ReactJS and the Web Audio API: Building Music Applications
In recent years, web technologies have rapidly evolved, allowing developers to create complex and engaging multimedia experiences directly in the browser. One such technology is the Web Audio API, which provides a powerful and flexible system for controlling audio. When combined with ReactJS, a popular JavaScript library for building user interfaces, developers can create sophisticated music applications. This blog will explore how to integrate the Web Audio API with ReactJS, discuss key concepts, and provide practical examples.
Understanding the Web Audio API
The Web Audio API is a high-level JavaScript API that enables developers to manipulate audio in web applications. It supports various audio operations like creating sound effects, mixing, and processing audio streams in real-time.
Key Components
- AudioContext: The starting point for using the Web Audio API. It represents the environment in which audio is played and provides methods for creating and controlling audio nodes.
- AudioNode: The building blocks of the audio graph. Each node represents an audio source, effect, or destination.
- AudioBuffer: Represents a short audio file that can be played or manipulated.
Setting Up a ReactJS Project
Before diving into the Web Audio API, we need a basic ReactJS setup. If you don’t already have a React project, you can create one using Create React App:
```bash npx create-react-app react-audio-app cd react-audio-app npm start ```
This command creates a new React project and starts the development server.
Integrating the Web Audio API with ReactJS
Now, let’s integrate the Web Audio API into our React application. We’ll start by creating a simple component that plays a sound when a button is clicked.
Creating an AudioContext
First, we need to create an AudioContext. This can be done in the component’s useEffect hook to ensure it is initialized once the component is mounted.
j ```javascript import React, { useEffect, useRef } from 'react'; const AudioPlayer = () => { const audioContextRef = useRef(null); useEffect(() => { audioContextRef.current = new (window.AudioContext || window.webkitAudioContext)(); }, []); return <div>Audio Player</div>; }; export default AudioPlayer; ```
Loading and Playing Audio
Next, we’ll load an audio file and play it using the AudioContext. We’ll use the fetch API to load the audio file and the decodeAudioData method to decode it into an AudioBuffer.
```javascript const loadAndPlayAudio = async () => { const response = await fetch('path/to/audio/file.mp3'); const arrayBuffer = await response.arrayBuffer(); const audioBuffer = await audioContextRef.current.decodeAudioData(arrayBuffer); playAudio(audioBuffer); }; const playAudio = (audioBuffer) => { const source = audioContextRef.current.createBufferSource(); source.buffer = audioBuffer; source.connect(audioContextRef.current.destination); source.start(); }; ```
Adding a Play Button
To complete our simple audio player, we’ll add a button that calls loadAndPlayAudio when clicked.
```javascript return ( <div> <button onClick={loadAndPlayAudio}>Play Sound</button> </div> ); ```
Advanced Features
Creating an Audio Visualizer
To enhance the user experience, you can create an audio visualizer that displays a graphical representation of the audio being played. This can be achieved by connecting an AnalyserNode to the audio graph and using the data to draw on a canvas.
```javascript const createVisualizer = () => { const analyser = audioContextRef.current.createAnalyser(); source.connect(analyser); analyser.connect(audioContextRef.current.destination); // Use analyser to get audio data and draw on canvas }; ```
Implementing Audio Effects
The Web Audio API also allows you to apply effects like gain (volume control), panning, and filters. For example, you can create a gain node to control the volume of the audio.
```javascript const gainNode = audioContextRef.current.createGain(); source.connect(gainNode); gainNode.connect(audioContextRef.current.destination); gainNode.gain.value = 0.5; // Set volume to 50% ```
Conclusion
Integrating the Web Audio API with ReactJS provides a robust platform for building interactive and dynamic music applications. With the ability to control and manipulate audio in real-time, developers can create unique user experiences. Whether you’re building a simple audio player or a complex music production tool, the combination of ReactJS and the Web Audio API offers endless possibilities.
Further Reading
Table of Contents
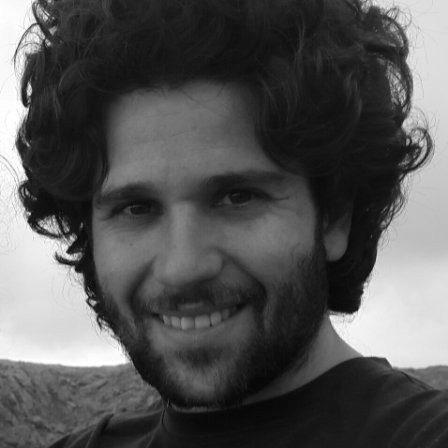
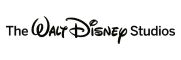