Optimizing ReactJS Functions for Performance: A Comprehensive Guide to useCallback
ReactJS is a popular JavaScript library used for building user interfaces. As your React applications grow in complexity, optimizing performance becomes crucial. One way to achieve this is by using the useCallback hook, which helps optimize functions to prevent unnecessary re-renders. In this blog post, we will explore the useCallback hook in ReactJS and learn how to leverage its power to boost the performance of your applications.
1. Understanding useCallback
The useCallback hook is a built-in React hook that allows you to memoize functions, ensuring that they are not recreated on every render unless their dependencies change. By memoizing functions, React can skip unnecessary re-renders and improve the overall performance of your application.
The useCallback hook takes two arguments: the callback function to memoize and an array of dependencies. The dependencies are used to determine whether the callback function needs to be recreated or not.
Code Example 1: Basic usage of useCallback
import React, { useCallback } from 'react'; const MyComponent = () => { const handleClick = useCallback(() => { console.log('Button clicked'); }, []); return ( <button onClick={handleClick}>Click Me</button> ); };
In the code above, we define a functional component called MyComponent. Inside the component, we declare a callback function handleClick using the useCallback hook. Since we pass an empty dependency array [], the function is memoized and won’t be recreated on subsequent renders.
2. Preventing Unnecessary Re-renders
One of the key benefits of using useCallback is preventing unnecessary re-renders. When a component re-renders, all functions defined within the component are recreated. This can be a problem when passing functions as props to child components, as they may receive new function references on each render.
By memoizing the functions with useCallback and providing the necessary dependencies, we can ensure that the function references remain the same unless the dependencies change.
Code Example 2: useCallback with dependencies
import React, { useState, useCallback } from 'react'; const ParentComponent = () => { const [count, setCount] = useState(0); const increment = useCallback(() => { setCount(count + 1); }, [count]); return ( <div> <ChildComponent onIncrement={increment} /> </div> ); }; const ChildComponent = ({ onIncrement }) => { return ( <button onClick={onIncrement}>Increment</button> ); };
In the code snippet above, we have a ParentComponent that maintains a count state. We define the increment function using useCallback and include the count variable in the dependency array. This ensures that the increment function remains the same as long as the count state doesn’t change.
The increment function is then passed as a prop to the ChildComponent. Since the function reference remains the same unless count changes, the ChildComponent doesn’t need to re-render unnecessarily when ParentComponent re-renders.
3. Optimizing Complex and Expensive Functions
Sometimes, your callback functions might be computationally expensive or depend on large data sets. In such cases, you can leverage useCallback to optimize the performance by ensuring that the function is only recalculated when necessary.
Code Example 3: useCallback with expensive computation
import React, { useState, useCallback } from 'react'; const MyComponent = () => { const [data, setData] = useState([]); const processData = useCallback(() => { // Expensive computation on the data array // ... }, [data]); // Update the // Update the data array and trigger the processData function const updateData = () => { // ... setData(newData); }; return ( <div> <button onClick={updateData}>Update Data</button> </div> ); };
In the code example above, we have a MyComponent that maintains a data state, representing a large data set. We define the processData function using useCallback and include the data variable in the dependency array.
The processData function performs an expensive computation on the data array. By memoizing the function with useCallback, we ensure that the computation is only executed when the data array changes. This prevents unnecessary recalculations during component re-renders, thereby optimizing performance.
4. Additional Tips for Using useCallback
Use useCallback strategically: While useCallback can be beneficial for optimizing functions, it’s important to use it strategically. Not all functions need to be memoized with useCallback, especially if they don’t have any dependencies. Evaluate the specific use case and consider the potential performance gains before applying useCallback.
Avoid unnecessary dependencies: When defining the dependencies array for useCallback, be mindful of including only the necessary variables or state values. Including unnecessary dependencies can lead to excessive recalculations and diminish the performance benefits.
Extract expensive computations or heavy logic: If your callback function includes computationally expensive operations or complex logic, consider extracting them into separate functions. By memoizing these functions with useCallback, you can optimize their performance individually and improve overall code readability.
Combine useCallback with other optimization techniques: useCallback can be used in combination with other React optimization techniques like memoization (using React.memo) and useMemo. These tools work together to ensure that both components and functions are memoized and re-rendered only when needed.
