React Native Performance Profiling and Optimization Techniques
React Native has become a popular choice for building mobile applications, thanks to its ability to share code between iOS and Android platforms. However, as your app grows in complexity, you may start to encounter performance issues. Slow load times, sluggish animations, and unresponsive UIs can frustrate users and hinder the success of your app. To ensure your React Native app runs smoothly, it’s essential to dive into performance profiling and optimization techniques. In this blog, we’ll explore various strategies and tools to help you identify and address performance bottlenecks in your React Native applications.
Table of Contents
1. Understanding the Importance of Performance
1.1. Why Performance Matters
Performance is a crucial aspect of any mobile application. Users have high expectations when it comes to app responsiveness and speed. A slow app not only frustrates users but can lead to a high uninstall rate and negative reviews. Additionally, poor performance can impact user engagement, conversion rates, and overall user satisfaction. Therefore, ensuring that your React Native app performs well is essential for its success.
1.2. The Impact of Poor Performance
Poor performance can manifest in various ways, such as:
- Slow Startup Times: Long loading times can deter users from using your app.
- Laggy Animations: Choppy animations can make your app feel unpolished and unprofessional.
- Unresponsive UI: Delayed responses to user interactions can lead to frustration.
- High Battery Consumption: Inefficient apps drain device batteries quickly, leading to a negative user experience.
- Crashes and Freezes: Performance issues can result in crashes and app freezes, causing user data loss and irritation.
To avoid these problems, it’s crucial to proactively profile and optimize your React Native app.
2. Profiling Your React Native App
Profiling is the process of analyzing your application’s behavior and performance to identify bottlenecks and areas for improvement. React Native provides several tools and techniques to help you profile your app effectively.
2.1. React Native DevTools
React Native DevTools is an invaluable tool for profiling your app. It allows you to inspect the component hierarchy, view Redux actions, and monitor performance. To enable React Native DevTools, follow these steps:
- Install the DevTools package: npm install -g react-devtools
- Launch the DevTools: react-devtools
- Connect the DevTools to your running React Native app.
With React Native DevTools, you can identify which components are rendering and re-rendering unnecessarily, helping you optimize your app’s rendering performance.
2.2. Chrome DevTools
Chrome DevTools is another powerful tool for profiling React Native apps, especially for debugging JavaScript performance issues. To use Chrome DevTools:
- Run your React Native app in debug mode using react-native run-ios or react-native run-android.
- Open Google Chrome on your development machine.
- Press Ctrl+Shift+I to open Chrome DevTools.
- In Chrome DevTools, go to the “Sources” tab to debug and profile your JavaScript code.
- Use the “Performance” tab to record and analyze performance traces, including CPU and memory usage.
2.3. Performance Monitoring Tools
There are several third-party performance monitoring tools like Firebase Performance Monitoring and New Relic that can help you gain insights into your app’s performance in real-world scenarios. These tools provide metrics and analytics to identify performance bottlenecks, crashes, and user experience issues.
3. Identifying Performance Bottlenecks
Before optimizing your React Native app, you need to identify the specific areas that require improvement. Profiling can help you pinpoint performance bottlenecks in different aspects of your app.
3.1. CPU Profiling
CPU profiling helps you understand how your app utilizes the device’s CPU resources. You can use tools like React Native DevTools and Chrome DevTools to capture CPU profiles while interacting with your app. Look for functions or components that consume excessive CPU time and optimize them.
Here’s a code sample to capture a CPU profile using React Native DevTools:
javascript import { unstable_trace as trace } from 'scheduler/tracing'; function expensiveOperation() { // Perform some CPU-intensive operation here } function App() { return ( <button onClick={() => { trace('Button Click', performance.now(), () => { expensiveOperation(); }); }}> Click Me </button> ); }
3.2. Memory Profiling
Memory profiling helps you identify memory leaks and excessive memory consumption in your app. Tools like React Native DevTools and Chrome DevTools provide memory profiling capabilities. Pay attention to components or objects that are not properly cleaned up and are causing memory bloat.
Here’s a code sample to capture a memory profile using React Native DevTools:
javascript import { unstable_trace as trace } from 'scheduler/tracing'; function createMemoryLeak() { const array = []; for (let i = 0; i < 100000; i++) { array.push(i); } } function App() { return ( <button onClick={() => { trace('Button Click', performance.now(), () => { createMemoryLeak(); }); }}> Create Memory Leak </button> ); }
3.3. Network Profiling
Network profiling helps you optimize network requests and reduce unnecessary data transfers. You can use browser developer tools or third-party network monitoring tools to inspect API calls and their performance. Look for opportunities to optimize API endpoints, reduce the number of requests, and implement data caching strategies.
