ReactJS and Micro-Frontends: A Guide to Building Modular Applications
In the evolving world of web development, building modular applications is becoming increasingly important for scalability and maintainability. One approach gaining traction is the use of ReactJS in conjunction with micro-frontends. This guide will delve into how ReactJS and micro-frontends can be combined to create robust and modular applications.
What Are Micro-Frontends?
Micro-frontends extend the microservices pattern to the frontend. The idea is to break down a frontend application into smaller, independently deployable pieces, each owned by a separate team. This modular approach allows for better scalability, faster development cycles, and improved maintainability.
Why Use ReactJS for Micro-Frontends?
ReactJS is an excellent choice for building micro-frontends due to its component-based architecture. With React, you can encapsulate functionality within reusable components, making it easier to manage and scale. React’s component model aligns well with the principles of micro-frontends, which emphasize modularity and separation of concerns.
Setting Up a ReactJS Micro-Frontend Architecture
To get started with ReactJS and micro-frontends, you need to follow a few key steps:
1. Create Independent React Applications:
Each micro-frontend should be a separate React application. This isolation ensures that each part of your frontend can be developed, tested, and deployed independently.
```bash npx create-react-app microfrontend1 npx create-react-app microfrontend2 ```
2. Use a Container or Host Application:
This application will act as the shell that hosts the micro-frontends. It’s responsible for rendering and orchestrating the different micro-frontends.
```bash npx create-react-app container-app ```
3. Integrate Micro-Frontends:
One common way to integrate micro-frontends is by using Webpack Module Federation, which allows multiple builds to be consumed by a host application.
– Micro-Frontend Configuration:
```javascript // webpack.config.js for microfrontend1 module.exports = { plugins: [ new ModuleFederationPlugin({ name: 'microfrontend1', filename: 'remoteEntry.js', exposes: { './MicroFrontend1': './src/MicroFrontend1', }, }), ], }; ``` - **Host Application Configuration:** ```javascript // webpack.config.js for container-app module.exports = { plugins: [ new ModuleFederationPlugin({ remotes: { microfrontend1: 'microfrontend1@http://localhost:3001/remoteEntry.js', }, }), ], }; ```
4. Load Micro-Frontends Dynamically:
Use React’s dynamic imports to load the micro-frontends as needed.
```javascript // App.js in container-app import React, { Suspense, lazy } from 'react'; const MicroFrontend1 = lazy(() => import('microfrontend1/MicroFrontend1')); function App() { return ( <div className="App"> <Suspense fallback={<div>Loading...</div>}> <MicroFrontend1 /> </Suspense> </div> ); } export default App; ```
Best Practices for Building Micro-Frontends
1. Decouple Micro-Frontends:
Ensure that micro-frontends are loosely coupled. They should interact through well-defined APIs rather than direct communication.
2. Use Consistent Styling:
Maintain a consistent look and feel across micro-frontends by using a shared design system or CSS framework.
3. Optimize Performance:
Implement strategies to minimize the impact on performance, such as lazy loading and efficient asset management.
4. Monitor and Maintain:
Regularly monitor and maintain micro-frontends to ensure they work well together and adhere to best practices.
Conclusion
Combining ReactJS with micro-frontends allows developers to build scalable, modular applications that are easier to manage and evolve. By following the steps outlined above and adhering to best practices, you can leverage these technologies to create robust applications that meet modern development needs.
Further Reading
Table of Contents
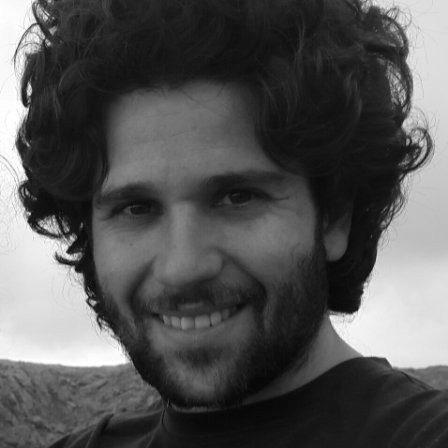
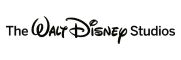