Profile and test performance: As with any optimization technique, it’s essential to profile and test the performance of your application before and after implementing useCallback. Tools like React Developer Tools and browser performance profilers can help you analyze the impact of your optimizations and make informed decisions.
By following these tips and considering the specific needs of your React application, you can harness the power of useCallback to improve performance and provide a smooth user experience.
5. Advanced Usage: Dynamic Callback Dependencies
So far, we have seen examples where the dependencies array of useCallback contains static values or state variables. However, there might be scenarios where you need to dynamically generate the dependencies based on the function’s logic. In such cases, you can use useCallback in conjunction with other hooks, such as useEffect, to achieve dynamic dependencies.
Code Example 4: Dynamic dependencies with useCallback and useEffect
import React, { useState, useEffect, useCallback } from 'react'; const DynamicDependenciesComponent = () => { const [data, setData] = useState([]); const [filter, setFilter] = useState(''); useEffect(() => { // Fetch data from API // ... setData(apiData); }, []); const filteredData = data.filter(item => item.includes(filter)); const handleFilterChange = useCallback((event) => { setFilter(event.target.value); }, []); return ( <div> <input type="text" value={filter} onChange={handleFilterChange} /> <ul> {filteredData.map(item => <li key={item}>{item}</li>)} </ul> </div> ); };
In the code example above, we have a component that fetches data from an API and stores it in the data state. The filter state represents a filter value for the data. We use the useEffect hook to fetch the data and populate the data state initially.
The handleFilterChange function, defined with useCallback, updates the filter state whenever the input value changes. However, the dependencies array for useCallback is empty, indicating that the function doesn’t have any direct dependencies.
The function’s dependencies are determined dynamically based on its logic. In this case, since the function relies on the filter state, it will automatically include filter as a dependency.
By utilizing the dynamic nature of dependencies, you can ensure that the function is memoized correctly and only recreated when its dependent state changes.
6. Common Pitfalls and Considerations
While useCallback can greatly optimize function performance, there are a few pitfalls and considerations to keep in mind:
Overusing useCallback: While useCallback can be beneficial, using it excessively can lead to over-optimization and reduced code readability. Evaluate the specific functions that truly benefit from memoization and apply useCallback judiciously.
Incorrect dependencies: It’s crucial to provide the correct dependencies in the array passed to useCallback. Missing or incorrect dependencies can lead to stale data or unnecessary re-renders. Carefully analyze the dependencies and ensure they accurately reflect the variables or state values that the function relies on.
Avoid unnecessary nesting: Nesting components with useCallback can lead to unnecessary re-memoization of functions. If a child component doesn’t depend on the callback function, it’s better to define the function outside the component and pass it as a prop.
Performance trade-offs: While useCallback improves performance by reducing re-renders, it’s important to balance the trade-off between performance gains and memory usage. Memoized functions remain in memory, so excessively memoizing functions that aren’t frequently used can lead to increased memory consumption.
Testing memoized functions: Memoized functions can introduce challenges when writing unit tests. Since the function references remain the same, you may need to simulate changes in the dependencies to ensure appropriate test coverage.
Evaluating performance gains: Before and after applying useCallback, it’s essential to profile and measure the performance of your application. Benchmarking and analyzing the impact of useCallback can help determine if the optimization is significant enough to justify the added complexity.
Let’s dive into a code example that demonstrates the use of useCallback in a more complex scenario.
Code Example 5: useCallback with a complex computation
import React, { useState, useCallback } from 'react'; const ComplexComputationComponent = () => { const [data, setData] = useState([]); const [result, setResult] = useState(null); const processData = useCallback(() => { // Complex computation using the data array const computedResult = data.reduce((accumulator, item) => accumulator + item, 0); setResult(computedResult); }, [data]); const handleDataChange = useCallback((event) => { const newData = event.target.value.split(',').map(Number); setData(newData); }, []); return ( <div> <input type="text" onChange={handleDataChange} /> <button onClick={processData}>Process Data</button> {result && <p>Result: {result}</p>} </div> ); };
In the code example above, we have a component called ComplexComputationComponent that allows the user to input a comma-separated list of numbers. When the “Process Data” button is clicked, the processData function performs a complex computation on the data array and updates the result state with the computed result.
