Integrating Social Media Sharing in React Native Apps
In the digital age, social media has become an integral part of our lives, connecting individuals and businesses across the globe. For developers, incorporating social media sharing functionality into mobile apps is a strategic move to enhance user engagement and increase the app’s reach. In this guide, we will delve into the process of integrating social media sharing capabilities into your React Native app, exploring various platforms and sharing types. Whether you’re a seasoned React Native developer or just starting, this step-by-step tutorial will equip you with the knowledge to effectively implement social media sharing features.
Table of Contents
1. Why Integrate Social Media Sharing?
The advantages of incorporating social media sharing features into your React Native app are manifold:
- Increased Exposure: Users sharing your app’s content expose it to a wider audience, potentially attracting new users.
- User Engagement: The ability to share content fosters a sense of involvement, encouraging users to spend more time within your app.
- Viral Potential: With the right content, your app can go viral as users share interesting or valuable information.
- Brand Promotion: Social media sharing facilitates organic brand promotion as users spread the word about your app.
- Data Insights: Monitoring shared content provides insights into user preferences and trends.
2. Prerequisites
Before diving into the integration process, ensure you have the following:
- Basic knowledge of React Native and JavaScript
- Node.js and npm installed on your development machine
- A code editor (e.g., Visual Studio Code)
- A React Native project set up using react-native-cli or Expo
3. Integrating Facebook Sharing
3.1. Setting Up a Facebook App
To enable Facebook sharing in your React Native app, you need to create a Facebook App and obtain an App ID. Follow these steps:
- Create a New App: Visit the Facebook for Developers portal and create a new app. Provide necessary details like the app name and contact email.
- Platform Selection: Choose “iOS” and “Android” as the platforms for your app.
- Bundle Identifier/Package Name: Enter your app’s bundle identifier (iOS) or package name (Android).
- Settings: Configure basic settings, add platform-specific details, and save changes.
- App ID: Once your app is created, you’ll receive an App ID. You’ll need this ID when configuring your React Native app.
3.2. Installing Dependencies
In your React Native project, install the react-native-fbsdk package to enable Facebook sharing:
bash npm install react-native-fbsdk --save
3.3. Implementing the Share Button
In your app’s component, import necessary modules and implement the Facebook share functionality:
javascript import React from 'react'; import { View, Text, TouchableOpacity } from 'react-native'; import { ShareDialog } from 'react-native-fbsdk'; const FacebookShareButton = () => { const shareLinkContent = { contentType: 'link', contentUrl: 'https://www.example.com', }; const shareOnFacebook = async () => { const canShow = await ShareDialog.canShow(shareLinkContent); if (canShow) { try { const result = await ShareDialog.show(shareLinkContent); if (result.isCancelled) { console.log('Sharing cancelled'); } else { console.log('Sharing successful'); } } catch (error) { console.log('Error sharing:', error); } } }; return ( <View> <TouchableOpacity onPress={shareOnFacebook}> <Text>Share on Facebook</Text> </TouchableOpacity> </View> ); }; export default FacebookShareButton;
By following these steps, your React Native app will have a functional Facebook sharing feature.
4. Integrating Twitter Sharing
4.1. Setting Up a Twitter App
To enable Twitter sharing, you need to create a Twitter Developer App and obtain API keys:
- Create a Twitter Developer Account: If you don’t have one, create a developer account at the Twitter Developer Portal.
- Create a New App: Once logged in, create a new Twitter app by providing necessary details.
- App Details: After creating the app, navigate to the “Keys and tokens” tab to access your API keys.
4.2. Installing Necessary Packages
Install the react-native-twitter package to facilitate Twitter sharing:
bash npm install react-native-twitter --save
4.3. Creating a Share Function
Implement Twitter sharing in your React Native component:
javascript import React from 'react'; import { View, Text, TouchableOpacity } from 'react-native'; import { TwitterShareButton } from 'react-native-twitter'; const TwitterShareButton = () => { const shareOnTwitter = async () => { try { const response = await TwitterShareButton.share({ text: 'Check out this amazing content!', url: 'https://www.example.com', }); if (response === 'success') { console.log('Sharing successful'); } else if (response === 'cancel') { console.log('Sharing cancelled'); } } catch (error) { console.log('Error sharing:', error); } }; return ( <View> <TouchableOpacity onPress={shareOnTwitter}> <Text>Share on Twitter</Text> </TouchableOpacity> </View> ); }; export default TwitterShareButton;
5. Integrating Instagram Sharing
5.1. Preparing for Instagram Sharing
Instagram sharing is a bit different, as it primarily allows sharing images and videos from your app to Instagram Stories. Users need to manually post the content to their Instagram feed.
User-Initiated Sharing: Instagram doesn’t provide a direct API for posting content to feeds. However, you can use a library like react-native-instagram-share to open the Instagram app with the shared content, allowing users to post it themselves.
Installing the Package: Install the react-native-instagram-share package:
bash npm install react-native-instagram-share --save
5.2. Constructing the Sharing Functionality
Implement Instagram sharing in your React Native component:
javascript import React from 'react'; import { View, Text, TouchableOpacity } from 'react-native'; import InstagramShare from 'react-native-instagram-share'; const InstagramShareButton = () => { const shareOnInstagram = async () => { try { const result = await InstagramShare.share({ type: 'image/jpeg', // Content type filename: 'image.jpg', // Your image filename // You might need to provide the full path to the image file }); if (result) { console.log('Shared to Instagram'); } else { console.log('Sharing cancelled'); } } catch (error) { console.log('Error sharing:', error); } }; return ( <View> <TouchableOpacity onPress={shareOnInstagram}> <Text>Share on Instagram</Text> </TouchableOpacity> </View> ); }; export default InstagramShareButton;
Conclusion
Incorporating social media sharing features into your React Native app can have a profound impact on user engagement and app visibility. By following the steps outlined in this guide, you can seamlessly integrate sharing functionality for Facebook, Twitter, and even Instagram. Remember to test thoroughly on both iOS and Android devices to ensure a consistent and user-friendly experience. Empower your users to share exciting content, thereby expanding your app’s reach and fostering a vibrant user community. Happy coding and sharing!
Table of Contents
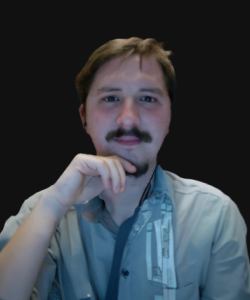
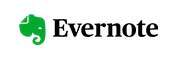