Building Real-Time Chat Apps with React Native and Socket.io
In today’s fast-paced digital world, real-time communication is a must-have feature for mobile applications. Whether you’re creating a messaging app, a customer support chat, or any other application that requires instant messaging capabilities, React Native and Socket.io are powerful tools to help you achieve real-time chat functionality.
Table of Contents
In this comprehensive guide, we’ll walk you through the process of building a real-time chat app using React Native and Socket.io. You’ll learn how to set up your development environment, create a chat interface, handle user authentication, and implement real-time messaging features. Let’s dive in!
1. Prerequisites
Before we get started, make sure you have the following prerequisites installed on your development machine:
- Node.js and npm (Node Package Manager)
- React Native CLI
- Expo CLI (optional but recommended for faster development)
- A code editor (e.g., Visual Studio Code)
If you haven’t already installed these tools, you can find installation instructions on their respective websites.
2. Setting Up Your Project
Let’s start by creating a new React Native project. Open your terminal and run the following command:
bash npx react-native init RealTimeChatApp
This command will create a new React Native project called “RealTimeChatApp.” Once the project is generated, navigate to its directory:
bash cd RealTimeChatApp
Now, let’s add Socket.io to our project by running:
bash npm install socket.io-client --save
We’ll also need a server for our Socket.io implementation. You can set up a Socket.io server using Node.js, but for this tutorial, we’ll use a hosted service called Heroku. If you prefer to run your server locally, you can find plenty of resources online to help you set it up.
3. Creating the Chat Interface
3.1. Building the User Interface
Before we dive into the Socket.io integration, let’s create the basic chat interface using React Native components. We’ll keep it simple with a text input field, a list of messages, and a send button.
jsx import React, { useState } from 'react'; import { View, TextInput, FlatList, Button, Text } from 'react-native'; const ChatScreen = () => { const [message, setMessage] = useState(''); const [messages, setMessages] = useState([]); const handleSendMessage = () => { // Implement message sending logic here }; return ( <View> <FlatList data={messages} keyExtractor={(item, index) => index.toString()} renderItem={({ item }) => <Text>{item}</Text>} /> <TextInput placeholder="Type your message" value={message} onChangeText={setMessage} /> <Button title="Send" onPress={handleSendMessage} /> </View> ); }; export default ChatScreen;
This code sets up the basic structure of our chat screen. The useState hook is used to manage the message input field and the list of messages. However, at this point, sending messages won’t work because we haven’t integrated Socket.io yet.
4. Integrating Socket.io for Real-Time Messaging
Socket.io allows us to establish real-time, bidirectional communication between the client and server. We’ll use it to send and receive messages instantly.
4.1. Connecting to the Socket.io Server
In your React Native component, import and initialize the Socket.io client:
jsx import io from 'socket.io-client'; const socket = io('https://your-socket-server-url.com');
Replace ‘https://your-socket-server-url.com’ with the URL of your Socket.io server.
4.2. Sending Messages
Now, let’s implement the logic to send messages when the user taps the “Send” button:
jsx const handleSendMessage = () => { if (message.trim() === '') { return; // Don't send empty messages } // Emit a 'chat message' event to the server socket.emit('chat message', message); // Update the local state to display the sent message setMessages([...messages, message]); // Clear the message input field setMessage(''); };
In the code above, we check if the message is not empty, emit a ‘chat message’ event to the server using Socket.io, update the local state to display the sent message, and clear the message input field.
4.3. Receiving Messages
To receive messages in real-time, we need to listen for incoming messages from the server. Modify your component to include the following code:
jsx import React, { useState, useEffect } from 'react'; // ... const ChatScreen = () => { // ... useEffect(() => { // Listen for 'chat message' events from the server socket.on('chat message', (msg) => { // Update the local state to display the received message setMessages([...messages, msg]); }); // Clean up the event listener when the component unmounts return () => { socket.off('chat message'); }; }, [messages]); // ... };
The useEffect hook listens for ‘chat message’ events from the server and updates the local state with the received messages. Don’t forget to clean up the event listener when the component unmounts to prevent memory leaks.
5. User Authentication
In most chat applications, user authentication is a crucial aspect. You want to ensure that users can only access their own chats and that messages are sent and received by the correct users. Let’s add basic user authentication to our chat app.
