Building Voice Assistants with React Native and Dialogflow
In today’s fast-paced world, voice assistants have become an integral part of our lives. They help us with tasks ranging from setting alarms to answering questions and controlling smart devices. Building your own voice assistant can be a rewarding and educational experience, and with the combination of React Native and Dialogflow, it’s easier than you might think. In this comprehensive guide, we’ll walk you through the process of creating your own voice assistant using these powerful tools.
Table of Contents
1. Why Build a Voice Assistant with React Native and Dialogflow?
Before we dive into the technical details, let’s understand why React Native and Dialogflow are an excellent choice for building voice assistants.
1.1. Cross-Platform Compatibility
React Native is a popular framework for building cross-platform mobile applications. This means you can create voice assistants that work seamlessly on both Android and iOS devices, saving you time and effort in development.
1.2. Natural Language Processing
Dialogflow, powered by Google Cloud, is a robust natural language processing (NLP) platform. It can understand and respond to user queries in a conversational manner, making it perfect for voice assistant applications.
1.3. Scalability and Customization
Dialogflow allows you to train your voice assistant with specific intents and contexts, making it highly customizable. You can adapt it to various use cases, from simple task automation to complex interactions.
1.4. Google’s Speech Recognition
Dialogflow integrates seamlessly with Google’s speech recognition technology, providing accurate voice input processing.
Now that you understand why React Native and Dialogflow are a winning combination let’s get started with building your voice assistant.
2. Prerequisites
Before we dive into coding, ensure you have the following prerequisites:
2.1. Node.js and npm
Make sure you have Node.js and npm installed on your system. You can download them from the official website.
2.2. React Native CLI
Install the React Native CLI by running the following command:
bash npm install -g react-native-cli
2.3. Google Cloud Account
You’ll need a Google Cloud account to use Dialogflow. Sign up for one if you haven’t already.
2.4. Dialogflow Account
Create a Dialogflow account and set up a new project. This is where we’ll configure our voice assistant.
2.5. React Native Project
Set up a new React Native project using the following command:
bash react-native init VoiceAssistant
Once you have these prerequisites in place, we can start building our voice assistant.
3. Creating a React Native Voice Assistant
Step 1: Setting Up the Project
Navigate to your project directory and open it in your code editor. Let’s start by installing the necessary dependencies:
bash cd VoiceAssistant npm install @react-native-voice/voice npm install @react-native-community/viewpager npm install @react-navigation/native npm install @react-navigation/stack npm install react-native-dialogflow
These packages include essential components for our voice assistant project.
Step 2: Setting Up React Navigation
We’ll use React Navigation to manage the navigation flow within our app. Open the App.js file and set up the navigation structure as follows:
jsx import React from 'react'; import { NavigationContainer } from '@react-navigation/native'; import { createStackNavigator } from '@react-navigation/stack'; import HomeScreen from './screens/HomeScreen'; import VoiceAssistantScreen from './screens/VoiceAssistantScreen'; const Stack = createStackNavigator(); function App() { return ( <NavigationContainer> <Stack.Navigator initialRouteName="Home"> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="VoiceAssistant" component={VoiceAssistantScreen} /> </Stack.Navigator> </NavigationContainer> ); } export default App;
In this code, we’ve set up two screens: HomeScreen and VoiceAssistantScreen. The VoiceAssistantScreen is where we’ll integrate our voice assistant functionality.
Step 3: Creating the Home Screen
Let’s create the HomeScreen component, which will serve as the entry point to our voice assistant. This screen will have a button that the user can tap to start voice interaction.
jsx import React from 'react'; import { View, Text, Button, StyleSheet } from 'react-native'; const HomeScreen = ({ navigation }) => { return ( <View style={styles.container}> <Text style={styles.title}>Voice Assistant</Text> <Button title="Start Voice Assistant" onPress={() => navigation.navigate('VoiceAssistant')} /> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, title: { fontSize: 24, fontWeight: 'bold', }, }); export default HomeScreen;
Step 4: Setting Up Dialogflow
Now, let’s configure Dialogflow to understand and respond to voice commands. First, create a new agent in your Dialogflow project, and then define intents for various actions you want your voice assistant to perform.
For example, you can create intents like:
- “Turn on the lights”
- “Set an alarm”
- “Tell me a joke”
Dialogflow provides a user-friendly interface to create and manage intents. For each intent, you’ll define training phrases and responses.
