Sound Design for User Delight: Crafting Custom Audio Player Hooks with ReactJS’s useAudio
ReactJS is a library used to build user interfaces, primarily for single-page applications. It offers a great way to build reusable UI components, handle state, and create clean, easily testable code. However, like any tool, it doesn’t handle everything out of the box. For more specific tasks like audio playback, you may need to build custom hooks to add the functionality you need.
In this post, we’ll delve into the creation of a custom ReactJS Hook for audio playback, known as `useAudio`. By the end of this article, you should be comfortable building and using your custom hook in your own applications.
Introduction to React Hooks
Introduced in React 16.8, Hooks are functions that allow you to “hook into” React state and lifecycle features from functional components. This advancement meant that we no longer needed to convert a functional component into a class component to access state, lifecycle methods, or other React features.
Some built-in hooks provided by React include `useState`, `useEffect`, `useContext`, etc. But React also gives us the flexibility to create our own custom hooks when we need more specific functionality that isn’t covered by the built-in hooks.
Building the useAudio Hook
We’ll create a `useAudio` hook that handles audio playback. This hook will return an object that allows us to play, pause, stop, loop, and monitor the progress of the audio.
import { useState, useEffect } from 'react'; function useAudio(url) { const
= useState(new Audio(url)); const [playing, setPlaying] = useState(false); const [progress, setProgress] = useState(0); const [duration, setDuration] = useState(0); const toggle = () => setPlaying(!playing); useEffect(() => { playing ? audio.play() : audio.pause(); }, [playing]); useEffect(() => { audio.addEventListener('ended', () => setPlaying(false)); return () => { audio.removeEventListener('ended', () => setPlaying(false)); }; }, []); useEffect(() => { audio.addEventListener('loadedmetadata', () => { setDuration(audio.duration); }); audio.addEventListener('timeupdate', () => { setProgress(audio.currentTime); }); return () => { audio.removeEventListener('loadedmetadata', () => {}); audio.removeEventListener('timeupdate', () => {}); }; }, []); return { playing, progress, duration, toggle, }; }
The `useAudio` hook takes a URL as a parameter and sets up an `Audio` object with that URL. It has a `playing` state variable that keeps track of whether the audio is currently playing. The `toggle` function flips the `playing` state, starting or pausing the audio as necessary.
There are three `useEffect` hooks that handle different aspects of the audio. The first updates whenever `playing` changes and either plays or pauses the audio. The second adds an event listener for the ‘ended’ event, setting `playing` to false when the audio finishes. The third adds event listeners for the ‘loadedmetadata’ and ‘timeupdate’ events, which update the `duration` and `progress` of the audio respectively.
Using the useAudio Hook
You can use the `useAudio` hook in a functional component just like any other hook:
import useAudio from './hooks/useAudio'; function AudioPlayer({ url }) { const { playing, progress, duration, toggle } = useAudio(url); return ( <div> <button onClick={toggle}>{playing ? "Pause" : "Play"}</button> <progress value={progress} max={duration}></progress> </div> ); }
This `AudioPlayer` component uses the `useAudio` hook to control audio playback. It displays a button that either plays or pauses the audio when clicked, and a progress bar that shows the progress of the audio.
Conclusion
Hooks in React have revolutionized the way we manage state and lifecycle methods in functional components. By creating a custom hook like `useAudio`, we’re able to extend React’s built-in functionality and customize our applications even further.
This `useAudio` hook is a basic implementation that can be expanded according to your needs. For example, you could add functionality for seeking to different points in the audio, adjusting the volume, or handling multiple audio tracks.
React’s flexibility is one of its greatest strengths, and custom hooks are an excellent example of that. So whether you’re working with audio, video, animations, or any other aspect of your application, don’t be afraid to create your own hooks to tailor React to your needs.
Table of Contents
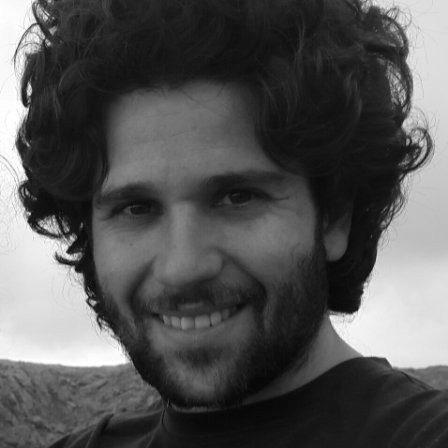
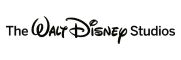