Voice to Victory: Building Intuitive Voice-Controlled Applications with ReactJS’s useSpeechRecognition Hook
In the evolving realm of web development, developers constantly seek tools that enhance user engagement and offer seamless interactions. Voice-controlled applications are one such advancement that’s rapidly gaining traction. This post will explore ReactJS useSpeechRecognition, an innovative library that lets developers easily build voice-controlled applications.
Introduction to useSpeechRecognition
The useSpeechRecognition is a handy hook provided by the React Speech Recognition library. This library allows developers to harness the Web Speech API’s SpeechRecognition interface, letting users interact with the application through their voice.
While the SpeechRecognition API has numerous functionalities, React Speech Recognition simplifies its usage, making it more accessible for React developers.
Getting Started
To get started, first, install the library in your React application with the npm package manager:
npm install --save react-speech-recognition
Or, using yarn:
yarn add react-speech-recognition
Ensure your project runs on a server that supports HTTPS since the browser’s speech recognition API only works with secure contexts.
Basic Usage of useSpeechRecognition
Once you’ve successfully installed the package, import `useSpeechRecognition` from ‘react-speech-recognition’ in your component file:
import { useSpeechRecognition } from 'react-speech-recognition';
Now, let’s create a simple voice-controlled component to understand the use of `useSpeechRecognition`:
import React from 'react'; import { useSpeechRecognition } from 'react-speech-recognition'; const Dictaphone = () => { const { transcript, resetTranscript } = useSpeechRecognition(); if (!SpeechRecognition.browserSupportsSpeechRecognition()) { return null; } return ( <div> <button onClick={SpeechRecognition.startListening}>Start</button> <button onClick={SpeechRecognition.stopListening}>Stop</button> <button onClick={resetTranscript}>Reset</button> <p>{transcript}</p> </div> ); }; export default Dictaphone;
In the above component, `startListening` initiates the voice recognition, `stopListening` halts it, and `resetTranscript` clears the recognized transcript. The `transcript` variable contains the recognized text.
Command-Based Voice Control
Building a fully voice-controlled application usually involves more than just transcribing user voice into text. It often requires the interpretation of specific spoken commands. The React Speech Recognition library provides the `commands` prop to handle this:
import React from 'react'; import { useSpeechRecognition } from 'react-speech-recognition'; const CommandControlledComponent = () => { const commands = [ { command: 'go back', callback: () => window.history.back(), description: 'Goes back to the previous page', }, { command: 'go forward', callback: () => window.history.forward(), description: 'Goes to the next page', }, ]; const { transcript } = useSpeechRecognition({ commands }); return ( <div> <button onClick={SpeechRecognition.startListening}>Start</button> <button onClick={SpeechRecognition.stopListening}>Stop</button> <p>{transcript}</p> </div> ); }; export default CommandControlledComponent;
In the `CommandControlledComponent` component, we’ve defined an array of commands. Each command object contains the `command` property, which is the spoken phrase to match, and the `callback` property, which is the function to execute when the phrase is recognized.
Handling Unsupported Browsers
It’s crucial to consider that not all browsers support the SpeechRecognition API. Fortunately, the React Speech Recognition library provides a `browserSupportsSpeechRecognition` function. It returns a boolean indicating whether the user’s browser supports speech recognition.
if (!SpeechRecognition.browserSupportsSpeechRecognition()) { return ( <p> Sorry, your browser does not support in-built speech recognition. Consider using the latest version of Google Chrome. </p> ); }
This way, developers can provide a fallback or informative message for users with unsupported browsers.
Conclusion
The useSpeechRecognition hook from the React Speech Recognition library opens the door to a more interactive and accessible user experience. By effectively harnessing this tool, developers can build voice-controlled applications, improving accessibility and fostering greater user engagement.
The above examples just scratch the surface of what you can achieve with useSpeechRecognition. Remember that although voice-controlled applications add novelty and increase accessibility, they shouldn’t replace traditional user interfaces entirely. Instead, consider them an additional layer of interaction to make your application more user-friendly and accessible to a broader audience.
With useSpeechRecognition, ReactJS developers can readily integrate voice-controlled functionality into their applications, creating more engaging, interactive, and accessible user experiences.
Table of Contents
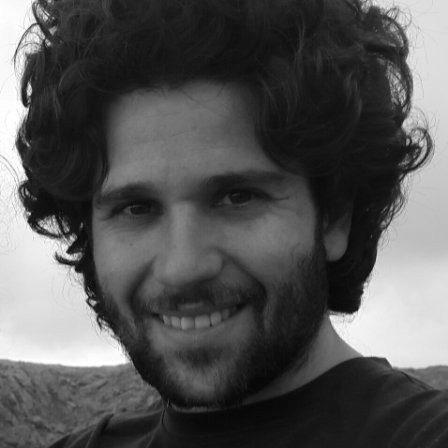
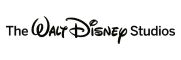