React Native and Redux Saga: Managing Side Effects in Your App
In the world of mobile app development, React Native has emerged as a powerful framework for building cross-platform applications with a native feel. One of the key challenges in mobile app development is handling side effects, such as making network requests, managing async actions, and handling complex state updates. Redux Saga comes to the rescue as a middleware library that simplifies handling side effects in a React Native app. In this blog post, we’ll explore how to effectively use Redux Saga to manage side effects in your React Native application.
Table of Contents
1. What Are Side Effects?
Side effects in the context of a React Native application are operations or interactions that occur outside of the normal flow of data within your app. These can include:
- Making API calls to fetch or send data.
- Handling asynchronous tasks like timeouts or intervals.
- Accessing and modifying browser storage.
- Navigating to different screens or routes.
- Managing animations or transitions.
These side effects can introduce complexity and potential issues in your application if not handled properly. Redux Saga is a library that provides a structured and efficient way to manage these side effects.
2. Setting Up Your React Native Project
Before we dive into using Redux Saga, let’s set up a basic React Native project if you haven’t already. You can use the following commands to create a new React Native project:
bash npx react-native init MyReduxSagaApp cd MyReduxSagaApp
Now that we have our project set up, let’s proceed with adding Redux Saga to it.
3. Installing Redux Saga
Redux Saga is designed to work seamlessly with Redux, so make sure you have Redux set up in your project. If not, you can follow the official Redux documentation to set it up.
To install Redux Saga, use npm or yarn:
bash npm install redux-saga # or yarn add redux-saga
Once you’ve installed Redux Saga, you need to configure it in your Redux store. Let’s create a basic Redux store configuration:
javascript // src/store/index.js import { createStore, applyMiddleware } from 'redux'; import createSagaMiddleware from 'redux-saga'; import rootReducer from './reducers'; import rootSaga from './sagas'; const sagaMiddleware = createSagaMiddleware(); const store = createStore( rootReducer, applyMiddleware(sagaMiddleware) ); sagaMiddleware.run(rootSaga); export default store;
In the code above, we import createSagaMiddleware from Redux Saga, combine it with our Redux store using applyMiddleware, and then run our root saga with sagaMiddleware.run(rootSaga).
4. Creating Your First Saga
Now that Redux Saga is set up, let’s create our first saga. A saga is a generator function that listens for specific actions and performs side effects when those actions are dispatched. Here’s an example of a simple saga that listens for a FETCH_DATA action and makes an API call:
javascript // src/sagas/dataSaga.js import { call, put, takeEvery } from 'redux-saga/effects'; import { fetchDataSuccess, fetchDataFailure } from '../actions/dataActions'; import { fetchDataFromAPI } from '../api'; function* fetchData(action) { try { const data = yield call(fetchDataFromAPI); yield put(fetchDataSuccess(data)); } catch (error) { yield put(fetchDataFailure(error)); } } function* dataSaga() { yield takeEvery('FETCH_DATA', fetchData); } export default dataSaga;
In this code:
- We import necessary functions from Redux Saga, such as call for making asynchronous calls, put for dispatching actions, and takeEvery for listening to actions.
- The fetchData generator function is responsible for making the API call and dispatching success or failure actions based on the result.
- The dataSaga generator function uses takeEvery to listen for FETCH_DATA actions and trigger the fetchData saga.
5. Dispatching Actions to Trigger Sagas
To trigger the FETCH_DATA saga, you’ll need to dispatch an action from your React component. Here’s an example of how you can do that:
javascript // src/components/DataComponent.js import React, { useEffect } from 'react'; import { connect } from 'react-redux'; import { fetchData } from '../actions/dataActions'; const DataComponent = ({ data, fetchData }) => { useEffect(() => { // Dispatch the FETCH_DATA action when the component mounts fetchData(); }, [fetchData]); return ( <div> {/* Render your data here */} </div> ); }; const mapStateToProps = (state) => ({ data: state.data, }); export default connect(mapStateToProps, { fetchData })(DataComponent);
In this example, we’re using the useEffect hook to dispatch the FETCH_DATA action when the DataComponent mounts. This action triggers our dataSaga, which, in turn, makes the API call and updates the Redux store with the fetched data.
