React Native Push Notifications: Engaging Users with App Notifications
In today’s competitive app landscape, user engagement is a crucial factor that can make or break the success of your mobile application. One of the most powerful tools at your disposal for enhancing user engagement is push notifications. Push notifications allow you to proactively reach out to your users with timely and relevant information, even when your app is not open. In this article, we will explore how React Native push notifications can be leveraged to engage users effectively and provide a seamless experience.
1. Understanding the Power of Push Notifications
Push notifications are short messages that can be sent to a user’s device, displaying as a banner, alert, or badge, regardless of whether the user is actively using the app. They are highly visible and can quickly capture a user’s attention, making them a perfect channel for delivering updates, reminders, personalized offers, and other relevant content.
When it comes to user engagement, push notifications offer several benefits:
- Timely Information: Push notifications enable you to keep your users informed about important updates in real-time. Whether it’s a breaking news story, a limited-time sale, or a new feature announcement, push notifications ensure that users are always in the loop.
- Re-engagement: Users may not use your app regularly, but push notifications can serve as gentle reminders to revisit it. By sending personalized and valuable content, you can entice users to re-engage with your app.
- Personalization: With the right data and segmentation, you can tailor push notifications to individual user preferences, increasing the chances of them finding the content relevant and valuable.
- Increased Conversions: Push notifications can drive conversions by nudging users to take specific actions, such as completing a purchase, reading an article, or watching a video.
2. Implementing Push Notifications in React Native
Now that we understand the significance of push notifications, let’s delve into implementing them in a React Native app. We’ll be using the popular library react-native-push-notification to achieve this. Follow these steps to get started:
Step 1: Installation
Begin by installing the react-native-push-notification package:
bash npm install react-native-push-notification --save
Step 2: Linking the Library
Link the library to your project using the following command:
bash react-native link react-native-push-notification
Step 3: Configuration
For both iOS and Android platforms, you’ll need to perform some additional configuration.
For iOS:
In your Xcode project, navigate to AppDelegate.m and add the following code:
objective #import <React/RCTPushNotificationManager.h> // Inside didFinishLaunchingWithOptions method if ([UNUserNotificationCenter class] != nil) { // iOS 10+ [UNUserNotificationCenter currentNotificationCenter].delegate = self; } [application registerForRemoteNotifications];
For Android:
In your android/app/src/main/java/com/yourapp/MainApplication.java file, add the following import:
java import com.dieam.reactnativepushnotification.ReactNativePushNotificationPackage;
And then add the package to the getPackages method:
java @Override protected List<ReactPackage> getPackages() { return Arrays.asList( // ... new ReactNativePushNotificationPackage() ); }
Step 4: Sending Push Notifications
With the setup in place, you can now send push notifications from your server using services like Firebase Cloud Messaging (FCM) or Apple Push Notification Service (APNs).
javascript import PushNotification from 'react-native-push-notification'; // Configure PushNotification PushNotification.configure({ onNotification: function (notification) { // Handle notification when received }, }); // Schedule a local notification PushNotification.localNotification({ title: 'Hello', message: 'This is a local notification', }); // Schedule a notification with data PushNotification.localNotification({ title: 'New Message', message: 'You have a new message', data: { sender: 'John' }, });
3. Best Practices for Effective Push Notifications
While implementing push notifications is a significant step towards engaging users, it’s essential to follow best practices to ensure you’re delivering value rather than annoyance:
3.1 Segmentation and Personalization
Sending generic notifications to all users might lead to opt-outs or uninstalls. Instead, segment your user base and send personalized notifications based on their preferences, behaviors, and demographics.
3.2 Timing Is Crucial
Timing plays a crucial role in the success of your notifications. Sending notifications at inappropriate times can lead to frustration. Consider time zones and the user’s local time when scheduling notifications.
3.3 Clear and Compelling Content
Craft concise and clear messages that communicate the value of the notification. Whether it’s a discount, a news update, or a social interaction, make sure the user understands why the notification is important.
3.4 A/B Testing
Experiment with different notification styles, content, and timing to understand what resonates best with your audience. A/B testing allows you to refine your approach and maximize engagement.
3.5 Opt-In and Opt-Out Mechanisms
Respect user preferences by allowing them to opt in or out of notifications easily. Provide clear instructions on how to manage notification settings within your app.
Conclusion
In the era of mobile apps, keeping users engaged is a challenge that requires strategic thinking and effective tools. React Native push notifications are a powerful instrument for driving user engagement, re-engagement, and conversions. By understanding the nuances of push notifications, implementing them correctly, and adhering to best practices, you can create a user experience that keeps your audience informed and delighted. Remember, the key lies in providing value and relevance through every notification you send.
Whether you’re a news app, an e-commerce platform, or a social network, push notifications can significantly impact your app’s success. So, take the time to explore the possibilities, experiment with different strategies, and watch as your app’s engagement metrics soar.
Table of Contents
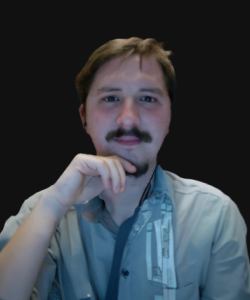
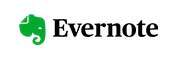