ReactJS and Augmented Reality: Building WebAR Applications
Augmented Reality (AR) has become a transformative technology, enhancing user experiences by overlaying digital information on the real world. Integrating AR with ReactJS can unlock powerful, interactive web applications. This blog will guide you through the essentials of building WebAR applications using ReactJS, including key libraries and code examples to get you started.
What is WebAR?
WebAR allows users to experience AR through web browsers without needing a dedicated app. It uses technologies like WebXR and WebGL to provide immersive experiences directly on websites. Combining WebAR with ReactJS lets you leverage React’s component-based architecture to build engaging, interactive AR applications efficiently.
Getting Started with ReactJS and WebAR
Setting Up Your Project
To build a WebAR application with ReactJS, you’ll first need to set up your development environment. Start by creating a new React project using Create React App.
```bash npx create-react-app my-webar-app cd my-webar-app ```
Next, install the necessary dependencies. For WebAR, you can use aframe (a web framework for building virtual reality experiences) along with react-aframe to integrate AR components into your React application.
```bash npm install aframe react-aframe ```
Integrating A-Frame for AR
A-Frame is a powerful library for building VR and AR experiences on the web. Here’s a basic
```jsx import React from 'react'; import 'aframe'; import { AFrameRenderer, Marker, MarkerCtrl } from 'react-aframe'; const App = () => ( <AFrameRenderer> <Marker> <a-marker preset="hiro"> <a-box position="0 0.5 0" rotation="0 45 0" color="#4CC3D9"></a-box> </a-marker> </Marker> </AFrameRenderer> ); export default App; ```
In this example, an A-Frame <a-box> element is positioned in AR space, and a Hiro marker is used to trigger its appearance.
Advanced WebAR Features
Adding 3D Models
To enhance your WebAR application, you might want to integrate 3D models. You can load models in formats like GLTF or OBJ using A-Frame’s <a-asset-item>.
```jsx <a-assets> <a-asset-item id="my-model" src="path/to/model.gltf"></a-asset-item> </a-assets> <a-entity gltf-model="#my-model" position="0 1 -3" scale="1 1 1" rotation="0 45 0" ></a-entity> ```
Interactivity and Animations
WebAR applications can benefit from interactive elements and animations. Use A-Frame’s components and React hooks to add dynamic behavior.
```jsx import { useState } from 'react'; const InteractiveBox = () => { const [color, setColor] = useState('#4CC3D9'); return ( <a-box position="0 0.5 -3" rotation="0 45 0" color={color} event-set__mouseenter="_event: mouseenter; color: #FFC65D" event-set__mouseleave="_event: mouseleave; color: #4CC3D9" onClick={() => setColor('#FF0000')} ></a-box> ); }; const App = () => ( <AFrameRenderer> <InteractiveBox /> </AFrameRenderer> ); ```
In this example, the box changes color when hovered over and toggles color on click.
Testing and Optimization
Testing WebAR applications can be challenging. Use various devices and browsers to ensure compatibility. Optimize performance by minimizing the use of complex 3D models and ensuring efficient asset loading.
Conclusion
Building WebAR applications with ReactJS opens up exciting possibilities for creating interactive, immersive web experiences. By leveraging libraries like A-Frame and integrating AR components, you can develop engaging applications that work seamlessly across different platforms.
Further Reading
Table of Contents
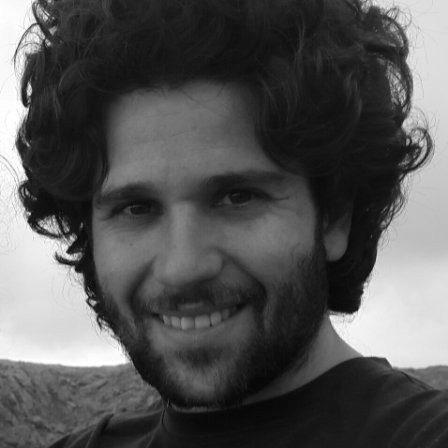
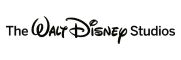