Building E-commerce Applications with ReactJS and Stripe
Building a robust e-commerce application involves multiple layers, from product management to handling payments. Integrating Stripe with ReactJS can streamline the payment process and enhance the user experience. In this guide, we’ll walk through the essentials of setting up an e-commerce application using ReactJS and Stripe, covering both the frontend and backend components.
Setting Up Your ReactJS Application
Before diving into Stripe integration, you’ll need a ReactJS application. If you haven’t already created one, you can start with Create React App:
```bash npx create-react-app ecommerce-app cd ecommerce-app npm start ```
This will set up a basic ReactJS application for you to build upon.
Installing Stripe Dependencies
To integrate Stripe, you’ll need both Stripe’s JavaScript library and its React components. Install them using npm:
```bash npm install @stripe/stripe-js @stripe/react-stripe-js ```
Setting Up Stripe in Your React Application
Creating a Stripe Context
Create a new file, `StripeProvider.js`, in your `src` folder to manage Stripe context and load the Stripe library:
```javascript // src/StripeProvider.js import React from 'react'; import { Elements } from '@stripe/react-stripe-js'; import { loadStripe } from '@stripe/stripe-js'; const stripePromise = loadStripe('your-publishable-key-here'); const StripeProvider = ({ children }) => ( <Elements stripe={stripePromise}> {children} </Elements> ); export default StripeProvider; ```
Creating a Checkout Form
Next, create a `CheckoutForm.js` component where users will enter their payment details:
```javascript // src/CheckoutForm.js import React, { useState } from 'react'; import { CardElement, useStripe, useElements } from '@stripe/react-stripe-js'; const CheckoutForm = () => { const stripe = useStripe(); const elements = useElements(); const [error, setError] = useState(null); const [success, setSuccess] = useState(null); const handleSubmit = async (event) => { event.preventDefault(); if (!stripe || !elements) return; const { error, paymentMethod } = await stripe.createPaymentMethod({ type: 'card', card: elements.getElement(CardElement), }); if (error) { setError(error.message); setSuccess(null); } else { setSuccess('Payment Successful!'); setError(null); console.log(paymentMethod); // Call your backend to handle payment } }; return ( <form onSubmit={handleSubmit}> <CardElement /> <button type="submit" disabled={!stripe}>Pay</button> {error && <div>{error}</div>} {success && <div>{success}</div>} </form> ); }; export default CheckoutForm; ```
Integrating StripeProvider and CheckoutForm
Wrap your main application component with `StripeProvider` and include `CheckoutForm`:
```javascript // src/App.js import React from 'react'; import StripeProvider from './StripeProvider'; import CheckoutForm from './CheckoutForm'; const App = () => ( <StripeProvider> <div> <h1>ReactJS E-commerce App</h1> <CheckoutForm /> </div> </StripeProvider> ); export default App; ```
Handling Payments on the Backend
You’ll need a backend server to handle payment processing securely. Here’s a simple Express server example:
```javascript // server.js const express = require('express'); const stripe = require('stripe')('your-secret-key-here'); const app = express(); app.use(express.json()); app.post('/create-payment-intent', async (req, res) => { const { amount } = req.body; const paymentIntent = await stripe.paymentIntents.create({ amount, currency: 'usd', }); res.json({ clientSecret: paymentIntent.client_secret }); }); app.listen(4000, () => console.log('Server is running on port 4000')); ```
Connecting Frontend to Backend
Update the `handleSubmit` function in `CheckoutForm.js` to call your backend:
```javascript const handleSubmit = async (event) => { event.preventDefault(); if (!stripe || !elements) return; const { error: backendError, clientSecret } = await fetch('/create-payment-intent', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ amount: 1000 }), // Example amount }).then((res) => res.json()); if (backendError) { setError(backendError.message); setSuccess(null); return; } const { error, paymentIntent } = await stripe.confirmCardPayment(clientSecret, { payment_method: { card: elements.getElement(CardElement), }, }); if (error) { setError(error.message); setSuccess(null); } else if (paymentIntent.status === 'succeeded') { setSuccess('Payment Successful!'); setError(null); } }; ```
Conclusion
Integrating Stripe with ReactJS provides a streamlined way to handle payments in your e-commerce application. By setting up Stripe on both the frontend and backend, you can ensure a secure and user-friendly checkout experience. Experiment with additional features such as saving payment methods or handling subscriptions to further enhance your app.
Further Reading
Table of Contents
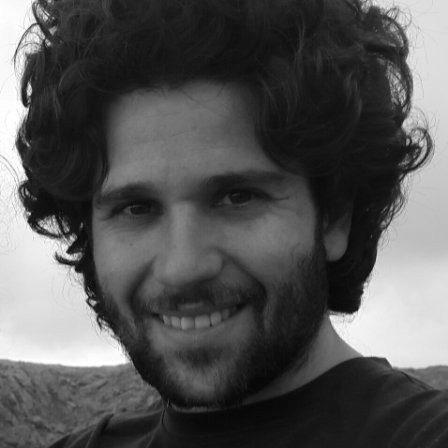
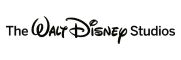