5.1. Setting Up User Authentication
You can implement user authentication in various ways, such as email/password authentication or third-party authentication providers like Google or Facebook. For simplicity, we’ll create a basic login screen where users can enter their username.
jsx import React, { useState } from 'react'; import { View, TextInput, Button } from 'react-native'; const AuthScreen = ({ onLogin }) => { const [username, setUsername] = useState(''); const handleLogin = () => { if (username.trim() === '') { return; // Don't allow empty usernames } // Pass the username to the parent component for authentication onLogin(username); }; return ( <View> <TextInput placeholder="Enter your username" value={username} onChangeText={setUsername} /> <Button title="Login" onPress={handleLogin} /> </View> ); }; export default AuthScreen;
This code defines an authentication screen where users can enter their username. When the “Login” button is pressed, the provided username is passed to the parent component for authentication.
5.2. Implementing User Authentication Logic
In your main application component, import the AuthScreen and include logic for handling user authentication:
jsx import React, { useState } from 'react'; import { View, TextInput, Button, Text } from 'react-native'; import AuthScreen from './AuthScreen'; // ... const App = () => { const [user, setUser] = useState(null); const handleLogin = (username) => { // Implement authentication logic here // For simplicity, we'll just set the user state with the username setUser(username); }; return ( <View> {user ? ( // Render the chat screen when a user is authenticated <ChatScreen user={user} /> ) : ( // Render the authentication screen when no user is authenticated <AuthScreen onLogin={handleLogin} /> )} </View> ); }; export default App;
In this code, we maintain a user state in the main application component. When a user successfully logs in, we set the user state with the provided username. This allows us to conditionally render the chat screen or the authentication screen based on the user’s authentication status.
6. Handling Multiple Chat Rooms
In real-world chat applications, users often have the option to join different chat rooms or have one-on-one conversations. To implement this feature, you can extend your application to support multiple chat rooms or private conversations.
7. Creating Chat Rooms
You can create chat rooms by defining them on the server and allowing users to join specific rooms. On the server-side, you’ll need to manage multiple Socket.io rooms and ensure that messages are broadcasted only to the users in the same room.
Here’s an example of how you can join a specific room on the client-side:
jsx // Join a room when a user clicks on it const handleJoinRoom = (roomName) => { // Leave the current room (if any) if (currentRoom) { socket.emit('leave room', currentRoom); } // Join the new room socket.emit('join room', roomName); setCurrentRoom(roomName); };
In this code, we emit ‘join room’ and ‘leave room’ events to the server to manage room memberships. The server should handle these events and update room memberships accordingly.
8. Displaying Room-Specific Messages
To display messages from a specific room, you can filter the messages based on the current room:
jsx const ChatScreen = ({ user, currentRoom }) => { // ... useEffect(() => { socket.on('chat message', (msg) => { // Filter messages based on the current room if (msg.room === currentRoom) { setMessages([...messages, msg]); } }); // Clean up the event listener when the component unmounts return () => { socket.off('chat message'); }; }, [messages, currentRoom]); // ... };
By filtering messages based on the current room, you can ensure that users only see messages from the room they’re in.
Conclusion
In this tutorial, we’ve covered the essential steps to build a real-time chat app with React Native and Socket.io. We started by setting up the project, creating the chat interface, and integrating Socket.io for real-time messaging. We also implemented basic user authentication and explored how to handle multiple chat rooms.
Building a full-fledged chat application involves more features like message persistence, push notifications, and user presence status. However, this guide provides a solid foundation for you to start building your real-time chat app. Feel free to expand on these concepts and explore additional features to make your chat app even more engaging and user-friendly.
Real-time chat apps are not only useful for social networking but also for enhancing customer support, team collaboration, and various other communication needs. With React Native and Socket.io, you have the power to create seamless, real-time experiences for your users.
Now that you’ve learned the basics, it’s time to dive deeper into the world of real-time communication and build your own innovative chat application. Happy coding!
Table of Contents
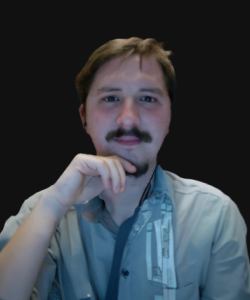
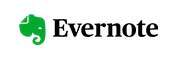