React Native and Machine Learning: Exploring ML in Mobile Apps
In the ever-evolving landscape of mobile app development, the integration of Machine Learning (ML) has emerged as a game-changer. Imagine having the power of predictive analytics, image recognition, and natural language processing right at your fingertips within your mobile app. This is where the fusion of React Native and Machine Learning comes into play, enabling developers to create smarter, more intuitive, and responsive applications. In this article, we will dive deep into the world of React Native and Machine Learning synergy, explore its possibilities, and learn how to integrate ML capabilities into your mobile apps.
Table of Contents
1. Understanding React Native and Its Advantages
1.1. What is React Native?
React Native is an open-source JavaScript framework developed by Facebook that allows developers to build mobile applications using a single codebase. This means you can write code once and deploy it on both iOS and Android platforms, reducing development time and effort. React Native utilizes native components, ensuring a smoother and more responsive user experience compared to web-based hybrid frameworks.
1.2. The Advantages of Using React Native
- Cross-Platform Compatibility: Building separate apps for iOS and Android can be time-consuming and costly. React Native solves this issue by enabling the development of cross-platform apps with a single codebase.
- Native Performance: React Native apps are not just web views wrapped in an app shell. They use native components, allowing them to achieve high performance levels comparable to apps built using native languages.
- Hot Reloading: With hot reloading, developers can instantly see the changes they make in the code on the app. This significantly speeds up the debugging and development process.
- Large Community and Ecosystem: React Native has a vast and active community, resulting in extensive documentation, numerous libraries, and third-party plugins that can be easily integrated into your app.
- Cost-Effective: Developing a single codebase for multiple platforms reduces development costs and shortens time-to-market.
2. Unleashing the Power of Machine Learning in Mobile Apps
2.1. The Role of Machine Learning in Apps
Machine Learning involves training algorithms to learn patterns from data and make predictions or decisions based on new inputs. In the context of mobile apps, ML can elevate user experiences by providing personalized recommendations, efficient search functionalities, and even enabling features like image and speech recognition.
2.2. Machine Learning Techniques and Applications
Image and Video Analysis: ML algorithms can be trained to recognize objects, faces, gestures, and even emotions within images and videos. This technology is extensively used in social media apps, security systems, and entertainment applications.
python # Example of image classification using TensorFlow.js const model = await tf.loadLayersModel('model/model.json'); const image = document.getElementById('image'); const tensor = tf.browser.fromPixels(image); const predictions = model.predict(tensor);
Natural Language Processing (NLP): NLP enables apps to understand and generate human language. It’s used in chatbots, language translation, sentiment analysis, and content summarization.
python # Example of sentiment analysis using Natural Language Toolkit (NLTK) in Python from nltk.sentiment import SentimentIntensityAnalyzer sia = SentimentIntensityAnalyzer() text = "I love using this app! It's so helpful and user-friendly." sentiment = sia.polarity_scores(text)
Predictive Analytics: Apps can leverage ML models to predict user behavior, preferences, or outcomes. This is widely seen in e-commerce apps for personalized product recommendations and in fitness apps for estimating calorie expenditure.
javascript // Example of user behavior prediction using scikit-learn in Python const model = new LogisticRegression(); model.fit(trainingData, labels); const predictedBehavior = model.predict(newUserData);
3. Integrating Machine Learning with React Native
3.1. Setting Up a React Native Project
Before integrating Machine Learning into your React Native app, ensure you have a working React Native project. You can set up a new project using the following commands:
bash npx react-native init MyMLApp cd MyMLApp
3.2. Choosing the Right ML Library
Selecting the appropriate ML library is crucial. TensorFlow and PyTorch are popular choices for complex ML tasks, while smaller libraries like ML Kit and Core ML are great for mobile-focused applications.
3.3. Incorporating ML Models into React Native Apps
Example: Sentiment Analysis in a Social Media App
Let’s consider a scenario where you want to perform sentiment analysis on user reviews in a social media app. You can follow these steps:
Collect and Label Data: Gather a dataset of user reviews labeled with their corresponding sentiments (positive, negative, neutral).
Train the Model: Use a machine learning library like TensorFlow to train a sentiment analysis model on the labeled dataset.
Convert and Integrate: Convert the trained model into a format compatible with React Native (such as TensorFlow.js) and integrate it into your app.
javascript // Example of using TensorFlow.js for sentiment analysis in React Native import * as tf from '@tensorflow/tfjs'; const model = await tf.loadLayersModel('model/model.json'); const userReview = "This app is amazing! I love it."; const encodedReview = preprocess(userReview); // Convert text to numerical data const prediction = model.predict(encodedReview); // Determine sentiment based on prediction const sentiment = prediction > 0.5 ? 'Positive' : 'Negative';
Example: Image Recognition in an E-Commerce App
In an e-commerce app, you might want to implement image recognition to allow users to search for products using images. Here’s a simplified version of how you could do this:
Train the Image Recognition Model: Use a library like TensorFlow or a pre-trained model to create an image recognition model that can classify products.
Integrate the Model: Convert the model into a format compatible with React Native and integrate it into your app.
javascript // Example of using TensorFlow.js for image recognition in React Native import * as tf from '@tensorflow/tfjs'; import { camera } from 'react-native-camera'; const model = await tf.loadLayersModel('model/model.json'); const image = await camera.takePicture(); const tensor = tf.browser.fromPixels(image); const prediction = model.predict(tensor); // Identify the product based on prediction const product = getProductFromPrediction(prediction);
4. Best Practices for Seamless Integration
4.1. Optimizing App Performance
- Model Size: Keep the size of your ML models as small as possible to prevent excessive memory usage and long loading times.
- On-Device vs. Cloud: Consider whether the ML processing should happen on-device or in the cloud. On-device processing offers privacy benefits and faster response times, while cloud processing can leverage more powerful servers.
4.2. Ensuring a Smooth User Experience
- Asynchronous Processing: Perform ML operations asynchronously to prevent freezing or slowing down the app’s UI.
- Loading States: Implement loading indicators to inform users when the app is processing ML tasks.
4.3. Handling Model Updates and Maintenance
- Version Control: Maintain version control for your ML models to ensure consistency across different app versions.
- Remote Updates: Consider implementing a mechanism to remotely update ML models without requiring users to update the entire app.
5. Future Trends and Possibilities
The integration of Machine Learning into React Native apps opens the door to countless possibilities:
5.1. Personalized User Experiences
Apps can adapt to user preferences and behavior, offering personalized content and recommendations.
5.2. Real-time Data Processing
ML-powered apps can process real-time data streams for instant decision-making and insights.
5.3. Enhanced Security Features
Implement advanced security measures, such as biometric authentication based on ML algorithms.
Conclusion
In conclusion, the marriage of React Native and Machine Learning empowers mobile app developers to create innovative and intelligent applications. By understanding the fundamentals of both technologies and following best practices, you can craft apps that provide personalized experiences and leverage the power of data-driven insights. As Machine Learning continues to evolve, the potential for enhancing mobile apps is limitless. So, take the plunge, experiment with integrating ML into your React Native projects, and embark on a journey of creating smarter, more capable mobile applications.
Table of Contents
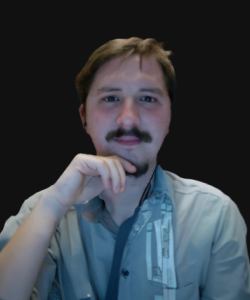
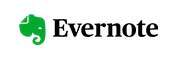