The processData function is memoized using useCallback with the data dependency. This ensures that the function is only recreated if the data array changes, preventing unnecessary recalculations on subsequent renders.
The handleDataChange function, also defined with useCallback, updates the data state whenever the input value changes. This allows for efficient handling of input changes without recreating the function unnecessarily.
By using useCallback in this scenario, we optimize the performance by preventing redundant computations when the data remains unchanged.
Let’s explore another code example that showcases the usage of useCallback with dependent functions.
Code Example 6: useCallback with dependent functions
import React, { useState, useCallback } from 'react'; const DependentFunctionsComponent = () => { const [count, setCount] = useState(0); const increment = useCallback(() => { setCount((prevCount) => prevCount + 1); }, []); const decrement = useCallback(() => { setCount((prevCount) => prevCount - 1); }, []); return ( <div> <button onClick={increment}>Increment</button> <button onClick={decrement}>Decrement</button> <p>Count: {count}</p> </div> ); };
In this code example, we have a component called DependentFunctionsComponent that maintains a count state. It renders two buttons, one for incrementing and another for decrementing the count value. The increment and decrement functions are memoized using useCallback.
The increment function increases the count value by 1 when invoked, while the decrement function decreases the count value by 1. Both functions rely on the setCount function provided by the useState hook.
By using useCallback, we ensure that the increment and decrement functions remain the same between renders unless the dependencies change. This avoids unnecessary re-rendering of the buttons and improves the overall performance of the component.
Code Example 7: useCallback with event handlers
import React, { useState, useCallback } from 'react'; const EventHandlersComponent = () => { const [inputValue, setInputValue] = useState(''); const [displayText, setDisplayText] = useState(''); const handleChange = useCallback((event) => { setInputValue(event.target.value); }, []); const handleSubmit = useCallback((event) => { event.preventDefault(); setDisplayText(inputValue); }, [inputValue]); return ( <div> <form onSubmit={handleSubmit}> <input type="text" value={inputValue} onChange={handleChange} /> <button type="submit">Submit</button> </form> {displayText && <p>Display Text: {displayText}</p>} </div> ); };
In this code example, we have a component called EventHandlersComponent that includes an input field and a submit button. The handleChange function is memoized using useCallback and updates the inputValue state with the current input value.
The handleSubmit function, also memoized with useCallback, prevents the default form submission behavior, sets the displayText state with the current input value, and displays it below the form.
By memoizing the event handlers using useCallback, we ensure that they are only recreated if their dependencies (inputValue in this case) change. This optimization prevents unnecessary re-renders of the component and improves performance.
Code Example 8: useCallback with callbacks passed to child components
import React, { useState, useCallback } from 'react'; const ParentComponent = () => { const [count, setCount] = useState(0); const handleIncrement = useCallback(() => { setCount((prevCount) => prevCount + 1); }, []); const handleDecrement = useCallback(() => { setCount((prevCount) => prevCount - 1); }, []); return ( <div> <ChildComponent onIncrement={handleIncrement} onDecrement={handleDecrement} /> <p>Count: {count}</p> </div> ); }; const ChildComponent = ({ onIncrement, onDecrement }) => { return ( <div> <button onClick={onIncrement}>Increment</button> <button onClick={onDecrement}>Decrement</button> </div> ); };
In this code example, we have a ParentComponent that maintains a count state. It renders a ChildComponent and passes the handleIncrement and handleDecrement functions as props.
Both handleIncrement and handleDecrement are memoized using useCallback. This ensures that the function references remain the same unless the dependencies change, preventing unnecessary re-renders of the ChildComponent.
The ChildComponent receives the onIncrement and onDecrement props and attaches them to the corresponding buttons’ onClick handlers.
By using useCallback to memoize the callback functions passed to child components, we optimize the performance by avoiding unnecessary re-renders of the child component when the parent component updates.
