Building Video Recording in React Native Apps
Are you looking to integrate video recording functionality into your React Native app? Whether you’re creating a social media platform, a video-sharing app, or a personalized video messaging service, incorporating video recording capabilities can significantly enhance user engagement and interaction. In this guide, we’ll walk you through the process of building video recording functionality in React Native apps, covering everything from setting up the necessary dependencies to implementing the recording feature using the Expo Camera API.
Setting Up Your Development Environment
Before diving into the implementation, make sure you have Node.js and npm installed on your system. Additionally, you’ll need to have React Native CLI or Expo CLI installed, depending on your preference for app development. If you’re new to React Native, Expo provides a great starting point with its simplified development workflow and access to various APIs, including the Camera API, which we’ll be utilizing for video recording.
Installing Dependencies
To get started, create a new React Native project using the following command:
npx react-native init VideoRecordingApp
Next, navigate to your project directory and install the Expo Camera package:
expo install expo-camera
The Expo Camera package provides a simple interface for accessing the device’s camera and capturing photos and videos.
Implementing Video Recording
Now that we have our project set up and the necessary dependencies installed, let’s implement the video recording feature. We’ll create a simple screen with a record button that starts and stops video recording when tapped.
import React, { useState, useRef } from 'react'; import { View, TouchableOpacity, Text } from 'react-native'; import { Camera } from 'expo-camera'; const VideoRecordingScreen = () => { const [isRecording, setIsRecording] = useState(false); const cameraRef = useRef(null); const toggleRecording = async () => { if (isRecording) { cameraRef.current.stopRecording(); setIsRecording(false); } else { const { uri } = await cameraRef.current.recordAsync(); console.log('Video recorded at:', uri); setIsRecording(true); } }; return ( <View style={{ flex: 1 }}> <Camera style={{ flex: 1 }} type={Camera.Constants.Type.back} ref={cameraRef} /> <TouchableOpacity onPress={toggleRecording} style={{ alignSelf: 'center' }}> <Text style={{ fontSize: 20, marginBottom: 20 }}> {isRecording ? 'Stop Recording' : 'Start Recording'} </Text> </TouchableOpacity> </View> ); }; export default VideoRecordingScreen;
In this code snippet, we create a VideoRecordingScreen component that renders a camera preview using the Expo Camera component. We use state to track whether recording is currently in progress and a ref to access the camera instance. The toggleRecording function starts or stops video recording based on the current recording state.
Testing Your Implementation
Now that we’ve implemented the video recording feature, it’s time to test it on a device or emulator. Run your React Native project using the following command:
npx react-native run-android
or
npx react-native run-ios
Make sure to grant the app permission to access the device’s camera when prompted.
Conclusion
In this guide, we’ve covered the process of building video recording functionality in React Native apps using the Expo Camera API. By following these steps, you can seamlessly integrate video recording capabilities into your app, opening up a world of possibilities for user engagement and interaction.
External Resources:
Table of Contents
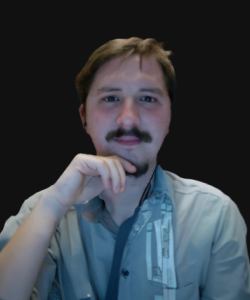
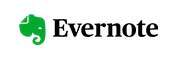