ReactJS and Electron: Building Cross-Platform Desktop Applications
In the world of application development, creating cross-platform applications has become increasingly important. ReactJS and Electron offer a powerful combination for building desktop applications that work seamlessly across different operating systems. ReactJS provides a robust framework for building interactive user interfaces, while Electron allows you to package these applications as native desktop apps. This blog explores how to leverage both technologies to create cross-platform desktop applications.
Understanding ReactJS and Electron
What is ReactJS?
ReactJS is a popular JavaScript library developed by Facebook for building user interfaces, particularly single-page applications where responsiveness and user experience are crucial. It allows developers to create reusable UI components, which can be efficiently updated and rendered.
What is Electron?
Electron is an open-source framework developed by GitHub that enables developers to build cross-platform desktop applications using web technologies like HTML, CSS, and JavaScript. It combines Chromium (the engine behind Google Chrome) and Node.js, allowing for the creation of desktop apps with web development tools.
Setting Up a ReactJS and Electron Project
Prerequisites
Before diving into the code, make sure you have Node.js and npm (Node Package Manager) installed on your machine. You can download and install them from [Node.js official website](https://nodejs.org/).
Step 1: Create a New ReactJS Project
First, create a new ReactJS project using Create React App:
```bash npx create-react-app my-electron-app cd my-electron-app ```
This will set up a basic ReactJS project in a directory named `my-electron-app`.
Step 2: Install Electron
Next, install Electron as a development dependency:
```bash npm install --save-dev electron ```
Step 3: Configure Electron
Create a new file named `main.js` in the root directory of your project. This file will serve as the entry point for Electron.
```javascript const { app, BrowserWindow } = require('electron'); const path = require('path'); function createWindow () { const mainWindow = new BrowserWindow({ width: 800, height: 600, webPreferences: { preload: path.join(__dirname, 'preload.js'), nodeIntegration: true, contextIsolation: false } }); mainWindow.loadURL('http://localhost:3000'); } app.whenReady().then(() => { createWindow(); app.on('activate', () => { if (BrowserWindow.getAllWindows().length === 0) { createWindow(); } }); }); app.on('window-all-closed', () => { if (process.platform !== 'darwin') { app.quit(); } }); ```
This script initializes an Electron application and opens a window that loads the React app.
Step 4: Update `package.json`
Modify the `scripts` section in `package.json` to include a start script for Electron:
```json "scripts": { "start": "react-scripts start", "electron-start": "electron ." } ```
This setup allows you to start your React app and Electron simultaneously. Run the following command to start both:
```bash npm run start npm run electron-start ```
Building and Packaging the Application
Once your application is ready, you need to package it for distribution. Electron offers a package called `electron-builder` for this purpose. Install it using:
```bash npm install --save-dev electron-builder ```
Add a `build` script to your `package.json`:
```json "scripts": { "build": "react-scripts build", "electron-build": "electron-builder" } ```
To build your application, run:
```bash npm run build npm run electron-build ```
This will create a packaged version of your application that you can distribute.
Conclusion
ReactJS and Electron together offer a powerful solution for building cross-platform desktop applications. ReactJS’s component-based architecture and Electron’s ability to package web apps as native applications make this combination a popular choice among developers. By following the steps outlined in this blog, you can start creating your own desktop applications with ease.
Further Reading
Table of Contents
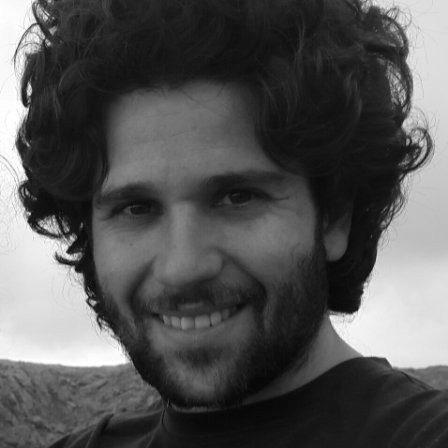
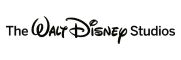