ReactJS and Firebase: Building Serverless Web Applications
In the modern web development landscape, building scalable and performant applications often means navigating the complex world of backend infrastructure. However, the advent of serverless architectures has simplified this process significantly. Combining ReactJS, a powerful front-end library, with Firebase, a comprehensive serverless backend platform, allows developers to create robust web applications with ease. This blog explores how to leverage these technologies to build serverless web applications effectively.
Why Choose ReactJS and Firebase?
ReactJS: A Dynamic Front-End Library
ReactJS, developed by Facebook, is a popular JavaScript library for building user interfaces. Its component-based architecture allows developers to create reusable UI components, making it easier to manage and scale applications. React’s declarative approach and virtual DOM enhance performance, leading to a smooth user experience.
Firebase: A Serverless Backend Solution
Firebase, a platform developed by Google, offers a range of cloud-based services designed to simplify backend development. Key features include:
– Firestore: A flexible, scalable NoSQL cloud database.
– Authentication: Easy-to-use authentication solutions.
– Hosting: Fast and secure web hosting.
– Functions: Serverless functions for custom backend logic.
Combining Firebase with ReactJS allows developers to focus on creating rich user experiences without managing traditional server infrastructure.
Setting Up Your Development Environment
To get started, you’ll need to set up your development environment for ReactJS and Firebase.
Installing ReactJS
First, create a new ReactJS project using Create React App:
```bash npx create-react-app my-app cd my-app ```
Adding Firebase to Your Project
Install Firebase SDK:
```bash npm install firebase ```
Integrating Firebase with ReactJS
To connect Firebase with your ReactJS app, follow these steps:
Configuring Firebase
Create a Firebase project in the Firebase Console. Add a new web app to obtain your Firebase configuration object.
Adding Firebase Configuration
In your React project, create a new file `firebase.js` inside the `src` directory:
```javascript // src/firebase.js import firebase from 'firebase/app'; import 'firebase/firestore'; import 'firebase/auth'; // Your web app's Firebase configuration const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_PROJECT_ID.firebaseapp.com", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_PROJECT_ID.appspot.com", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" }; // Initialize Firebase firebase.initializeApp(firebaseConfig); const firestore = firebase.firestore(); const auth = firebase.auth(); export { firestore, auth }; ```
Using Firebase in React Components
You can now use Firebase services in your React components. Here’s an example of how to use Firestore to display a list of items:
```javascript // src/App.js import React, { useState, useEffect } from 'react'; import { firestore } from './firebase'; function App() { const [items, setItems] = useState([]); useEffect(() => { const unsubscribe = firestore.collection('items').onSnapshot(snapshot => { const itemsData = snapshot.docs.map(doc => ({ id: doc.id, ...doc.data() })); setItems(itemsData); }); return () => unsubscribe(); }, []); return ( <div> <h1>Item List</h1> <ul> {items.map(item => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); } export default App; ```
Authentication with Firebase
Adding authentication to your ReactJS application is straightforward with Firebase. You can use Firebase Authentication to handle user sign-ins and sign-ups.
Setting Up Authentication
Update your `firebase.js` file to include authentication:
```javascript import 'firebase/auth'; // Initialize Firebase Authentication const auth = firebase.auth(); export { auth }; ```
Adding Authentication to Components
Here’s an example of a simple sign-in form:
```javascript // src/SignIn.js import React, { useState } from 'react'; import { auth } from './firebase'; function SignIn() { const [email, setEmail] = useState(''); const [password, setPassword] = useState(''); const handleSignIn = async () => { try { await auth.signInWithEmailAndPassword(email, password); // Redirect or update UI } catch (error) { console.error('Error signing in', error); } }; return ( <div> <h2>Sign In</h2> <input type="email" value={email} onChange={(e) => setEmail(e.target.value)} placeholder="Email" /> <input type="password" value={password} onChange={(e) => setPassword(e.target.value)} placeholder="Password" /> <button onClick={handleSignIn}>Sign In</button> </div> ); } export default SignIn; ```
Conclusion
Combining ReactJS with Firebase enables you to build serverless web applications efficiently. ReactJS handles the frontend, offering a dynamic and responsive user experience, while Firebase provides a scalable backend solution with minimal management. By leveraging these technologies, developers can focus more on creating engaging features rather than dealing with infrastructure complexities.
Further Reading
Table of Contents
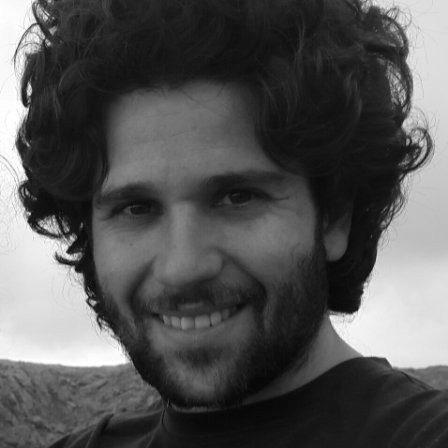
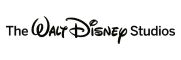