Testing React Native Apps: Strategies and Tools for Effective Testing
In today’s fast-paced world of app development, ensuring the quality and reliability of your React Native apps is crucial to delivering a seamless user experience. Robust testing practices not only catch bugs and issues early in the development process but also help maintain the app’s performance, stability, and overall user satisfaction. In this blog post, we’ll dive into the world of testing React Native apps, exploring essential strategies, tools, and best practices that can elevate your app development game.
1. Understanding the Importance of Testing in React Native Apps
When it comes to mobile app development, testing isn’t just a phase; it’s an ongoing process that directly impacts the success of your app. React Native, a popular framework for building cross-platform mobile apps, allows developers to create apps that look and feel native on both iOS and Android platforms. However, this versatility introduces complexities that can lead to subtle bugs and issues.
Comprehensive testing helps ensure that your React Native app functions as intended across various devices, screen sizes, and operating systems. It minimizes the risk of crashes, enhances user satisfaction, and maintains your app’s reputation. Let’s explore the different testing strategies you can employ.
2. Essential Testing Strategies
2.1 Unit Testing
Unit testing involves isolating individual components or functions of your app and testing them in isolation. In React Native, components are the building blocks of your app’s user interface. Unit tests for components ensure that they render correctly, handle user interactions properly, and exhibit the expected behavior.
Here’s a simple example of a unit test using Jest, a popular testing framework:
javascript // ButtonComponent.test.js import React from 'react'; import { render } from '@testing-library/react-native'; import ButtonComponent from '../ButtonComponent'; test('ButtonComponent renders correctly', () => { const { getByText } = render(<ButtonComponent label="Click me" />); const buttonElement = getByText('Click me'); expect(buttonElement).toBeTruthy(); });
2.2 Integration Testing
Integration testing goes a step further by testing how different components of your app work together. It ensures that your app’s components integrate seamlessly and produce the expected outcomes. This is particularly important in React Native, where the UI can be a composition of various components.
javascript // Integration test example // Suppose we're testing how a login form and authentication service work together import React from 'react'; import { render, fireEvent } from '@testing-library/react-native'; import LoginForm from '../LoginForm'; import AuthService from '../AuthService'; test('Login process works correctly', async () => { const { getByPlaceholderText, getByText } = render(<LoginForm />); const emailInput = getByPlaceholderText('Email'); const passwordInput = getByPlaceholderText('Password'); const loginButton = getByText('Login'); fireEvent.changeText(emailInput, 'test@example.com'); fireEvent.changeText(passwordInput, 'password123'); fireEvent.press(loginButton); // Assuming AuthService.login returns a success response const loginResponse = await AuthService.login('test@example.com', 'password123'); expect(loginResponse.success).toBeTruthy(); });
2.3 End-to-End Testing
End-to-end (E2E) testing simulates user interactions with your app in a real-world scenario. It ensures that the entire app workflow, from user input to the final result, functions as expected. E2E tests catch high-level issues and provide confidence in the overall functionality of your app.
Tools like Detox make E2E testing in React Native easier:
javascript // Detox E2E test example // Suppose we're testing the navigation flow between two screens import { device, element, by } from 'detox'; describe('Navigation Flow', () => { beforeEach(async () => { await device.reloadReactNative(); }); it('navigates from HomeScreen to DetailsScreen', async () => { await element(by.id('homeButton')).tap(); await expect(element(by.id('detailsScreen'))).toBeVisible(); }); });
3. Testing Tools for React Native Apps
3.1 Jest
Jest is a widely used JavaScript testing framework that simplifies writing unit tests, integration tests, and snapshot tests. It comes with built-in mocking capabilities and provides a smooth testing experience for React Native developers. Jest’s snapshot testing allows you to capture the current state of a component and compare it against future changes, helping you catch unexpected UI changes.
3.2 React Testing Library
React Testing Library is designed to encourage best practices for testing user interfaces. It focuses on testing how users interact with your components, ensuring that your tests closely resemble real-world scenarios. With its intuitive API, React Testing Library encourages developers to write tests that simulate user behavior, resulting in more reliable and maintainable test suites.
3.3 Detox
Detox is a powerful end-to-end testing framework specifically designed for React Native. It allows you to write E2E tests that interact with your app just like a real user would. Detox supports parallel test execution, device synchronization, and a straightforward API for writing robust E2E tests.
4. Best Practices for Effective Testing
4.1 Writing Testable Code
To facilitate effective testing, it’s essential to write code that is testable from the outset. This involves breaking down your app’s logic into modular components and functions. Following the principles of modularity and separation of concerns not only makes your codebase more manageable but also makes it easier to test individual parts of your app.
4.2 Automation
Automating your testing process helps catch issues early and reduces the chance of human error. Continuous Integration (CI) tools like Jenkins, CircleCI, or GitHub Actions can automatically run your test suite whenever you push code to your repository. This ensures that any new changes don’t introduce regressions or unexpected behavior.
4.3 Continuous Integration
Integrating testing into your CI/CD pipeline is crucial for maintaining code quality. By running tests automatically before deploying changes to production, you can catch potential problems before they impact users. This practice enhances collaboration among team members and helps maintain a stable codebase.
5. Monitoring and Debugging
Testing doesn’t end once your app is deployed. Monitoring and debugging are ongoing processes that help you identify and resolve issues in real-world scenarios. Utilize tools like Firebase Crashlytics or Sentry to monitor crashes and errors in your app. User feedback and analytics also provide valuable insights into how your app performs in the wild.
Conclusion
Testing React Native apps is an integral part of delivering a high-quality product. By employing unit testing, integration testing, and end-to-end testing, you can catch bugs early and ensure that your app functions as expected across different scenarios. Tools like Jest, React Testing Library, and Detox provide powerful testing capabilities that simplify the testing process.
Remember to follow best practices, write testable code, and integrate testing into your development workflow. Continuous testing and monitoring contribute to a stable app that provides an excellent user experience. In the ever-evolving landscape of app development, investing time and effort into testing will undoubtedly pay off in the long run, leading to satisfied users and a successful app.
Table of Contents
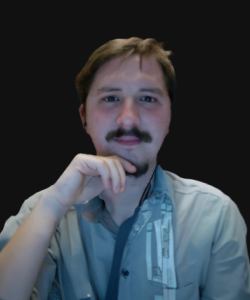
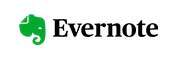