React Native and Notifications: Local and Remote Push Notifications
Push notifications have become an integral part of modern mobile applications, providing developers with a powerful tool to engage users and deliver timely information. In the realm of React Native, a popular framework for building cross-platform mobile apps using JavaScript, implementing both local and remote push notifications is essential to keep users informed and engaged. In this guide, we’ll delve into the world of push notifications and explore how to seamlessly integrate them into your React Native applications.
Table of Contents
1. Understanding Push Notifications
1.1. What are Push Notifications?
Push notifications are short messages sent to mobile devices, alerting users about new updates, events, or activities related to an application. These notifications can appear even when the app is not actively in use, making them a valuable communication channel for engaging users and driving them back to your app.
1.2. Benefits of Push Notifications in Mobile Apps
Push notifications offer several benefits to both app developers and users:
- Real-time Engagement: Push notifications enable developers to instantly communicate important information to users, fostering real-time engagement.
- User Retention: By reminding users about your app’s presence, you can boost user retention rates and prevent app abandonment.
- Personalization: Push notifications can be personalized based on user preferences and behaviors, leading to a more tailored user experience.
- Promotions and Updates: Developers can use push notifications to announce promotions, new features, and app updates.
- Direct Actions: Notifications can include actionable buttons, allowing users to take direct actions without opening the app.
2. Getting Started with React Native Push Notifications
2.1. Setting Up a React Native Project
Before diving into push notifications, ensure you have a React Native project ready. You can create a new project using the following command:
bash npx react-native init PushNotificationApp
Navigate to the project directory:
bash cd PushNotificationApp
2.2. Installing Dependencies
To implement push notifications in React Native, we’ll need to install the necessary packages. The two primary packages we’ll use are react-native-push-notification for local notifications and @react-native-firebase/app for remote notifications. Let’s install them:
bash npm install react-native-push-notification @react-native-firebase/app
After installing the packages, link the native modules by running:
bash npx react-native link react-native-push-notification
Note: If you’re using a version of React Native that’s 0.60 or newer, the linking process is automatic.
3. Implementing Local Push Notifications
3.1. Creating a Basic Notification
First, let’s implement a basic local push notification. Open the App.js file in your project and import the necessary modules:
javascript import PushNotification from 'react-native-push-notification';
Next, configure and initialize react-native-push-notification in your App.js:
javascript PushNotification.configure({ // Configuration options here });
Now, let’s create a simple local notification triggered by a button press:
javascript import React from 'react'; import { View, Button } from 'react-native'; const App = () => { const handleNotification = () => { PushNotification.localNotification({ title: 'Hello!', message: 'This is a local notification.', }); }; return ( <View> <Button title="Show Notification" onPress={handleNotification} /> </View> ); }; export default App;
Run your app using npx react-native run-android or npx react-native run-ios, and you’ll see a button that, when pressed, triggers a local notification.
3.2. Adding Custom Styling and Actions
Local notifications can be customized further with styles and actions. Modify the handleNotification function to include custom styling and actions:
javascript const handleNotification = () => { PushNotification.localNotification({ title: 'Important Meeting', message: 'You have a meeting at 2:00 PM.', largeIcon: 'ic_launcher', // Add your icon in the 'android/app/src/main/res/drawable' folder color: 'blue', // Notification color actions: ['Snooze', 'Dismiss'], }); };
In this example, we’ve added a custom large icon and notification color, along with two actions: “Snooze” and “Dismiss.” You can then handle these actions to perform specific tasks when the user interacts with the notification.
4. Integrating Remote Push Notifications
4.1. Configuring Firebase Cloud Messaging (FCM)
To enable remote push notifications, we’ll use Firebase Cloud Messaging (FCM) as our notification service provider. Follow these steps to set up FCM for your React Native project:
Create a Firebase project (if you don’t have one) on the Firebase Console.
Add a new Android or iOS app to your Firebase project and follow the setup instructions to integrate Firebase with your React Native project.
After setting up Firebase, you’ll need to retrieve the FCM server key and sender ID from your Firebase project settings. These will be used to send notifications to your app.
4.2. Handling Remote Notifications in React Native
With FCM set up, let’s integrate remote push notifications into your React Native app. Install the necessary package:
bash npm install @react-native-firebase/messaging
Initialize Firebase messaging in your App.js:
javascript import messaging from '@react-native-firebase/messaging'; messaging().setBackgroundMessageHandler(async remoteMessage => { console.log('Message handled in the background!', remoteMessage); });
The setBackgroundMessageHandler function allows your app to handle notifications even when it’s in the background or terminated.
5. Advanced Push Notification Features
5.1. Data Payloads and Customization
Remote push notifications can include data payloads that carry additional information. When sending a notification from your server, you can include custom data that’s relevant to the notification context. In your Firebase Cloud Messaging console, customize the notification payload with data fields.
json { "notification": { "title": "New Message", "body": "You have a new message", "sound": "default" }, "data": { "type": "message", "messageId": "123" } }
Handle the data payload in your app:
javascript messaging().onMessage(async remoteMessage => { const { type, messageId } = remoteMessage.data; // Handle the notification based on the data });
5.2. Handling Notification Interactions
Remote notifications can also include actions that users can take directly from the notification. Set up notification actions in the payload:
json { "notification": { "title": "New Message", "body": "You have a new message", "sound": "default" }, "data": { "type": "message", "messageId": "123" }, "actions": [ { "action": "reply", "title": "Reply" }, { "action": "dismiss", "title": "Dismiss" } ] }
Handle notification actions in your app:
javascript messaging().onNotificationOpenedApp(remoteMessage => { const { action } = remoteMessage; if (action === 'reply') { // Handle the reply action } else if (action === 'dismiss') { // Handle the dismiss action } });
5.3. Managing Notification Permissions
Before sending or receiving notifications, you need to request permission from the user. Use the requestPermission method:
javascript messaging().requestPermission();
You can also check the notification permission status using:
javascript const authStatus = await messaging().hasPermission();
6. Testing and Debugging Push Notifications
6.1. Testing Locally with Expo
For local testing, Expo offers an easy way to preview push notifications on a real device. Expo’s Notifications module provides a simple interface to send and receive notifications during development.
6.2. Remote Notification Testing
When testing remote notifications, you can use tools like the Firebase Cloud Messaging console or third-party services to send notifications to your app. Make sure to include the appropriate FCM server key and sender ID for your app to receive notifications.
7. Best Practices for Push Notifications
7.1. Personalization and Targeting
Segment your user base and send relevant notifications based on user preferences, behaviors, and interactions with your app. Personalized notifications have a higher chance of engaging users.
7.2. Frequency and Timing
Avoid overwhelming users with too many notifications. Respect their time and send notifications at appropriate times. Consider time zones and the user’s local time when scheduling notifications.
Conclusion
Push notifications are a potent tool to enhance user engagement and keep them connected to your React Native application. Whether through local notifications for timely reminders or remote notifications for real-time updates, mastering the art of push notifications can significantly improve the user experience. By following the steps and best practices outlined in this guide, you’re well on your way to creating compelling and effective push notifications in your React Native projects. Happy coding!
Table of Contents
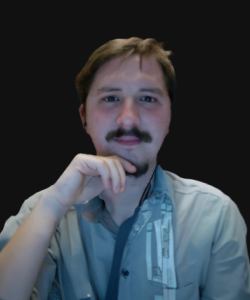
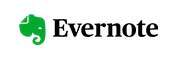