ReactJS and Docker: Containerizing Your Application for Deployment
In modern web development, deploying applications efficiently and consistently is crucial. Combining ReactJS, a powerful library for building user interfaces, with Docker, a robust platform for containerization, can streamline the deployment process and ensure that your application runs smoothly across various environments. This blog will guide you through containerizing a ReactJS application using Docker, covering the essential steps and providing practical code samples.
Why Use Docker for ReactJS Applications?
Docker simplifies the deployment process by encapsulating your application and its dependencies into a container. This approach ensures that your ReactJS application will run the same way regardless of the environment, whether it’s your local machine, a staging server, or a production server.
Benefits of using Docker with ReactJS include:
– Consistency: Docker containers provide a consistent environment for your application, eliminating the “it works on my machine” problem.
– Scalability: Containers can be easily scaled horizontally, making it simple to handle increased traffic.
– Isolation: Each container runs in its own isolated environment, reducing conflicts with other applications.
Setting Up Your ReactJS Application
Before diving into Docker, you need a ReactJS application. If you don’t have one yet, you can quickly set up a new project using Create React App.
```bash npx create-react-app my-react-app cd my-react-app ```
This command creates a new ReactJS project in a directory named `my-react-app`.
Creating a Dockerfile
A Dockerfile is a script that contains a series of instructions to build a Docker image. For a ReactJS application, you need to create a Dockerfile in the root of your project.
Here’s a basic Dockerfile for a ReactJS application:
```Dockerfile # Use the official Node.js image as the base image FROM node:18 AS build # Set the working directory in the container WORKDIR /app # Copy package.json and package-lock.json COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application code COPY . . # Build the ReactJS application RUN npm run build # Use a smaller image to serve the built application FROM nginx:alpine # Copy the build output from the previous stage to the Nginx container COPY --from=build /app/build /usr/share/nginx/html # Expose port 80 to be able to access the application EXPOSE 80 # Command to run Nginx in the foreground CMD ["nginx", "-g", "daemon off;"] ```
Building the Docker Image
With your Dockerfile in place, you can now build the Docker image. Run the following command in your terminal:
```bash docker build -t my-react-app . ```
This command builds the Docker image and tags it as `my-react-app`.
Running the Docker Container
Once the image is built, you can run it as a container:
```bash docker run -p 80:80 my-react-app ```
This command maps port 80 of the container to port 80 of your host machine, allowing you to access your ReactJS application via `http://localhost`.
Testing and Debugging
To test your container, open a web browser and navigate to `http://localhost`. You should see your ReactJS application running. If you encounter any issues, use Docker’s logs and inspection commands to debug:
```bash docker logs <container_id> docker inspect <container_id> ```
Conclusion
Containerizing your ReactJS application with Docker provides numerous benefits, including consistency, scalability, and isolation. By following the steps outlined in this blog, you can create a Dockerfile, build a Docker image, and run your application in a containerized environment. This approach simplifies deployment and ensures that your application runs seamlessly across different environments.
Further Reading
Table of Contents
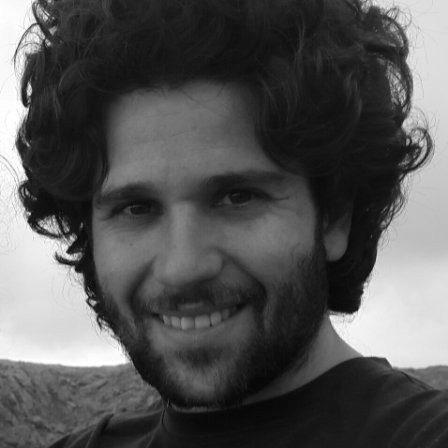
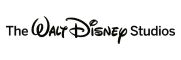