Speaking the User’s Language: Building Dynamic Text-to-Speech Applications with ReactJS’s useSpeechSynthesis Hook
When it comes to enriching user experience in web development, implementing Text-to-Speech (TTS) can be a game-changer. In this blog post, we’ll explore a powerful, yet underused tool in ReactJS called useSpeechSynthesis.
What is useSpeechSynthesis?
First, let’s establish what useSpeechSynthesis is. It’s a custom hook provided by the Web Speech API that converts text into audible speech. In simpler terms, it’s a feature that allows a website or app to “speak” to users. Integrating useSpeechSynthesis into your React application can significantly improve accessibility and offer a more interactive user experience.
Setting Up a New React App
To get started, let’s set up a new React app. If you haven’t done it yet, install Node.js and npm. Next, install `create-react-app` globally on your computer and initiate a new project as follows:
npx create-react-app tts-react-app cd tts-react-app npm start
Now, open your browser and navigate to `http://localhost:3000/` to see your new React application running.
Install useSpeechSynthesis Hook
Next, we’ll need to install a package that provides the useSpeechSynthesis hook. We’ll use `react-speech-kit` for this. Navigate to your project directory and run:
npm install react-speech-kit
Creating a Simple Text-to-Speech Feature
With our new React app set up and the useSpeechSynthesis hook installed, we can start to build a basic TTS feature.
Let’s create a new file `SpeechSynthesis.js` and add the following code:
import React, { useState } from 'react'; import { useSpeechSynthesis } from 'react-speech-kit'; function SpeechSynthesis() { const [text, setText] = useState(''); const { speak } = useSpeechSynthesis(); return ( <div> <input type="text" value={text} onChange={(event) => setText(event.target.value)} /> <button onClick={() => speak({ text: text })}>Speak</button> </div> ); } export default SpeechSynthesis;
Here, we’ve created an input field and a button. The text entered into the input field will be spoken aloud when the ‘Speak’ button is clicked.
Expanding the TTS Feature
Our simple TTS feature works fine, but it’s a bit basic. Let’s improve it by adding an option to choose from different voices.
Update the `SpeechSynthesis.js` file with the following code:
import React, { useState } from 'react'; import { useSpeechSynthesis } from 'react-speech-kit'; function SpeechSynthesis() { const [text, setText] = useState(''); const { speak, voices } = useSpeechSynthesis(); const [voiceIndex, setVoiceIndex] = useState(0); return ( <div> <input type="text" value={text} onChange={(event) => setText(event.target.value)} /> <select onChange={(event) => setVoiceIndex(event.target.value)}> {voices.map((voice, index) => ( <option key={voice.voiceURI} value={index}> {`${voice.lang} - ${voice.name}`} </option> ))} </select> <button onClick={() => speak({ text: text, voice: voices[voiceIndex] })}>Speak</button> </div> ); } export default SpeechSynthesis;
In this code, we’ve introduced the `voices` object which contains different voice options. We’ve also added a dropdown menu to allow users to choose from these different voices. The selected voice is then passed as a parameter to the `speak` function.
Error Handling and Control
Our application can be further improved by introducing error handling and some control features like ‘pause’, ‘resume’, and ‘cancel’. Update your `SpeechSynthesis.js` file as follows:
import React, { useState } from 'react'; import { useSpeechSynthesis } from 'react-speech-kit'; function SpeechSynthesis() { const [text, setText] = useState(''); const { speak, voices, speaking, cancel, pause, resume } = useSpeechSynthesis(); const [voiceIndex, setVoiceIndex] = useState(0); if (!voices.length) { return null; } return ( <div> <input type="text" value={text} onChange={(event) => setText(event.target.value)} /> <select onChange={(event) => setVoiceIndex(event.target.value)}> {voices.map((voice, index) => ( <option key={voice.voiceURI} value={index}> {`${voice.lang} - ${voice.name}`} </option> ))} </select> <button disabled={speaking} onClick={() => speak({ text: text, voice: voices[voiceIndex] })}>Speak</button> {speaking && <button onClick={pause}>Pause</button>} {speaking && <button onClick={resume}>Resume</button>} {speaking && <button onClick={cancel}>Stop</button>} </div> ); } export default SpeechSynthesis;
Here, we added three new buttons: ‘Pause’, ‘Resume’, and ‘Stop’. They provide control over the speech output, giving users the ability to pause, resume, or stop the speech as needed. We’ve also added a `disabled` attribute to the ‘Speak’ button to prevent overlapping speeches.
Conclusion
Creating a Text-to-Speech feature using useSpeechSynthesis in ReactJS isn’t as daunting as it may initially appear. The ability to transform text into speech can significantly enhance the user experience of your application, opening up possibilities for a wider audience, including visually impaired users.
In this blog post, we learned how to install and use the useSpeechSynthesis hook from the `react-speech-kit` library to create a basic TTS feature. We then added options to change voices and control buttons to pause, resume, or stop the speech output, providing a higher level of user interactivity.
However, our example is just the tip of the iceberg. There are countless ways to expand this feature according to your application’s specific needs. With useSpeechSynthesis and ReactJS, your creativity is the only limit.
So go ahead, integrate useSpeechSynthesis in your applications and create more immersive and interactive user experiences.
Happy coding!
Table of Contents
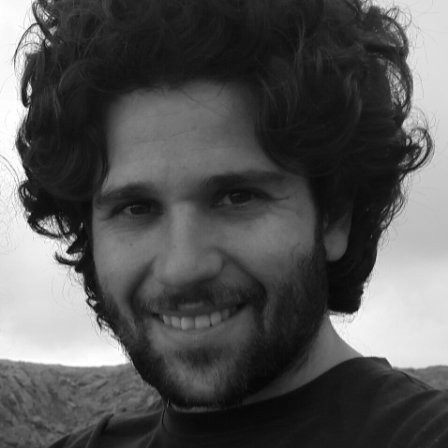
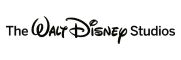