React Native and Firebase Analytics: Tracking App Usage and Metrics
In today’s highly competitive app market, building a great app is just the first step towards success. To stay ahead of the game, you need insights into how users are interacting with your app. This is where Firebase Analytics comes into play. In this guide, we’ll explore how to integrate Firebase Analytics into your React Native app to track usage and gather essential metrics.
Table of Contents
1. Why Firebase Analytics?
Firebase Analytics is a powerful tool offered by Google’s Firebase suite, designed to help app developers understand user behavior and engagement within their applications. It provides detailed insights into user interactions, allowing you to make data-driven decisions to improve your app’s performance and user experience.
Here are some key reasons why Firebase Analytics is a great choice for tracking app usage and metrics in your React Native app:
1.1. Real-time Data:
Firebase Analytics offers real-time tracking, allowing you to monitor user actions and events as they happen. This means you can quickly respond to changes in user behavior and optimize your app accordingly.
1.2. User Segmentation:
You can segment your users based on various criteria, such as demographics, location, and user properties. This helps you tailor your app’s content and features to specific user groups, increasing user engagement.
1.3. Event Tracking:
Firebase Analytics allows you to define custom events to track specific user interactions within your app. You can monitor events like button clicks, screen views, and in-app purchases, giving you a comprehensive view of user behavior.
1.4. Integration with Other Firebase Services:
Firebase Analytics seamlessly integrates with other Firebase services, such as Cloud Firestore and Cloud Functions. This enables you to trigger actions based on user behavior, making your app more dynamic and responsive.
1.5. Free and Scalable:
Firebase Analytics offers a free tier with generous usage limits, making it accessible to small and large app developers alike. Plus, it scales with your app’s growth, ensuring it remains a valuable tool as your user base expands.
Now that we understand the benefits of Firebase Analytics, let’s dive into the steps to integrate it into your React Native app.
2. Prerequisites
Before we get started, make sure you have the following prerequisites in place:
- React Native Project: You should have a React Native project up and running.
- Firebase Project: Create a Firebase project on the Firebase Console.
- Firebase SDK: Install the Firebase SDK in your React Native project. You can do this using npm or yarn.
bash # Using npm npm install --save @react-native-firebase/app # Using yarn yarn add @react-native-firebase/app
- Firebase Configuration: Retrieve your Firebase project’s configuration settings from the Firebase Console. These settings will be needed during the setup process.
With these prerequisites in place, let’s move on to integrating Firebase Analytics into your React Native app.
3. Integration Steps
3.1. Add Firebase to Your React Native Project
To begin, you’ll need to initialize Firebase in your React Native project. This is done by linking your Firebase project’s configuration settings.
First, create a file named firebase.js in the root directory of your project and add the Firebase configuration as follows:
javascript // firebase.js import { initializeApp } from '@firebase/app'; const firebaseConfig = { apiKey: 'YOUR_API_KEY', authDomain: 'YOUR_AUTH_DOMAIN', projectId: 'YOUR_PROJECT_ID', storageBucket: 'YOUR_STORAGE_BUCKET', messagingSenderId: 'YOUR_MESSAGING_SENDER_ID', appId: 'YOUR_APP_ID', }; const app = initializeApp(firebaseConfig); export default app;
Replace the placeholder values with the actual configuration settings from your Firebase project.
3.2. Install Additional Firebase Modules
To use Firebase Analytics, you’ll need to install the @react-native-firebase/analytics module. Run the following command to install it:
bash # Using npm npm install --save @react-native-firebase/analytics # Using yarn yarn add @react-native-firebase/analytics
3.3. Initialize Firebase Analytics
Now that you’ve installed the Firebase Analytics module, you need to initialize it in your app. Open your app’s entry file (usually index.js or App.js) and add the following code at the top:
javascript import { AppRegistry } from 'react-native'; import { name as appName } from './app.json'; import { App } from './App'; // Import your app component import analytics from '@react-native-firebase/analytics'; // Initialize Firebase Analytics analytics().setAnalyticsCollectionEnabled(true); // Register your app AppRegistry.registerComponent(appName, () => App);
This code sets up Firebase Analytics and enables data collection.
3.4. Tracking Events
With Firebase Analytics initialized, you can now start tracking events in your React Native app. Events can be anything from button clicks to screen views, and you can define custom events to track specific user interactions.
Here’s an example of tracking a custom event when a user clicks a “Sign Up” button:
javascript import { Button } from 'react-native'; import analytics from '@react-native-firebase/analytics'; // Inside your component const handleSignUpButtonClick = () => { // Track the "Sign Up" button click event analytics().logEvent('sign_up_clicked'); }; // Render your button <Button title="Sign Up" onPress={handleSignUpButtonClick} />
In this example, we use the logEvent method to track the “sign_up_clicked” event when the user clicks the “Sign Up” button. You can define your own events based on your app’s functionality.
3.5. User Properties
Firebase Analytics allows you to set user properties to segment your user base further. For instance, you can set a user property to differentiate between free and premium users or track user preferences.
Here’s an example of setting a user property to track user types:
javascript import analytics from '@react-native-firebase/analytics'; // Set user property for user type analytics().setUserProperty('user_type', 'premium');
In this example, we set the “user_type” property to “premium” for a user. You can customize user properties to match your app’s requirements.
2. Viewing Analytics Data
Once you’ve integrated Firebase Analytics and started tracking events and user properties, you can view the data on the Firebase Console. Here’s how:
- Go to the Firebase Console.
- Select your project.
- In the left sidebar, click on “Analytics.”
- You’ll find a wealth of information, including user engagement, event tracking, and user properties, to help you make informed decisions about improving your React Native app.
Conclusion
Firebase Analytics is an invaluable tool for React Native developers looking to gain insights into their app’s usage and user behavior. By tracking events and user properties, you can make data-driven decisions to enhance your app’s performance and user experience, ultimately driving app growth and success.
In this guide, we covered the basics of integrating Firebase Analytics into your React Native app, from setting up Firebase to tracking events and user properties. With this knowledge, you can start harnessing the power of Firebase Analytics to supercharge your app’s analytics capabilities.
Don’t miss out on the opportunity to understand your users better and make your app the best it can be. Start integrating Firebase Analytics into your React Native app today!
Table of Contents
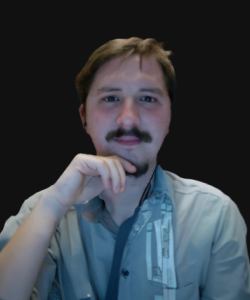
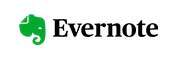