React Native and Native Modules: Integrating Native Code in Your App
In the world of app development, React Native has emerged as a game-changer by allowing developers to build cross-platform applications with a single codebase. However, there might be instances where you need to access native device functionalities that are beyond the scope of JavaScript. This is where the concept of “Native Modules” comes into play. In this article, we’ll delve into the process of integrating native code into your React Native app using Native Modules, exploring the benefits, steps, and providing code samples along the way.
1. Understanding the Need for Native Modules
React Native offers a rich set of pre-built components, allowing developers to create sophisticated UIs. However, there are instances where you might need to access device-specific features such as camera, GPS, or sensors that require native functionality. Native Modules act as a bridge between the JavaScript code and the native code, enabling seamless communication and interaction between the two.
1.1 Bridging the Gap between JavaScript and Native Code
React Native’s architecture allows you to write most of your app’s logic in JavaScript, making it easy to share code across different platforms. However, to access native features, you need a way to communicate with the underlying native code, written in languages like Java (for Android) or Swift/Objective-C (for iOS). Native Modules provide this bridge, enabling you to call native methods from your JavaScript code.
1.2 Leveraging Native Functionality
By integrating Native Modules, you can leverage the full power of device-specific features that might not be available through JavaScript alone. This is particularly beneficial when you want to create an app with a high degree of performance or one that requires access to hardware-level capabilities.
2. Creating Your First Native Module
Let’s dive into creating a basic Native Module to understand the integration process better.
2.1 Setting Up the Project
Before creating the Native Module, ensure you have a React Native project set up. If not, you can initialize one using:
bash npx react-native init NativeModuleExample
2.2 Creating the Native Module
First, let’s create a simple Native Module to perform a basic calculation. Navigate to the android/app/src/main/java/com/nativemoduleexample directory and create a new Java file named CalculatorModule.java. Here’s the code for the module:
java package com.nativemoduleexample; import com.facebook.react.bridge.ReactApplicationContext; import com.facebook.react.bridge.ReactContextBaseJavaModule; import com.facebook.react.bridge.ReactMethod; public class CalculatorModule extends ReactContextBaseJavaModule { public CalculatorModule(ReactApplicationContext reactContext) { super(reactContext); } @Override public String getName() { return "CalculatorModule"; } @ReactMethod public int add(int a, int b) { return a + b; } }
In this code, we’ve defined a simple module named CalculatorModule with a method add that takes two integers and returns their sum.
2.3 Bridging with JavaScript
To use this module in JavaScript, create a file named CalculatorModule.js in your project’s root directory:
javascript import { NativeModules } from 'react-native'; const { CalculatorModule } = NativeModules; export default CalculatorModule;
Now, you can import and use this module in your components:
javascript import React from 'react'; import { View, Text } from 'react-native'; import CalculatorModule from './CalculatorModule'; const App = () => { const sum = CalculatorModule.add(5, 3); return ( <View> <Text>Sum: {sum}</Text> </View> ); }; export default App;
In this example, the add method of the CalculatorModule Native Module is invoked to perform a calculation, and the result is displayed in the app’s UI.
3. Passing Data Between JavaScript and Native Modules
In more complex scenarios, you might need to pass data between JavaScript and Native Modules. Let’s explore how to achieve this.
3.1 Sending Data to Native Modules
Native Modules can accept parameters from JavaScript. Let’s enhance our CalculatorModule to accept an array of numbers and return their sum:
java // Inside CalculatorModule.java @ReactMethod public int addArray(ReadableArray numbers) { int sum = 0; for (int i = 0; i < numbers.size(); i++) { sum += numbers.getInt(i); } return sum; }
In this code, we’ve added a new method addArray that takes a ReadableArray (a data structure from React Native) containing numbers and returns their sum.
3.2 Receiving Data in JavaScript
To use this new method, modify the CalculatorModule.js file:
javascript // Inside CalculatorModule.js import { NativeModules } from 'react-native'; const { CalculatorModule } = NativeModules; export default { ...CalculatorModule, addArray: (numbers) => CalculatorModule.addArray(numbers), };
Now, you can use the addArray method in your components:
javascript // Inside your component const numbers = [2, 4, 6, 8]; const arraySum = CalculatorModule.addArray(numbers);
4. Handling Callbacks and Promises
Many native operations are asynchronous, such as accessing the camera or making network requests. To handle these cases, Native Modules offer callback functions or Promises.