Code Example 9: useCallback with dependency arrays
import React, { useState, useCallback } from 'react'; const DependencyArraysComponent = () => { const [count, setCount] = useState(0); const [text, setText] = useState(''); const handleIncrement = useCallback(() => { setCount(count + 1); }, [count]); const handleInputChange = useCallback((event) => { setText(event.target.value); }, []); return ( <div> <button onClick={handleIncrement}>Increment</button> <input type="text" value={text} onChange={handleInputChange} /> <p>Count: {count}</p> <p>Text: {text}</p> </div> ); };
In this code example, we have a component called DependencyArraysComponent that maintains a count state and a text state. It renders a button for incrementing the count, an input field for entering text, and displays the current count and text values.
The handleIncrement function is memoized using useCallback and has a dependency array of [count]. This means that the function will only be recreated if the count state changes.
Similarly, the handleInputChange function is memoized using useCallback and has an empty dependency array []. This ensures that the function is created only once and does not depend on any specific state value.
By providing dependency arrays to useCallback, we optimize the creation of the callback functions and ensure that they are only recreated when necessary.
Using useCallback with External APIs or Libraries:
Another scenario where useCallback can be useful is when working with external APIs or libraries that rely on callback functions. By memoizing these callback functions, we can ensure their stability and prevent unnecessary re-renders.
Code Example 10: useCallback with external library callback
import React, { useState, useCallback } from 'react'; import { ExternalLibrary } from 'external-library'; const ExternalLibraryComponent = () => { const [data, setData] = useState([]); const fetchData = useCallback(() => { ExternalLibrary.fetchData((response) => { setData(response.data); }); }, []); return ( <div> <button onClick={fetchData}>Fetch Data</button> <ul> {data.map((item) => ( <li key={item.id}>{item.name}</li> ))} </ul> </div> ); };
In this example, we have a fetchData function that uses an external library to fetch data asynchronously. The callback function inside the library’s method updates the state with the fetched data.
By memoizing the fetchData function using useCallback, we ensure that the callback function passed to the external library remains the same between renders unless the dependencies change. This prevents unnecessary re-renders and ensures stability when working with the library.
Using useCallback with Event Listeners:
Event listeners are another common use case for useCallback. By memoizing event handlers, we optimize their performance and prevent reattaching them on each render.
Code Example 11: useCallback with event listeners
import React, { useState, useCallback, useEffect } from 'react'; const EventListenerComponent = () => { const [scrollPosition, setScrollPosition] = useState(0); const handleScroll = useCallback(() => { setScrollPosition(window.scrollY); }, []); useEffect(() => { window.addEventListener('scroll', handleScroll); return () => { window.removeEventListener('scroll', handleScroll); }; }, [handleScroll]); return ( <div> <p>Scroll Position: {scrollPosition}px</p> </div> ); };
In this example, we have a component that tracks the scroll position of the window. The handleScroll function is memoized using useCallback to optimize its performance.
Inside the useEffect hook, we add the event listener for the ‘scroll’ event and attach the memoized handleScroll function. The cleanup function removes the event listener when the component unmounts.
By using useCallback for the event handler and providing it as a dependency in the useEffect hook, we ensure that the event listener is attached and detached correctly, without causing unnecessary re-renders.
Conclusion
In this blog post, we have explored various scenarios where useCallback can be applied to optimize functions for performance in ReactJS applications. We’ve seen examples of optimizing event handlers, working with external APIs or libraries, and using useCallback with event listeners.
Remember that useCallback is a powerful tool for preventing unnecessary re-renders and optimizing function performance. However, it should be used judiciously and applied only to functions that truly benefit from memoization.
By strategically implementing useCallback in your ReactJS applications, you can improve performance, enhance the user experience, and create efficient and responsive web applications.
Happy coding and optimizing your ReactJS applications!
Table of Contents
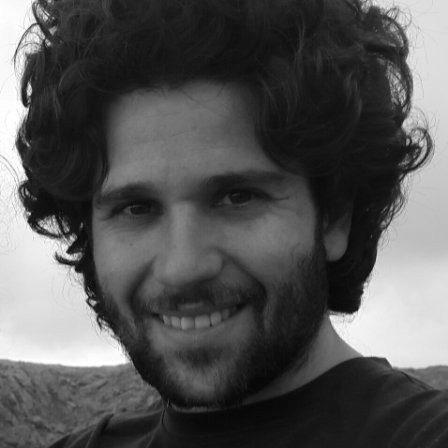
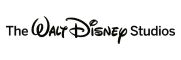