6. Handling Asynchronous Operations
Redux Saga excels in handling asynchronous operations, such as delaying actions, making multiple API calls in sequence, or waiting for a specific condition before proceeding. Let’s look at some common scenarios:
6.1. Delaying Actions
You can delay an action by using the delay function from Redux Saga. Here’s an example of delaying an action for 2 seconds:
javascript import { delay } from 'redux-saga/effects'; function* delayedAction() { yield delay(2000); // Delay for 2 seconds yield put({ type: 'DELAYED_ACTION_SUCCESS' }); }
6.2. Making Sequential API Calls
To make API calls sequentially, you can use the call effect inside a loop. For example, if you need to fetch data from two different endpoints one after the other:
javascript function* fetchSequentialData() { try { const data1 = yield call(fetchDataFromAPI1); yield put({ type: 'FETCH_DATA_SUCCESS', data: data1 }); const data2 = yield call(fetchDataFromAPI2); yield put({ type: 'FETCH_DATA_SUCCESS', data: data2 }); } catch (error) { yield put({ type: 'FETCH_DATA_FAILURE', error }); } }
6.3. Waiting for a Condition
You can also use the take effect to wait for a specific action before proceeding. For example, waiting for a user to click a “Submit” button:
javascript import { take } from 'redux-saga/effects'; function* waitForSubmit() { yield take('SUBMIT_BUTTON_CLICKED'); // Continue with your logic after the button is clicked }
7. Error Handling in Redux Saga
Error handling is crucial in any application, and Redux Saga provides mechanisms for handling errors gracefully. In the earlier example, we already saw how to catch errors using try-catch blocks in sagas. Additionally, Redux Saga allows you to centralize error handling using the takeEvery and takeLatest functions.
takeEvery allows multiple instances of a saga to run concurrently. If an error occurs in one instance, it won’t affect others.
javascript function* watchFetchData() { yield takeEvery('FETCH_DATA', fetchData); }
takeLatest cancels any currently running instances of the saga and only keeps the latest one. This is useful for scenarios where you want to ensure that only the latest request is processed.
javascript function* watchFetchData() { yield takeLatest('FETCH_DATA', fetchData); }
8. Advanced Saga Features
Redux Saga offers several advanced features for handling complex side effects:
8.1. Selectors
Selectors allow you to extract data from the Redux store and use it in your sagas. This can be particularly helpful when you need to make decisions based on the current state of the application.
javascript import { select } from 'redux-saga/effects'; function* someSaga() { const isAuthenticated = yield select((state) => state.auth.isAuthenticated); if (isAuthenticated) { // Perform actions for authenticated users } else { // Perform actions for unauthenticated users } }
8.2. Forking Sagas
Forking sagas allows you to run sagas concurrently. This can be useful for scenarios where you want to perform multiple tasks independently.
javascript import { fork } from 'redux-saga/effects'; function* mainSaga() { yield fork(saga1); yield fork(saga2); }
8.3. Cancelling Sagas
You can cancel a running saga using the cancel effect. This is handy when you want to stop a long-running task, such as a data synchronization process, when the user navigates away from a screen.
javascript import { cancel, fork, take, race } from 'redux-saga/effects'; function* dataSyncSaga() { while (true) { yield take('START_DATA_SYNC'); const syncTask = yield fork(syncData); yield take('STOP_DATA_SYNC'); yield cancel(syncTask); } }
8.4. Testing Sagas
Testing sagas is essential to ensure that your side effects are working as expected. Redux Saga provides testing utilities to make this process easier. You can use libraries like redux-saga-test-plan or redux-saga-test-engine to write unit tests for your sagas.
Conclusion
Managing side effects in your React Native app is a critical aspect of building a robust and responsive application. Redux Saga simplifies this process by providing a structured and efficient way to handle asynchronous operations, API calls, and more. By using sagas, you can keep your application logic clean and maintainable, making it easier to scale and maintain your React Native project.
In this blog post, we covered the basics of Redux Saga, including setting up the library, creating sagas, triggering sagas with actions, handling asynchronous operations, and advanced features like error handling, selectors, forking, and cancellation. Armed with this knowledge, you can start building React Native apps that are more reliable and user-friendly.
Redux Saga is a powerful tool in your React Native development arsenal. As you delve deeper into the world of React Native and Redux Saga, you’ll find even more ways to optimize your app’s side effect management and provide a seamless user experience. Happy coding!
Table of Contents
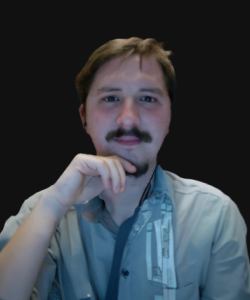
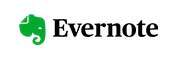