Mastering Clipboard Interactions: Building a Robust Clipboard Management Hook with ReactJS’s useCopyToClipboard
As we constantly evolve and refine our coding practices, the need for better, simpler, and more efficient ways of doing things continues to be a driving force. ReactJS, a robust and widely adopted JavaScript library for building user interfaces, offers an ecosystem filled with numerous hooks and components, facilitating better state and effect management. In this article, we will discuss how to build a clipboard management hook, which we will term `useCopyToClipboard`.
Why a Clipboard Management Hook?
When building a web application, you often encounter scenarios where you need to provide a feature for users to copy text to the clipboard. For instance, you may need a button that, when clicked, copies a URL, a code snippet, or any other text to the clipboard. Creating a reusable hook for this purpose not only keeps the code DRY (Don’t Repeat Yourself), but also promotes better code management and readability.
Prerequisites
Before we dive in, it’s essential that you have a basic understanding of React and how hooks work. Familiarity with the basics of JavaScript ES6 syntax, like arrow functions, destructuring, and promises, is also crucial.
Building the useCopyToClipboard Hook
First, let’s start by setting up a new React project, if you don’t already have one:
npx create-react-app clipboard-hook cd clipboard-hook
Once inside your project directory, let’s get started with our `useCopyToClipboard` hook:
import { useState, useEffect } from 'react'; const useCopyToClipboard = (text) => { const copyToClipboard = (str) => { const clipboard = window.navigator.clipboard; /* Modern browsers have navigator.clipboard API */ if(clipboard && window.isSecureContext) { return clipboard.writeText(str); } else { /* Fallback to older execCommand API for unsupported browsers */ let textArea = document.createElement('textarea'); textArea.value = str; textArea.style.position = "fixed"; //avoid scrolling to bottom document.body.appendChild(textArea); textArea.focus(); textArea.select(); try { document.execCommand('copy'); return Promise.resolve(true); }catch (err) { return Promise.reject(err); } finally { document.body.removeChild(textArea); } } } const [copied, setCopied] = useState(false); const copy = () => { if (!copied) setCopied(true); copyToClipboard(text); } useEffect(() = > { if (copied) { const timer = setTimeout(() => { setCopied(false); }, 2000); return () => clearTimeout(timer); } }, [copied]); return [copied, copy]; }; export default useCopyToClipboard;
Breaking Down the useCopyToClipboard Hook
Let’s dissect the `useCopyToClipboard` hook to understand how it works.
At its core, our `useCopyToClipboard` hook is a function that accepts a `text` parameter. We use the `navigator.clipboard.writeText()` method to copy this text into the clipboard. This method returns a promise that either resolves if the text is successfully copied or rejects if it fails.
We create a `copyToClipboard` function to handle this action and use feature detection to ensure that our function will work in both modern browsers (which support the Clipboard API) and older ones (which don’t). In the latter case, we create a temporary textarea, append it to the body, copy the text, and then remove the textarea.
Next, we define a `copied` state variable that helps us track whether the text has been successfully copied to the clipboard. We initialize this state as `false`.
Our `copy` function then updates this state to `true` if the text hasn’t already been copied, and calls the `copyToClipboard` function to perform the actual copy operation.
We use the `useEffect` hook to listen for changes to the `copied` state. If the state is `true`, it means that the text has been successfully copied to the clipboard, so we start a timer. After 2 seconds, we set the `copied` state back to `false`, indicating that the copy operation is complete. This delay could be used to show a feedback message to the user that the text has been copied.
Using the useCopyToClipboard Hook
You can now use the `useCopyToClipboard` hook in your components like this:
import React from 'react'; import useCopyToClipboard from './useCopyToClipboard'; function App() { const text = 'Hello, World!'; const [copied, copy] = useCopyToClipboard(text); return ( <div> <button onClick={copy}>Copy to clipboard</button> {copied && <span style={{color: 'green'}}>Copied to clipboard!</span>} </div> ); } export default App;
Here, we import our `useCopyToClipboard` hook and pass the text we want to copy. When the button is clicked, the `copy` function is called, copying the text to the clipboard and setting the `copied` state to `true`. We use this state to display a feedback message to the user.
Conclusion
Building custom hooks in React allows us to write cleaner, more readable and reusable code. The `useCopyToClipboard` hook we’ve built here is a handy tool that you can incorporate in your applications to facilitate text copying. However, it’s important to note that the Clipboard API might not be available or may require permission in some browsers, so always ensure to provide appropriate fallbacks for the best user experience.
Table of Contents
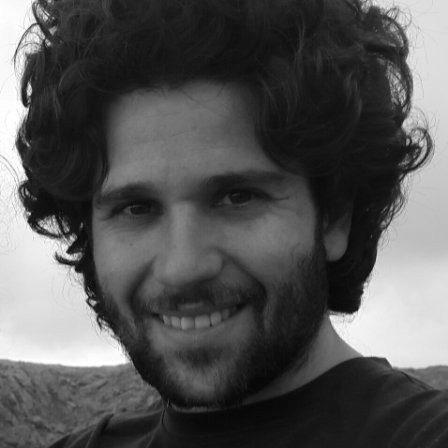
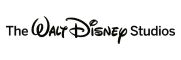