4.1 Asynchronous Communication
Let’s modify our CalculatorModule to perform asynchronous addition using a callback:
java // Inside CalculatorModule.java @ReactMethod public void addAsync(int a, int b, Callback callback) { int sum = a + b; callback.invoke(null, sum); }
In this code, the addAsync method takes two integers and a Callback parameter. Once the calculation is done, the callback’s invoke method is used to send the result back to JavaScript.
4.2 Promises for a Cleaner Approach
Alternatively, you can use Promises for asynchronous operations. Here’s how you can modify the module:
java // Inside CalculatorModule.java @ReactMethod public void addPromise(int a, int b, Promise promise) { int sum = a + b; promise.resolve(sum); }
In this case, the addPromise method takes a Promise parameter and resolves it with the result.
5. Accessing Device Features: A Practical Example
Let’s walk through a real-world scenario where we integrate the camera functionality into a React Native app using Native Modules.
5.1 Integrating the Camera Module
Start by creating a new Native Module named CameraModule. This module will provide methods to open the camera, take photos, and access the camera feed.
java // Inside CameraModule.java // Import necessary packages public class CameraModule extends ReactContextBaseJavaModule { // Constructor and other methods @ReactMethod public void openCamera() { // Code to open the camera } @ReactMethod public void takePhoto(Promise promise) { // Code to capture a photo and resolve the promise with the image URI } }
In this example, the openCamera method is used to open the camera, and the takePhoto method captures a photo and resolves the provided Promise with the image URI.
5.2 Requesting Permissions
To access device features like the camera, you might need to request permissions from the user. Add the necessary permission requests in your AndroidManifest.xml and Info.plist files for Android and iOS respectively.
5.3 Displaying the Camera Feed
In your JavaScript code, you can now utilize the CameraModule to open the camera and display the camera feed in your app:
javascript // Inside your component import React from 'react'; import { View, Button } from 'react-native'; import CameraModule from './CameraModule'; const App = () => { const openCamera = () => { CameraModule.openCamera(); }; return ( <View> <Button title="Open Camera" onPress={openCamera} /> {/* Display the camera feed here */} </View> ); }; export default App;
By following this example, you can seamlessly integrate native device features like the camera into your React Native app.
6. Platform-Specific Implementations
React Native aims to provide a consistent development experience across platforms. However, there might be scenarios where you need to write platform-specific code to handle variations in behavior or appearance.
6.1 Writing Platform-Specific Code
To write platform-specific code, you can use the Platform module provided by React Native:
javascript import { Platform } from 'react-native'; if (Platform.OS === 'ios') { // Code specific to iOS } else if (Platform.OS === 'android') { // Code specific to Android }
This allows you to encapsulate platform-specific logic while maintaining a unified codebase.
6.2 Keeping the Codebase Consistent
While platform-specific code might be necessary at times, it’s generally recommended to keep the codebase as consistent as possible across platforms. This helps in code maintenance and ensures a smoother development experience.
7. Debugging and Troubleshooting
Integrating native code into your React Native app can introduce new challenges and potential issues. Here are some common problems and their solutions:
- Module Not Found Error: Ensure that the module name in your JavaScript code matches the module name in the native code.
- Type Mismatch Errors: Double-check data types when passing data between JavaScript and Native Modules.
- Native Code Compilation Issues: Make sure your native code compiles without errors and that you’ve linked the module correctly.
- Permissions and Security: Address any permission-related issues when accessing device features.
- Memory Leaks: Be cautious about memory management in native code to avoid memory leaks.
React Native provides debugging tools like the Chrome Developer Tools and React Native Debugger, which can assist in diagnosing and resolving issues.
Conclusion
Integrating native code through Native Modules allows you to extend the capabilities of your React Native app by tapping into platform-specific functionalities. Whether it’s accessing device features like the camera or handling complex calculations efficiently, Native Modules bridge the gap between JavaScript and native code seamlessly. By following the steps outlined in this guide and understanding the intricacies of Native Modules, you can create robust, feature-rich cross-platform applications that harness the best of both worlds. Happy coding!
Table of Contents
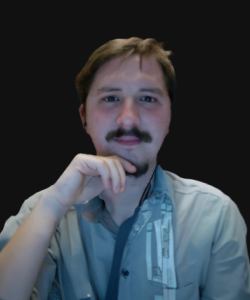
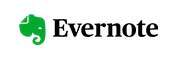