ReactJS and Web Workers: Boosting Performance with Multithreading
When building modern web applications, performance is a crucial factor that can significantly impact user experience. One of the primary challenges in web development is ensuring that the user interface (UI) remains responsive, especially when executing heavy computations or handling numerous tasks. ReactJS, a popular library for building UIs, offers several ways to manage state and lifecycle efficiently. However, when it comes to handling CPU-intensive operations, React alone may not be sufficient to maintain smooth performance. This is where Web Workers come into play, providing a solution for multithreading in JavaScript.
What are Web Workers?
Web Workers are a browser feature that allows you to run JavaScript in the background, independently of the main execution thread. This means that long-running scripts can be executed without blocking the UI, leading to a more responsive application. Web Workers can be especially beneficial for tasks such as data processing, large computations, and complex calculations that would otherwise slow down the main thread.
How Do Web Workers Work?
Web Workers run in an isolated thread, separate from the main JavaScript thread. They do not have direct access to the DOM or other JavaScript objects of the main thread. Communication between the main thread and the worker is done using messages, typically through the `postMessage` and `onmessage` methods.
Here’s a simple example to illustrate how Web Workers can be used in a React application:
Main Thread (React Component)
```javascript import React, { useEffect, useState } from 'react'; const WorkerExample = () => { const [result, setResult] = useState(null); useEffect(() => { if (window.Worker) { const worker = new Worker('./worker.js'); worker.postMessage('start computation'); worker.onmessage = (e) => { setResult(e.data); worker.terminate(); }; } else { console.error('Web Workers are not supported in your browser.'); } }, []); return ( <div> <h2>Web Worker Example</h2> <p>Result: {result}</p> </div> ); }; export default WorkerExample; ```
Worker Thread (worker.js)
```javascript self.onmessage = (e) => { if (e.data === 'start computation') { // Simulate a heavy computation let sum = 0; for (let i = 0; i < 1e9; i++) { sum += i; } self.postMessage(sum); } }; ```
In this example, a simple React component sends a message to a Web Worker to start a computation. The worker performs the calculation without blocking the UI, and once the computation is done, it sends the result back to the main thread.
Benefits of Using Web Workers in ReactJS
1. Enhanced Performance
By offloading heavy computations to a Web Worker, the main thread remains free to handle user interactions and UI updates. This separation of concerns ensures that the application remains responsive, even during intensive processing tasks.
2. Improved User Experience
A responsive UI significantly improves the user experience, as it prevents the application from freezing or becoming sluggish. Users can continue interacting with the app while background tasks are processed.
3. Scalability
Web Workers make it easier to scale applications by allowing parallel execution of tasks. This is particularly useful for applications that need to handle large amounts of data or complex operations.
Challenges and Considerations
While Web Workers offer numerous benefits, there are also some challenges to consider:
1. Limited Scope
Web Workers cannot access the DOM directly. This means that any DOM manipulation must be done in the main thread. Developers need to carefully design the communication between the worker and the main thread to ensure that the application remains efficient.
2. Browser Compatibility
Although Web Workers are widely supported, there may still be some limitations or inconsistencies across different browsers. It’s essential to test the application across various environments to ensure compatibility.
3. Complexity in Debugging
Debugging Web Workers can be more challenging than regular JavaScript code, as they run in a separate thread. Developers need to use specific tools and techniques to diagnose and fix issues.
Conclusion
Web Workers are a powerful tool for enhancing the performance of ReactJS applications. By offloading heavy computations to a separate thread, developers can ensure that their applications remain responsive and provide a smooth user experience. However, it’s crucial to consider the limitations and complexities of using Web Workers and to design applications accordingly.
Further Reading
Table of Contents
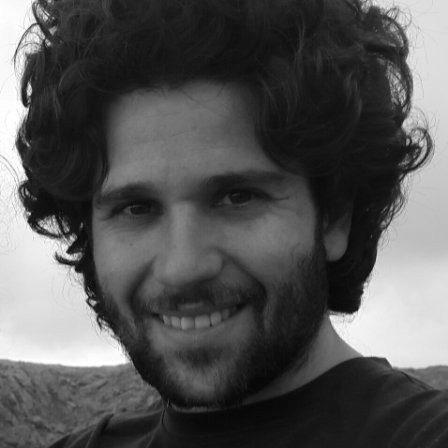
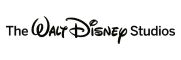