The Tale of Two Libraries: A Deep Dive into Redux and MobX for React Native State Management
State management in mobile applications is a critical component in the creation of dynamic and interactive user interfaces. It is a skillset highly sought-after when businesses look to hire React Native developers. In the realm of React Native, libraries such as Redux and MobX have emerged as popular tools for state management due to their robust functionality and flexibility. Any competent React Native developer should be well-versed with these tools. This post will explore how both Redux and MobX function in managing state within a React Native application, a vital consideration for those seeking to hire React Native developers, and will demonstrate examples to illustrate their usage.
1. Understanding State Management
To understand why Redux and MobX are important, it’s crucial to grasp the concept of state management. In a React Native application, “state” refers to the parts of the app which can change over time or due to interaction. It could be anything from a user’s login status to the contents of a shopping cart. The management of this state is crucial for the smooth and efficient operation of any application.
2. Redux: A Predictable State Container for JavaScript Apps
Redux is a popular open-source JavaScript library used for managing application state. It is often used with libraries like React and React Native for building user interfaces. Redux is renowned for its predictability in the application state, which becomes incredibly helpful when dealing with complex state transitions and when debugging applications.
Redux operates around a few core principles:
– Single source of truth: The state of your whole application is stored in a single state tree within a single store. This makes it easier to track changes over time and debug or inspect the application.
– State is read-only: The only way to change the state is to emit an action, an object describing what happened.
– Changes are made with pure functions: Reducers, which are pure functions, specify how the application’s state changes in response to actions sent to the store.
3. Redux in Action: A Simple Counter Application
Consider a simple counter application in React Native. The following code snippets demonstrate how we would implement this using Redux:
3.1. Setting up the Redux Store:
```js import { createStore } from 'redux'; const initialState = { counter: 0 }; const reducer = (state = initialState, action) => { switch(action.type) { case 'INCREMENT': return { counter: state.counter + 1 }; case 'DECREMENT': return { counter: state.counter - 1 }; default: return state; } }; const store = createStore(reducer); ```
3.2. Connecting React Native Components to the Store:
```js import { connect } from 'react-redux'; function Counter({ counter, dispatch }) { return ( <View> <Text>{counter}</Text> <Button title="Increment" onPress={() => dispatch({ type: 'INCREMENT' })} /> <Button title="Decrement" onPress={() => dispatch({ type: 'DECREMENT' })} /> </View> ); } const mapStateToProps = (state) => { return { counter: state.counter }; }; export default connect(mapStateToProps)(Counter); ```
The `connect` function connects the Redux store with the React Native component. Whenever the state changes, the component automatically re-renders with the new state.
4. MobX: Simple, Scalable State Management
MobX is another state management library for React Native applications that offers a slightly different approach to managing state compared to Redux. It’s renowned for its simplicity and scalability, treating your application state like a spreadsheet.
In MobX, you structure your application state as observable state trees. These observables can be updated by actions and subsequently can trigger reactions which automatically update the UI. This approach is fundamentally different from Redux’s “single state tree” approach, where state changes are triggered by dispatching actions to reducers.
5. MobX in Action: A Simple Counter Application
Let’s implement the same counter application using MobX:
5.1. Setting up the MobX Store:
```js import { makeAutoObservable } from "mobx" class CounterStore { counter = 0; constructor() { makeAutoObservable(this) } increment() { this.counter++; } decrement() { this.counter--; } } const counterStore = new CounterStore(); ```
5.2. Connecting React Native Components to the Store:
```js import { observer } from 'mobx-react'; const Counter = observer(({ counterStore }) => ( <View> <Text>{counterStore.counter}</Text> <Button title="Increment" onPress={() => counterStore.increment()} /> <Button title="Decrement" onPress={() => counterStore.decrement()} /> </View> )); export default () => <Counter counterStore={counterStore} />; ```
The `observer` function wraps the React Native component and automatically tracks which observables are used. Whenever an observable changes, the component is re-rendered.
Conclusion
Both Redux and MobX offer robust solutions to state management in React Native applications, shedding light on why businesses opt to hire React Native developers. Redux’s strict unidirectional data flow is excellent for larger applications where state changes can be complex and hard to trace, making it a favored choice among experienced React Native developers. On the other hand, MobX’s straightforward observability is a natural fit for smaller applications or for developers who prefer less boilerplate code.
It’s crucial to note that the decision between Redux and MobX isn’t binary. In certain projects, especially those handled by teams of hired React Native developers, both libraries can be used if the use-case requires. In the end, the art of state management is all about selecting the appropriate tool for the given task.
Table of Contents
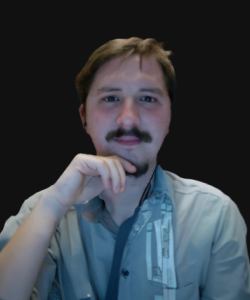
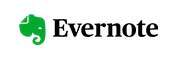