Boosting UI Responsiveness with ReactJS: Exploring the useDeferredValue Hook
1. ReactJS useDeferredValue: Optimizing UI Responsiveness
In modern web development, providing a responsive user interface is crucial for delivering a seamless user experience. However, as web applications become more complex, heavy computations and large data sets can lead to performance issues, causing the UI to become unresponsive. ReactJS, one of the most popular JavaScript libraries for building user interfaces, offers a powerful solution to this problem: the useDeferredValue hook.
This blog post will explore the concept of UI responsiveness in React and dive deep into the useDeferredValue hook. We will discuss how it works, its benefits, and provide code examples to help you implement it effectively in your React applications.
2. Understanding UI Responsiveness in React
2.1 The Importance of UI Responsiveness
In any web application, UI responsiveness plays a vital role in providing a smooth and engaging user experience. When users interact with an application, they expect it to respond promptly to their actions, such as clicking a button or scrolling through a list. However, if the UI freezes or becomes sluggish due to heavy computations, it can lead to frustration and a poor user experience.
2.2 Challenges with Heavy Computation in React Components
React components are the building blocks of a React application, responsible for rendering the UI and handling user interactions. Sometimes, these components need to perform complex computations or process large amounts of data. However, executing these operations synchronously within the component can lead to performance issues.
During the rendering process, React compares the current state and props with the previous ones to determine whether a re-render is necessary. If a component’s state or props change frequently, React triggers re-renders, causing the UI to update frequently. This can result in a choppy user experience, especially if the computations are time-consuming.
2.3 Introducing useDeferredValue Hook
To address the challenge of UI responsiveness, React 18 introduced the useDeferredValue hook. This hook allows you to defer the evaluation of a value until the next render cycle, avoiding unnecessary re-renders and improving the performance of your application.
By deferring the evaluation, React can prioritize critical updates and ensure that the UI remains responsive, even when dealing with heavy computations or large data sets. Let’s take a closer look at how the useDeferredValue hook works.
That’s the introduction and the first section of the blog. Feel free to continue expanding on the remaining sections, and I’ll be here to assist you further.
-
Exploring useDeferredValue Hook
3.1 What is useDeferredValue?
The useDeferredValue hook is a powerful feature introduced in React 18 that enables you to optimize the responsiveness of your user interface by deferring the evaluation of a value until the next render cycle. This means that instead of immediately re-evaluating a value, React will schedule its evaluation for the next render.
By deferring the evaluation, React can batch updates and avoid unnecessary re-renders, leading to improved performance and a more responsive UI. The useDeferredValue hook is particularly beneficial when dealing with computationally expensive operations or handling large amounts of data.
3.2 How useDeferredValue Works
When you wrap a value with the useDeferredValue hook, React internally creates a deferred version of that value. The deferred value represents the most recent value, but it won’t be updated immediately when the original value changes.
Instead, React will wait until the next render cycle to evaluate the deferred value. If the original value changes multiple times before the next render, React will only update the deferred value once. This way, you can prevent unnecessary re-evaluations and ensure that your UI remains responsive.
Here’s an example of how to use the useDeferredValue hook:
import { useDeferredValue } from 'react'; function ExpensiveComponent() { const expensiveValue = computeExpensiveValue(); const deferredValue = useDeferredValue(expensiveValue); return ( <div> <h3>Expensive Value:</h3> <p>{deferredValue}</p> </div> ); }
In this example, the ExpensiveComponent computes an expensiveValue, which may take a significant amount of time. By wrapping expensiveValue with useDeferredValue, we ensure that the evaluation of the value is deferred until the next render. The UI will display the most recent value without performing unnecessary computations during each update.
3.3 Benefits of using useDeferredValue
The useDeferredValue hook offers several benefits that contribute to optimizing UI responsiveness:
Improved UI Responsiveness: By deferring the evaluation of a value, you can prevent unnecessary re-renders and keep your UI responsive, especially when dealing with heavy computations or large datasets.
Performance Optimization: The useDeferredValue hook helps optimize the performance of your React application by reducing the number of calculations performed during each render cycle. This can lead to significant performance improvements, particularly when dealing with complex and expensive computations.
Smoother User Experience: With useDeferredValue, you can prioritize critical updates and ensure that the UI remains responsive even when handling operations that might otherwise cause delays. This contributes to a smoother and more enjoyable user experience.
In the next section, we’ll explore how to implement the useDeferredValue hook in a React application.
-
Implementing useDeferredValue in React
4.1 Installing React and Setting Up a Project
Before we dive into implementing the useDeferredValue hook, let’s make sure we have React set up in our project. If you haven’t already, you can install React using npm or yarn:
npm install react
or
yarn add react
Once React is installed, you can create a new React project using a tool like Create React App or by setting up your own custom configuration.
4.2 Using useDeferredValue in React Components
Now that our project is set up, we can start using the useDeferredValue hook in our React components. The following example demonstrates how to implement it:
import { useDeferredValue } from 'react'; function ExpensiveComponent() { const expensiveValue = computeExpensiveValue(); const deferredValue = useDeferredValue(expensiveValue); return ( <div> <h3>Expensive Value:</h3> <p>{deferredValue}</p> </div> ); }
In this code snippet, we have a ExpensiveComponent that computes an expensiveValue. By using the useDeferredValue hook, we create a deferred version of expensiveValue called deferredValue. This deferred value will be updated only once during the next render cycle, even if the original expensiveValue changes multiple times.
