React Native and Stripe Payments: Integrating Payment Gateways
In today’s digital economy, seamless payment processing is crucial for any mobile application. Whether you’re running an e-commerce platform, a subscription-based service, or a marketplace app, integrating a reliable payment gateway is essential. For React Native developers, Stripe emerges as a powerhouse solution for handling transactions securely and efficiently.
Why Choose Stripe for React Native?
Stripe offers a developer-friendly platform with robust APIs and documentation, making it an ideal choice for integrating payment functionalities into React Native apps. Here are some key reasons why developers prefer Stripe:
1. Developer Experience
Stripe’s developer-first approach provides intuitive APIs, comprehensive documentation, and helpful tools, streamlining the integration process.
2. Security
Security is paramount when handling financial transactions. Stripe offers built-in security features such as PCI compliance, two-factor authentication, and advanced fraud detection, ensuring secure payment processing.
3. Flexibility
Whether you’re accepting one-time payments, recurring subscriptions, or implementing complex billing models, Stripe offers flexible solutions to meet diverse business needs.
Getting Started with React Native and Stripe Integration
Integrating Stripe into your React Native app involves a few simple steps:
1. Set Up Stripe Account
Before integrating Stripe, sign up for a Stripe account at Stripe. Once registered, navigate to the Dashboard to retrieve your API keys.
2. Install Stripe SDK
In your React Native project directory, install the @stripe/stripe-react-native package using npm or yarn:
npm install @stripe/stripe-react-native
Or
yarn add @stripe/stripe-react-native
3. Initialize Stripe
Initialize Stripe with your publishable API key in your app’s entry point (e.g., App.js):
import { StripeProvider } from '@stripe/stripe-react-native'; const App = () => { return ( <StripeProvider publishableKey="YOUR_PUBLISHABLE_KEY"> <RootComponent /> </StripeProvider> ); }; export default App;
4. Implement Payment Functionality
Use Stripe’s APIs to handle payment processing within your React Native components. For example, to create a payment method:
import { CardField, useStripe } from '@stripe/stripe-react-native'; const CheckoutScreen = () => { const { confirmPayment } = useStripe(); const handlePayment = async () => { const { paymentIntent, error } = await confirmPayment({ type: 'Card', billingDetails: { email: 'customer@example.com', }, }); if (error) { console.error('Payment failed:', error); } else { console.log('Payment successful:', paymentIntent); } }; return ( <View> <CardField postalCodeEnabled={true} placeholder={{ number: '4242 4242 4242 4242', }} cardStyle={{ backgroundColor: '#FFFFFF', textColor: '#000000', }} style={{ width: '100%', height: 50, marginVertical: 30, }} /> <Button title="Pay" onPress={handlePayment} /> </View> ); }; export default CheckoutScreen;
Real-World Examples
1. Shopify Mobile App
Shopify, a leading e-commerce platform, leverages React Native and Stripe to power payments in its mobile app, enabling merchants to accept payments seamlessly on the go.
2. TaskRabbit
TaskRabbit, a popular marketplace for outsourcing errands and tasks, integrates Stripe into its React Native app, facilitating secure and hassle-free transactions between users and taskers.
3. Instacart
Instacart, a grocery delivery service, utilizes React Native and Stripe to process payments within its mobile app, providing users with a convenient way to shop for groceries online.
Conclusion
Integrating Stripe payments into your React Native app empowers you to offer a frictionless payment experience to your users. With Stripe’s developer-friendly platform and robust features, you can securely handle transactions and drive business growth. Start integrating Stripe into your React Native app today and revolutionize your payment processing workflow.
Remember, while Stripe is an excellent choice for payment integration, always prioritize security and compliance to safeguard sensitive financial information and build trust with your users.
Happy coding and happy payments!
Disclaimer: The examples mentioned above are for illustrative purposes only and may not reflect the exact implementation details of the respective applications.
Table of Contents
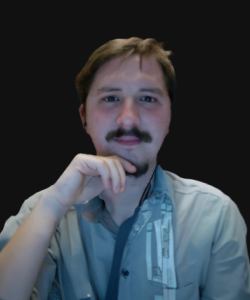
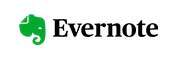