4. Optimization Techniques
Once you’ve identified performance bottlenecks, it’s time to implement optimization techniques to enhance your React Native app’s performance.
4.1. Code Splitting
Code splitting involves breaking your application code into smaller, more manageable chunks. By dynamically loading only the code needed for a specific route or feature, you can reduce the initial bundle size and improve app loading times.
React Native supports code splitting through tools like React Navigation and React Loadable. Here’s an example of code splitting with React Loadable:
javascript import Loadable from 'react-loadable'; const Home = Loadable({ loader: () => import('./Home'), loading: () => <Loading />, }); const About = Loadable({ loader: () => import('./About'), loading: () => <Loading />, });
4.2. Lazy Loading
Lazy loading is closely related to code splitting and involves loading assets, such as images and videos, only when they are visible on the screen. This reduces the initial load time of your app and improves the overall user experience.
React Native provides the react-lazyload library for lazy loading images. Here’s an example:
javascript import LazyLoad from 'react-lazyload'; function App() { return ( <div> <LazyLoad height={200} offset={100}> <img src="image.jpg" alt="Lazy Loaded Image" /> </LazyLoad> </div> ); }
4.3. Bundle Size Reduction
Reducing the size of your app’s JavaScript bundle is critical for faster loading times. You can achieve this by removing unused dependencies, optimizing imports, and minifying your code. Tools like Babel and Webpack can help you automate these processes.
Here’s a sample Webpack configuration to optimize bundle size:
javascript const TerserPlugin = require('terser-webpack-plugin'); module.exports = { // ... optimization: { minimize: true, minimizer: [new TerserPlugin()], }, };
4.4. Rendering Optimization
Optimizing rendering performance is essential for smooth animations and a responsive UI. React Native provides a PureComponent class and the shouldComponentUpdate method to prevent unnecessary re-renders. Additionally, consider using libraries like reselect for efficient data memoization.
Here’s an example of using PureComponent:
javascript class MyComponent extends PureComponent { // ... }
4.5. Native Modules Integration
For performance-critical tasks, consider integrating native modules written in Objective-C (iOS) or Java (Android). Native modules allow you to execute platform-specific code, bypassing the JavaScript bridge, and achieving better performance. React Native’s Native Modules API makes it easy to create and use native modules in your app.
5. Testing and Benchmarking
After implementing optimization techniques, it’s crucial to test and benchmark your React Native app to ensure that performance has improved and that you haven’t introduced new issues.
5.1. Setting Up Testing Environments
Set up testing environments for both iOS and Android to simulate real-world conditions. Emulators, simulators, and physical devices should be used to validate your app’s performance across different platforms and devices.
5.2. Performance Benchmarking
Use performance benchmarking tools like Lighthouse, PageSpeed Insights, and the React Native Benchmark suite to measure various aspects of your app’s performance, including loading times, rendering speed, and memory usage. Benchmarking provides quantitative data to track improvements and regressions over time.
5.3. Regression Testing
Implement regression testing to catch performance regressions early in the development process. Continuous integration (CI) pipelines can automate the process of running performance tests whenever code changes are pushed to the repository. Tools like Jest and Detox can help you write and run regression tests for your React Native app.
6. Best Practices for Sustainable Performance
Sustaining optimal performance is an ongoing process. Here are some best practices to ensure your React Native app maintains its performance over time:
6.1. Continuous Monitoring
Regularly monitor your app’s performance using profiling tools and performance monitoring services. Identify and address any new performance issues that arise as your app evolves.
6.3. Code Reviews
Incorporate performance reviews into your code review process. Encourage team members to review and discuss code changes that could impact performance, ensuring that performance considerations are part of the development workflow.
6.4. Regular Updates
Stay up to date with the latest React Native releases, libraries, and best practices. New updates often include performance improvements and bug fixes. Keeping your app’s dependencies current can help maintain optimal performance.
Conclusion
Performance profiling and optimization are essential steps in the development of a React Native app. By understanding the importance of performance, utilizing profiling tools, identifying bottlenecks, implementing optimization techniques, and following best practices, you can ensure that your app delivers a smooth and responsive user experience. Remember that performance optimization is an ongoing process, so regularly monitor and update your app to maintain its high performance standards. With these techniques and strategies in your toolkit, your React Native app can provide a lightning-fast experience for users on both iOS and Android platforms.
Table of Contents
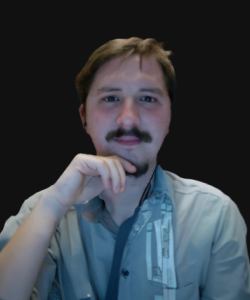
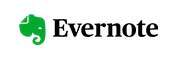