Ruby on Rails Tutorial: Understanding ActionMailer and Email Deliverability
Email is a fundamental part of modern web applications, used for everything from user notifications to password resets. In Ruby on Rails, ActionMailer is the framework’s tool for managing email functionality. However, sending an email is just the beginning; ensuring that those emails are successfully delivered to users’ inboxes is equally important. This blog will guide you through using ActionMailer and provide tips on improving email deliverability.
Getting Started with ActionMailer
ActionMailer is a powerful Rails component that allows you to send emails from your application. It provides a simple way to define email templates and handle email sending with various configurations.
Setting Up ActionMailer
First, ensure that ActionMailer is properly set up in your Rails application. By default, Rails includes ActionMailer, but you need to configure it to connect with an email service provider.
In your `config/environments/development.rb` (and similarly for other environments), configure ActionMailer with your email service settings:
```ruby config/environments/development.rb Rails.application.configure do Other configurations... config.action_mailer.delivery_method = :smtp config.action_mailer.smtp_settings = { address: 'smtp.example.com', port: 587, domain: 'example.com', user_name: 'your_username', password: 'your_password', authentication: 'plain', enable_starttls_auto: true } end ```
Replace the placeholders with your actual SMTP server details. This setup will enable ActionMailer to use SMTP for sending emails.
Creating Mailers in Rails
A mailer in Rails is a class that inherits from `ActionMailer::Base`. It defines methods that correspond to different types of emails your application will send.
Generate a new mailer using Rails’ generator:
```bash rails generate mailer UserMailer ```
This command creates a mailer class and view templates. Open the generated mailer class `app/mailers/user_mailer.rb` and define a method for sending a welcome email:
```ruby app/mailers/user_mailer.rb class UserMailer < ApplicationMailer default from: 'notifications@example.com' def welcome_email(user) @user = user @url = 'http://example.com/login' mail(to: @user.email, subject: 'Welcome to My Awesome Site') end end ```
Then, create a corresponding view template for the email in `app/views/user_mailer/welcome_email.html.erb`:
```erb <!-- app/views/user_mailer/welcome_email.html.erb --> <h1>Welcome to My Awesome Site, <%= @user.name %>!</h1> <p> You have successfully signed up to our amazing app. Your login URL is <%= link_to 'Login', @url %>. </p> ```
Sending the Email
To send the email, you can call the `welcome_email` method from a controller or background job:
```ruby UserMailer.welcome_email(@user).deliver_now ```
Ensuring Email Deliverability
While sending emails is straightforward, ensuring that they actually reach your users’ inboxes requires attention to several factors.
- Use a Reputable SMTP Provider
Choose a well-known SMTP provider like SendGrid, Mailgun, or Amazon SES. These providers have built-in features to handle deliverability issues and manage your email reputation.
- Implement SPF, DKIM, and DMARC
– SPF (Sender Policy Framework): Helps prevent email spoofing by specifying which mail servers are allowed to send emails on behalf of your domain.
– DKIM (DomainKeys Identified Mail): Adds a digital signature to your emails, allowing receiving servers to verify that the email hasn’t been altered.
– DMARC (Domain-based Message Authentication, Reporting & Conformance): Provides instructions to email servers on how to handle emails that fail SPF or DKIM checks.
You can set these up in your domain’s DNS settings.
- Monitor Email Engagement
Track metrics such as open rates, click-through rates, and bounce rates. High bounce rates or low engagement can impact your sender reputation. Use tools like Mailgun’s analytics or SendGrid’s dashboard to monitor these metrics.
Conclusion
ActionMailer in Ruby on Rails makes it easy to integrate email functionality into your application. By setting up mailers and ensuring proper email deliverability, you can enhance communication with your users and maintain a positive user experience. Following best practices for email deliverability will help ensure that your emails reach their intended recipients and avoid common pitfalls.
Further Reading
- [Rails Guides: Action Mailer Basics](https://guides.rubyonrails.org/action_mailer_basics.html)
- [Understanding SPF, DKIM, and DMARC](https://sendgrid.com/blog/what-is-spf-dkim-dmarc/)
- [Improving Email Deliverability](https://www.mailgun.com/blog/what-is-email-deliverability-and-how-to-improve-it/)
By applying these techniques, you can effectively manage email communications in your Rails application and improve the overall deliverability of your messages. Happy coding!
Table of Contents
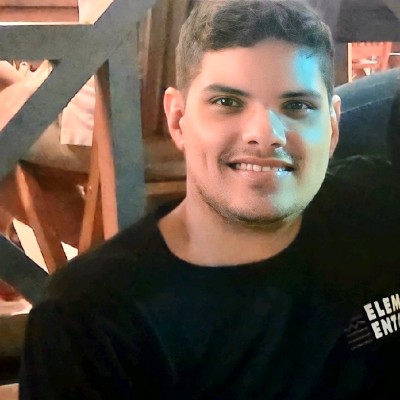
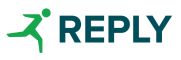