Step 5: Integrating Dialogflow with React Native
To integrate Dialogflow with React Native, we’ll use the react-native-dialogflow library. Create a new file named VoiceAssistantScreen.js and add the following code:
jsx import React, { useState } from 'react'; import { View, Text, Button, StyleSheet } from 'react-native'; import Voice from '@react-native-voice/voice'; import Dialogflow from 'react-native-dialogflow'; const VoiceAssistantScreen = () => { const [isListening, setIsListening] = useState(false); const [response, setResponse] = useState(''); const startListening = async () => { try { setIsListening(true); setResponse(''); // Initialize Dialogflow with your credentials await Dialogflow.setConfiguration( 'YOUR_DIALOGFLOW_CLIENT_ACCESS_TOKEN', Dialogflow.LANG_ENGLISH_US ); // Start listening for voice input await Voice.start('en-US'); } catch (error) { console.error('Error starting voice recognition: ', error); } }; const stopListening = async () => { try { setIsListening(false); // Stop voice recognition await Voice.stop(); // Process the user's voice input with Dialogflow const result = await Dialogflow.startListening(); setResponse(result.result.fulfillment.speech); } catch (error) { console.error('Error stopping voice recognition: ', error); } }; return ( <View style={styles.container}> <Text style={styles.title}>Voice Assistant</Text> <Button title={isListening ? 'Listening...' : 'Start Listening'} onPress={isListening ? stopListening : startListening} disabled={isListening} /> {response !== '' && ( <Text style={styles.response}>{response}</Text> )} </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, title: { fontSize: 24, fontWeight: 'bold', }, response: { marginTop: 20, fontSize: 16, }, }); export default VoiceAssistantScreen;
In this code, we import the necessary libraries and set up the VoiceAssistantScreen component. This component allows users to start and stop voice recognition. When a user stops speaking, the voice input is sent to Dialogflow for processing, and the assistant’s response is displayed on the screen.
Step 6: Testing Your Voice Assistant
You can now run your React Native application using the following command:
bash react-native run-android
or
bash react-native run-ios
Make sure to replace ‘YOUR_DIALOGFLOW_CLIENT_ACCESS_TOKEN’ with your actual Dialogflow client access token.
With the app running on your device or emulator, tap the “Start Voice Assistant” button on the home screen. Speak a command, and your voice assistant should respond accordingly based on the defined intents in Dialogflow.
4. Enhancements and Customization
Building a basic voice assistant is just the beginning. You can enhance and customize your voice assistant in various ways:
4.1. Adding More Intents
Extend your Dialogflow agent by defining additional intents to handle a wider range of commands and queries.
4.2. Voice Feedback
Implement voice feedback so that your assistant can respond with spoken words rather than just text.
4.3. Integration with Smart Devices
Integrate your voice assistant with smart devices like lights, thermostats, and locks to control your home automation.
4.4. Error Handling
Implement robust error handling to gracefully handle cases where the assistant doesn’t understand or encounters errors.
4.5. Multi-Language Support
Expand your voice assistant’s language support by configuring additional languages in Dialogflow.
Conclusion
In this tutorial, we’ve explored how to build a voice assistant using React Native and Dialogflow. You’ve learned how to set up the project, configure Dialogflow, and integrate voice recognition to create a functional voice assistant. With further enhancements and customization, you can create a powerful and personalized voice assistant tailored to your needs.
Voice assistants have the potential to make our lives more convenient and efficient. By mastering this technology, you can not only build your own voice assistant but also contribute to the exciting field of natural language processing and human-computer interaction. So go ahead and start building your voice-powered future today!
Building Voice Assistants with React Native and Dialogflow can open up endless possibilities for creating personalized and powerful voice-driven applications. By following this guide, you’ll have the foundation to create your own voice assistant and the potential to make it even more capable with additional features and integrations. So, what are you waiting for? Start building your voice assistant today and make your app stand out in the world of mobile applications.
Table of Contents
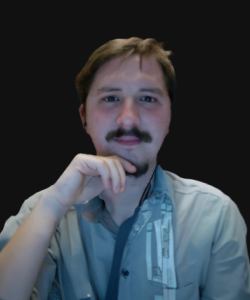
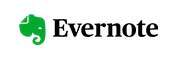