.3. Obtaining and Configuring Stripe API Keys
After installing the package, you’ll need to configure your Stripe API keys. Open your app’s configuration file (usually found in the src or config directory) and add the following code snippet:
javascript import { StripeProvider } from '@stripe/stripe-react-native'; // ... const stripePublishableKey = 'YOUR_STRIPE_PUBLISHABLE_KEY'; <StripeProvider publishableKey={stripePublishableKey}> {/* Your app components */} </StripeProvider>
Replace YOUR_STRIPE_PUBLISHABLE_KEY with your actual Stripe publishable key.
1.4. Creating a Payment Screen
Now that your app is set up with the Stripe provider, you can create a payment screen where users can enter their payment information. Use the CardField component provided by react-native-stripe-sdk to collect card details securely. Here’s an example of how you can create a simple payment screen:
javascript import React from 'react'; import { View, Text, Button } from 'react-native'; import { CardField, useStripe } from '@stripe/stripe-react-native'; const PaymentScreen = () => { const { handleCardAction, createPaymentMethod } = useStripe(); const handlePayment = async () => { const paymentMethod = await createPaymentMethod({ type: 'Card', }); if (paymentMethod) { const { error } = await handleCardAction(paymentMethod.id); if (error) { console.log('Payment failed:', error.message); } else { console.log('Payment successful!'); } } }; return ( <View> <Text>Enter your card information:</Text> <CardField postalCodeEnabled={false} placeholder={{ number: '4242 4242 4242 4242', }} /> <Button title="Make Payment" onPress={handlePayment} /> </View> ); }; export default PaymentScreen;
In this example, the handlePayment function creates a payment method using the entered card details and then initiates a payment using the handleCardAction function. If the payment is successful, the app logs a success message; otherwise, it logs an error message.
2. Integrating PayPal for Seamless Payments
2.1. Setting Up Your PayPal Developer Account
Before integrating PayPal, you need to sign up for a PayPal developer account if you don’t already have one. This account will allow you to obtain the necessary credentials for processing payments through PayPal.
2.2. Installing PayPal SDK
To integrate PayPal into your React Native app, you’ll need to install the react-native-paypal package. Run the following command in your terminal:
bash npm install react-native-paypal --save
2.3. Configuring PayPal Credentials
After installing the package, you’ll need to configure your PayPal credentials. Open your app’s configuration file and add the following lines:
javascript import PayPal from 'react-native-paypal'; // ... PayPal.initialize(PayPal.SANDBOX, 'YOUR_PAYPAL_CLIENT_ID');
Replace ‘YOUR_PAYPAL_CLIENT_ID’ with your actual PayPal client ID. You can obtain this client ID from your PayPal developer account.
2.4. Creating a PayPal Payment Button
With PayPal configured, you can now create a payment button that users can tap to initiate PayPal payments. Here’s an example:
javascript import React from 'react'; import { View, Text, Button } from 'react-native'; import PayPal from 'react-native-paypal'; const PayPalPaymentScreen = () => { const handlePayment = async () => { try { const confirmation = await PayPal.pay({ price: '10.00', currency: 'USD', description: 'Sample Payment', }); if (confirmation?.response?.state === 'approved') { console.log('Payment approved!'); } else { console.log('Payment not approved.'); } } catch (error) { console.log('Payment error:', error); } }; return ( <View> <Text>Tap the button below to make a PayPal payment:</Text> <Button title="Make PayPal Payment" onPress={handlePayment} /> </View> ); }; export default PayPalPaymentScreen;
In this example, the handlePayment function uses the PayPal.pay method to initiate a payment. If the payment is approved, a success message is logged; otherwise, an error message is logged.
Conclusion
Integrating payment gateways such as Stripe and PayPal into your React Native app opens up a world of opportunities to monetize your app, sell products, or accept donations. By following the steps outlined in this tutorial, you can provide your users with a seamless and secure payment experience, boosting user satisfaction and increasing conversions. Remember to always keep security in mind when handling sensitive payment information, and regularly update your app’s payment-related code to ensure compatibility with the latest SDK versions. Happy coding and successful payments!
Table of Contents
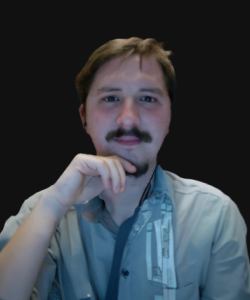
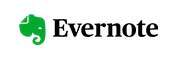