How to perform prop validation?
In React, components communicate and share data primarily through props. As applications grow in complexity, it becomes vital to ensure that the props passed to a component meet specific criteria or types. This is where prop validation comes into play.
Prop validation in React provides a way to specify the type and structure of the data that a component should receive. By validating props, developers can prevent bugs, ensure more predictable behaviors, and provide clear documentation for component usage. When a prop fails validation, React logs a clear warning in the console, aiding in faster debugging.
Historically, React included built-in prop type validation through the `propTypes` property. With `propTypes`, developers can specify the type of data each prop should contain, whether it’s a string, number, function, or even more complex data structures. They can also mark certain props as required, ensuring that a component receives all necessary data to function correctly. For example, using `React.PropTypes.string` or `React.PropTypes.number.isRequired` would indicate the expected type of a prop and its necessity.
However, as the JavaScript ecosystem evolved and tools like TypeScript and Flow gained prominence, some developers began leveraging these statically-typed languages for more robust type checking. Both TypeScript and Flow integrate well with React and offer more in-depth type validation mechanisms, going beyond the capabilities of `propTypes`.
Prop validation is an essential aspect of developing maintainable and bug-free React applications. Whether utilizing React’s built-in `propTypes` or opting for more advanced solutions like TypeScript, validating props ensures that components receive the right data, fostering more predictable component behaviors and streamlining the debugging process.
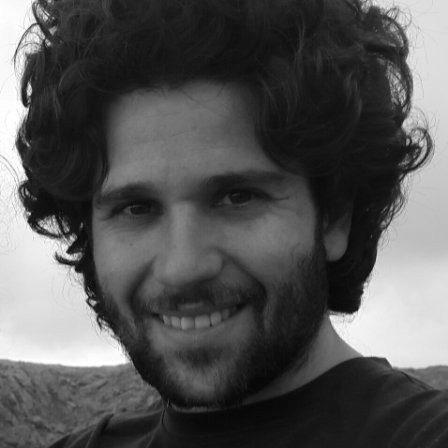
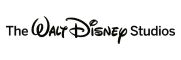