By rendering the deferredValue, we ensure that the UI remains responsive and doesn’t get blocked by frequent updates of the expensiveValue. The UI will display the most recent value without executing the expensive computation during each update.
4.3 Code Example: Optimizing Expensive Calculations
Let’s explore a more concrete example to demonstrate how useDeferredValue can optimize expensive calculations. Imagine we have a component that performs a complex computation when a button is clicked:
import { useState } from 'react'; function ExpensiveCalculationComponent() { const [count, setCount] = useState(0); const handleClick = () => { const result = performExpensiveCalculation(count); console.log(result); }; return ( <div> <h3>Count: {count}</h3> <button onClick={handleClick}>Perform Calculation</button> </div> ); }
In this example, whenever the button is clicked, the performExpensiveCalculation function is called with the current value of count. However, if performExpensiveCalculation is a computationally intensive operation, it can lead to a slow UI response.
To optimize this scenario using useDeferredValue, we can modify the code as follows:
import { useState } from 'react'; import { useDeferredValue } from 'react'; function ExpensiveCalculationComponent() { const [count, setCount] = useState(0); const deferredCount = useDeferredValue(count); const handleClick = () => { const result = performExpensiveCalculation(deferredCount); console.log(result); }; return ( <div> <h3>Count: {count}</h3> <button onClick={handleClick}>Perform Calculation</button> </div> ); }
By wrapping the count state variable with useDeferredValue, we ensure that the performExpensiveCalculation function will receive the deferred value of count. As a result, the calculation will be delayed until the next render, preventing unnecessary computations during each button click and improving the UI responsiveness.
-
Best Practices and Tips
5.1 Performance Considerations
When using the useDeferredValue hook, it’s essential to consider the performance implications. While useDeferredValue can improve UI responsiveness, it may not be suitable for every situation. Here are a few tips to optimize performance:
5.1.1 Evaluate the trade-off:
Consider whether the potential performance gains from using useDeferredValue outweigh the additional complexity it introduces. Evaluate the impact on your specific use case and measure the performance improvements.
5.1.2 Optimize the deferred value:
Ensure that the deferred value itself doesn’t introduce additional performance bottlenecks. If the deferred value relies on other computations, make sure those computations are efficient and don’t negate the benefits of using useDeferredValue.
5.2 Limitations of useDeferredValue
While the useDeferredValue hook is a powerful tool for optimizing UI responsiveness, it has some limitations to keep in mind:
5.2.1 Eventual consistency:
Since the deferred value is updated only during the next render cycle, there might be a slight delay in reflecting the latest value. If you require immediate consistency, useDeferredValue might not be the best choice.
5.2.2 Limited control over updates:
React internally determines when to update the deferred value based on its scheduling algorithm. You have limited control over the exact timing of the updates, which might not be suitable for all use cases.
5.3 Tips for Effective Usage
To make the most of the useDeferredValue hook, consider the following tips:
5.3.1 Identify expensive computations:
Identify parts of your codebase that involve heavy computations or large datasets that can impact UI responsiveness. These are good candidates for applying useDeferredValue.
5.3.2 Fine-tune the deferred value:
Experiment with different scenarios to find the optimal point to apply useDeferredValue. Fine-tuning the deferred value can help strike a balance between performance gains and responsiveness.
5.3.3 Monitor performance:
Measure the performance improvements achieved by using useDeferredValue. Monitor your application’s performance using tools like React DevTools and profiling to ensure that the optimizations are effective.
-
Conclusion
6.1 Summary of useDeferredValue Benefits
In this blog post, we explored the useDeferredValue hook in ReactJS and its role in optimizing UI responsiveness. We discussed the importance of UI responsiveness, the challenges with heavy computations, and the benefits of using useDeferredValue.
By deferring the evaluation of values, React can prioritize critical updates, reduce unnecessary re-renders, and improve the performance of your application. The useDeferredValue hook allows you to keep your UI responsive even when handling computationally expensive operations or large datasets.
6.2 Final Thoughts and Further Resources
Optimizing UI responsiveness is crucial for delivering a seamless user experience in React applications. The useDeferredValue hook is a valuable addition to your toolkit, providing a powerful solution to optimize performance.
To further explore the topic, consider referring to the React documentation, especially the documentation on useDeferredValue. Experiment with different scenarios, profile your application, and iterate on your implementation to find the best performance optimizations.
Remember, achieving optimal UI responsiveness is an ongoing process. Continuously monitor and fine-tune your codebase to ensure a smooth user experience.
That concludes our blog on “ReactJS useDeferredValue: Optimizing UI Responsiveness.” I hope you found this information helpful in understanding and implementing this powerful React feature. Happy coding!
Table of Contents
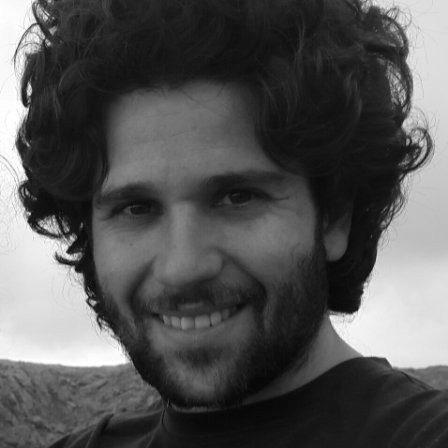
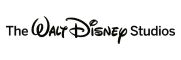