Building Responsive Web Applications with ReactJS and Material-UI
Building responsive web applications is essential in today’s multi-device world. With a wide array of screen sizes and resolutions, developers must create interfaces that adapt gracefully. ReactJS, a powerful front-end library, combined with Material-UI, a popular UI framework, offers an efficient and flexible solution for developing responsive web applications. In this blog, we’ll explore the key concepts and techniques for building responsive web apps using these technologies.
Introduction to Responsive Design
Responsive design ensures that a web application provides an optimal viewing experience across various devices, from desktops to smartphones. The goal is to create a seamless user experience, regardless of screen size. This involves fluid grids, flexible images, and CSS media queries.
Why Choose ReactJS and Material-UI?
ReactJS simplifies the process of building interactive user interfaces. Its component-based architecture allows developers to create reusable components, making the development process more efficient. Material-UI, a set of React components that implement Google’s Material Design, provides a comprehensive suite of pre-built components and styles, helping developers build consistent and attractive UIs quickly.
Setting Up the Environment
To start building a responsive web application with ReactJS and Material-UI, you need to set up your development environment. Follow these steps:
Create a React App: Use Create React App (CRA) to set up a new React project.
```bash npx create-react-app my-responsive-app cd my-responsive-app ```
Install Material-UI: Add Material-UI to your project.
```bash npm install @mui/material @emotion/react @emotion/styled ```
2.
Add Material-UI Icons: If you need icons, you can also install the Material-UI icons
package. ```bash npm install @mui/icons-material ```
3.
Implementing Responsive Components
Using Grid Layout
Material-UI provides a powerful grid system that is responsive by default. The Grid component allows you to create layouts that adapt to different screen sizes.
```jsx import React from 'react'; import { Grid, Typography } from '@mui/material'; function ResponsiveGrid() { return ( <Grid container spacing={2}> <Grid item xs={12} sm={6} md={4}> <Typography variant="h6">Item 1</Typography> </Grid> <Grid item xs={12} sm={6} md={4}> <Typography variant="h6">Item 2</Typography> </Grid> <Grid item xs={12} sm={6} md={4}> <Typography variant="h6">Item 3</Typography> </Grid> </Grid> ); } export default ResponsiveGrid; ```
In this example, the Grid component creates a responsive layout with three items. The xs, sm, and md props define the number of grid columns each item should span at different breakpoints.
Responsive Typography and Styling
Material-UI’s Typography component and theming capabilities make it easy to implement responsive typography. You can define different font sizes for various screen sizes using the useTheme hook.
```jsx import React from 'react'; import { Typography, useTheme } from '@mui/material'; import { useMediaQuery } from '@mui/material'; function ResponsiveTypography() { const theme = useTheme(); const isSmallScreen = useMediaQuery(theme.breakpoints.down('sm')); return ( <Typography variant={isSmallScreen ? 'h5' : 'h3'}> Responsive Typography </Typography> ); } export default ResponsiveTypography; ```
In this code, useMediaQuery is used to detect if the screen size is smaller than the “sm” breakpoint, adjusting the typography accordingly.
Conclusion
Building responsive web applications with ReactJS and Material-UI offers a streamlined and efficient approach. With their powerful features and components, you can easily create adaptive and visually appealing UIs. By leveraging the grid system, responsive typography, and theming, developers can ensure a seamless experience across all devices.
Further Reading
Table of Contents
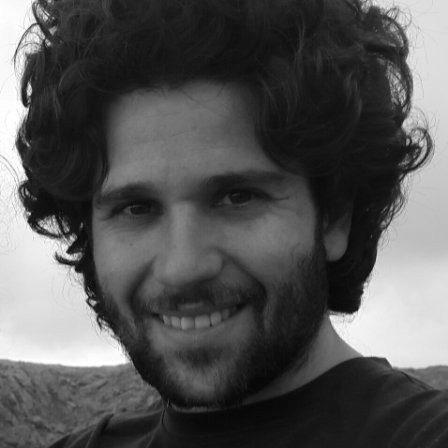
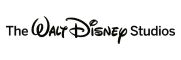