React Native and WebSockets: Real-Time Communication in Apps
In the ever-evolving world of mobile app development, user engagement is key. Users expect apps to provide real-time updates and interactive features. Whether it’s a chat application, a live sports score app, or a collaborative project management tool, real-time communication is becoming increasingly essential. That’s where WebSockets come into play.
Table of Contents
In this blog post, we will dive into the world of React Native and WebSockets, exploring how you can implement real-time communication in your mobile apps. We’ll cover the basics of WebSockets, how to integrate them into your React Native project, and provide you with practical examples and code snippets to get you started.
1. Understanding WebSockets
Before we jump into integrating WebSockets with React Native, let’s understand what WebSockets are and why they are essential for real-time communication.
1.1. What are WebSockets?
WebSockets are a communication protocol that provides full-duplex, bidirectional communication channels over a single TCP connection. Unlike traditional HTTP requests, which are stateless and require a new connection for each request, WebSockets allow continuous communication between a client and a server. This persistent connection enables real-time data exchange without the overhead of repeatedly establishing new connections.
1.2. Why use WebSockets?
WebSockets are the preferred choice for real-time communication in web and mobile applications for several reasons:
- Low Latency: WebSockets reduce latency by maintaining an open connection, ensuring that data can be sent and received instantly.
- Efficiency: Unlike polling or long-polling techniques, WebSockets are more efficient in terms of network and server resources.
- Bi-Directional: WebSockets enable two-way communication, making them suitable for chat applications, live updates, and collaborative features.
Now that we have a basic understanding of WebSockets, let’s see how we can integrate them into a React Native app.
2. Setting Up a React Native Project
If you don’t already have a React Native project, you can create one using the following commands:
bash # Install React Native CLI npm install -g react-native-cli # Create a new React Native project react-native init RealTimeApp
Once your project is set up, navigate to the project directory:
bash cd RealTimeApp
3. Adding WebSocket Support to Your React Native App
To enable WebSocket communication in your React Native app, you’ll need a library that simplifies WebSocket integration. One popular choice is the react-native-websocket library. Let’s add it to our project:
bash npm install react-native-websocket --save
Now that we have the library installed, we need to link it to our project:
bash react-native link react-native-websocket
4. Implementing WebSocket Communication
Let’s say you’re building a real-time chat application. Here’s a simplified example of how to implement WebSocket communication in React Native:
javascript import React, { Component } from 'react'; import { View, Text, TextInput, Button } from 'react-native'; import WebSocket from 'react-native-websocket'; class ChatApp extends Component { constructor(props) { super(props); this.state = { messages: [], message: '', }; this.socket = new WebSocket('wss://your-websocket-server.com'); this.socket.onopen = () => { console.log('WebSocket connected'); }; this.socket.onmessage = (e) => { const message = JSON.parse(e.data); this.setState((prevState) => ({ messages: [...prevState.messages, message], })); }; this.socket.onclose = () => { console.log('WebSocket closed'); }; } sendMessage = () => { if (this.state.message) { const message = { text: this.state.message, timestamp: new Date().getTime(), }; this.socket.send(JSON.stringify(message)); this.setState({ message: '' }); } }; render() { return ( <View> <View> {this.state.messages.map((message, index) => ( <Text key={index}>{message.text}</Text> ))} </View> <TextInput value={this.state.message} onChangeText={(message) => this.setState({ message })} /> <Button title="Send" onPress={this.sendMessage} /> </View> ); } } export default ChatApp;
In this example, we create a ChatApp component that establishes a WebSocket connection when the component mounts. It listens for incoming messages and updates the state to display the chat messages. Users can input messages through a TextInput, and when they click the “Send” button, the message is sent to the WebSocket server.
5. Handling Authentication and Error Handling
In a real-world application, you may need to implement authentication and handle errors gracefully. Additionally, it’s essential to manage the WebSocket connection’s lifecycle, ensuring that it reconnects if the connection is lost. You can use libraries like redux and redux-saga for more advanced state management and error handling.
6. Security Considerations
When working with WebSockets, it’s crucial to consider security. Here are some best practices:
- Use Secure Connections: Always use the wss:// protocol for WebSocket connections to encrypt data.
- Authentication: Implement proper authentication mechanisms to ensure that only authorized users can access your WebSocket server.
- Input Validation: Sanitize and validate incoming data to prevent security vulnerabilities like Cross-Site Scripting (XSS) attacks.
- Rate Limiting: Implement rate limiting to prevent abuse or denial-of-service attacks.
- Monitoring: Set up monitoring and logging to track and investigate any suspicious activity.
7. Scaling Your Real-Time App
As your real-time app grows, you may need to scale your WebSocket server to handle a larger number of concurrent connections. You can achieve this by:
- Load Balancing: Distributing incoming WebSocket connections across multiple servers to prevent overload.
- Caching: Caching frequently accessed data to reduce the load on your database.
- Horizontal Scaling: Adding more WebSocket server instances to handle increased traffic.
Conclusion
WebSockets are a powerful tool for adding real-time communication features to your React Native applications. Whether you’re building a chat app, a live sports score tracker, or a collaborative workspace, WebSockets enable you to deliver engaging user experiences with minimal latency.
In this blog post, we covered the basics of WebSockets, how to set up a React Native project, and how to integrate WebSocket communication using the react-native-websocket library. Remember to consider security best practices and scaling strategies as your app evolves.
Now that you have the knowledge and tools, go ahead and build your real-time React Native app with WebSockets, and take user engagement to the next level!
Table of Contents
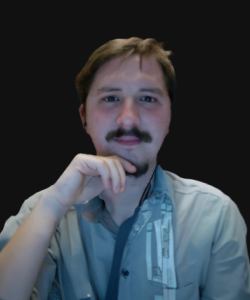
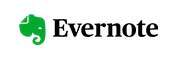