ReactJS and D3.js: Creating Interactive Data Visualizations
In the realm of web development, creating interactive and dynamic data visualizations has become essential for delivering engaging user experiences. ReactJS, a popular JavaScript library for building user interfaces, and D3.js, a powerful library for producing dynamic, interactive data visualizations in web browsers, are two tools that can be seamlessly integrated to build sophisticated visualizations. This blog will guide you through combining ReactJS and D3.js to create interactive data visualizations, highlighting key concepts and providing practical examples.
Understanding ReactJS and D3.js
What is ReactJS?
ReactJS, developed by Facebook, is a library for building user interfaces with a focus on component-based architecture. It allows developers to create reusable UI components that can efficiently update and render in response to data changes.
What is D3.js?
D3.js (Data-Driven Documents) is a JavaScript library for producing dynamic, interactive data visualizations using HTML, SVG, and CSS. It provides powerful tools for data manipulation and visual representation, enabling the creation of complex visualizations with a high degree of customization.
Integrating ReactJS with D3.js
Combining ReactJS with D3.js might initially seem challenging due to their different approaches to updating the DOM. React handles updates through its virtual DOM and state management, while D3.js directly manipulates the DOM. However, with the right approach, you can leverage the strengths of both libraries.
Setting Up Your Project
To get started, you need to set up a React project. You can use Create React App to quickly bootstrap a new project:
```bash npx create-react-app my-data-visualization cd my-data-visualization npm install d3 ```
Creating a Basic Component
Let’s create a simple React component that uses D3.js to render a bar chart. First, create a new file named `BarChart.js` in the `src` directory:
```javascript // src/BarChart.js import React, { useRef, useEffect } from 'react'; import * as d3 from 'd3'; const BarChart = ({ data }) => { const svgRef = useRef(); useEffect(() => { const svg = d3.select(svgRef.current) .attr('width', 500) .attr('height', 500); svg.selectAll('*').remove(); // Clear previous elements svg.selectAll('rect') .data(data) .enter() .append('rect') .attr('x', (d, i) => i * 50) .attr('y', d => 500 - d) .attr('width', 40) .attr('height', d => d) .attr('fill', 'steelblue'); }, [data]); return <svg ref={svgRef}></svg>; }; export default BarChart; ```
Using the Component
You can now use the `BarChart` component in your `App.js` file:
```javascript // src/App.js import React, { useState } from 'react'; import BarChart from './BarChart'; const App = () => { const [data, setData] = useState([100, 200, 300, 400, 500]); return ( <div> <h1>Interactive Data Visualization</h1> <BarChart data={data} /> <button onClick={() => setData(data.map(d => d + 50))}> Update Data </button> </div> ); }; export default App; ```
In this example, the `BarChart` component renders a bar chart using D3.js. The `useEffect` hook ensures that the chart updates when the `data` prop changes. The button in `App.js` demonstrates how to interact with the chart by updating the data.
Advanced Techniques
For more complex visualizations, consider incorporating D3.js features like transitions, scales, and axes. You might also use React’s context and hooks for state management and interactivity.
Further Reading
Table of Contents
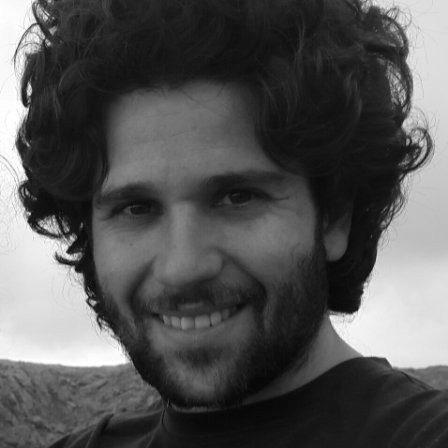
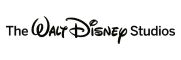